Assume classes LinkedQueue and Array5tack have been defined using the implementations in you textbook that myQQueue and myStack have been initialized so they are empty. Type the EXACT output of the following code segment. You may assume that the code compiles and executes without errors.
Assume classes LinkedQueue and Array5tack have been defined using the implementations in you textbook that myQQueue and myStack have been initialized so they are empty. Type the EXACT output of the following code segment. You may assume that the code compiles and executes without errors.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![Assume classes LinkedQueue and Array5tack have been defined using the implementations in your
textbook that myQueue and myStack have been initialized so they are empty. Type
the EXACT output of the following code segment. You may assume that the code compiles and
executes without errors.
Array5tack<int> my5tack;
LinkedQueue<int> myQueue;
int i- 1;
int j = 2;
int k = 3;
int n = 4;
myStack.push ();
myQueue.enqueue ();
myStack.push (k);
myQueue.enqueue (k);
n = my5tack.peek ();
my5tack.pop );
n = myQueue.peekFront ();
myQueue.dequeue ();
myStack.push (i);
myQueue.enqueue ();
myStack.push (n);
myQueue.enqueue (n);
my5tack.push (k);
myQueue.enqueue (k);
while (Imy5tack.isEmpty ()
{
i = my5tack.peek (0:
my5tack.pop ();
cout << i<< " ";
while (ImyQueue.isEmpty ())
{
j = myQueue.peekFront ();
myQueue.dequeue ();
cout << j<< "";
cout << endl;
[11]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1befada9-81e2-4d46-becb-ee0edf9c2902%2F4e6ff247-34d4-4c22-871f-270810a2a101%2Fpxrp8xv_processed.png&w=3840&q=75)
Transcribed Image Text:Assume classes LinkedQueue and Array5tack have been defined using the implementations in your
textbook that myQueue and myStack have been initialized so they are empty. Type
the EXACT output of the following code segment. You may assume that the code compiles and
executes without errors.
Array5tack<int> my5tack;
LinkedQueue<int> myQueue;
int i- 1;
int j = 2;
int k = 3;
int n = 4;
myStack.push ();
myQueue.enqueue ();
myStack.push (k);
myQueue.enqueue (k);
n = my5tack.peek ();
my5tack.pop );
n = myQueue.peekFront ();
myQueue.dequeue ();
myStack.push (i);
myQueue.enqueue ();
myStack.push (n);
myQueue.enqueue (n);
my5tack.push (k);
myQueue.enqueue (k);
while (Imy5tack.isEmpty ()
{
i = my5tack.peek (0:
my5tack.pop ();
cout << i<< " ";
while (ImyQueue.isEmpty ())
{
j = myQueue.peekFront ();
myQueue.dequeue ();
cout << j<< "";
cout << endl;
[11]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
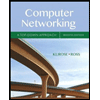
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
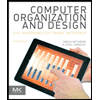
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
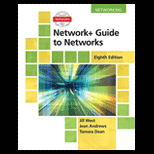
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
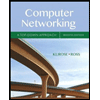
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
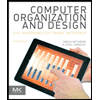
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
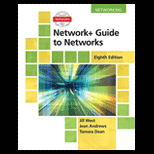
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
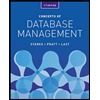
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
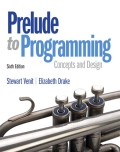
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
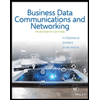
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY