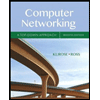
Assume that following initialization is given in your structure allStudent[5] and data is sorted based on name field,
struct studInfo
{ int stNr;
char name[10] ;
int grade;
};
int main() // already sorted base on name field
{
struct studInfo allStudent[5]={ 97790, “ADEM” , 77,
963582, “AFGAR” , 85,
957434 ,”AHMED”, 100,
921229 ,”BAKI” , 65,
971280 ,”ZEKI” , 88};
int n=5;/* Total number of students*/
char givenName[10];
gets(givenName);
k=binarySearch(………..);
…
….
sortBubble(……);
..
..
..
return 0;
}
- A) Write a C function named as binarySearch() and search a given student(get a name information from the monitor) in the given array allStudent[] , apply Binary Search , and list student information(stNr , name , grade).If student in not found give an error message in the main() program that “Student is not found”.
B)Write a C function named as sortBubble() that will sort the student information by Student Number(stNr) applying by Bubble Sort method, and after sort operation list sorted student information in the main program.

Step by stepSolved in 3 steps with 6 images

- Declare a student structure that contains : Student's first and last nameStudent IDCreate the following functions: getStudentInfo(void) Declares a single student, uses printf()/scanf() to get inputReturns the single student backprintStudentInfo(student_t *st_ptr) Takes the pointer to a student (to avoid making a copy)Prints out all of the student informationDeclare an array of five studentsUsing a for loop and getStudentInfo() function, get input of all the studentsUsing a for loop and printStudentInfo() function, print all the output of all students.arrow_forwardtypedef _people { int age; char name[ 32 ] ; } People_T ; People_T data [ 3 ]; Using string lib function, Assign 30 and Cathy to the first cell, Assign 40 and John to the second cell and Assign 50 and Tom to the third cell People_T *ptr ; Declare a pointer pointing to the structure data and print the age and name using the pointer. your output can be : 30 Cathy 40 John 50 Tomarrow_forwardA data structure in which all elements have the same type is called an ______arrow_forward
- Programarrow_forwardMy code from milestone 1 # Define the quiz_type dictionary quiz_type = { 1: "BabyAnimals", 2: "Brooklyn99", 3: "Disney", 4: "Hogwarts", 5: "MyersBriggs", 6: "SesameStreet", 7: "StarWars", 8: "Vegetables" } # Print the welcome message print("Welcome to the Personality Quiz!") print() print("What type of Personality Quiz do you want to run?") print() for number, quiz_name in quiz_type.items(): print(f"{number} - {quiz_name}") print() test_number = int(input("Choose test number (1-8): ")) # Check if the test_number is valid if test_number in quiz_type: quiz_name = quiz_type[test_number] print() print(f"Great! Let's begin the {quiz_name} Personality Quiz...") else: print("Invalid test number. Please choose a number between 1 and 8.") ------------------------------------------------------------------- Milestone #3 code : # Ending statements with the period to easily split them as mentioned in the question. questions = [ "I…arrow_forwardC# helparrow_forward
- struct Info { int id; float cost; }; Given the struct above, the following code segment will correctly declare and initialize an instance of that struct. Info myInfo = {10,4.99}; Group of answer choices True False ============== Consider the following code fragment. int a[] = {3, -5, 7, 12, 9}; int *ptr = a; *ptr = (*ptr) + 1; cout << *ptr; Group of answer choices 4 -5 3 Address of -5 =============== What will happen if I use new without delete? Group of answer choices The program will never compile The program will never run The program will run fine without memory leak None of thesearrow_forwardGiven the following declaration : char msg[100] = "Department of Computer Science"; What is printed by: strcpy_s(msg, "University"); int len = strlen(msg); for (int i = len-3; i >0; i--) { msg[i] = 'x'; } cout << msg;arrow_forwardC++ Write a program that will allow a user to enter students into a database. The student information should include full name, student ID, GPA and status. The program should allow delete any student based on student ID. You may not use a vector.arrow_forward
- Game of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forwardtypedef char string[50]; struct a Tag { int a; }; // 1. indicate the data type returned by function Exam() Exam(struct a Tag *ptr) { float b; double C[ 10 ]; string s; } // Access the members of the structure variable indirectly via ptr // You are REQUIRED to use the structure pointer operator; see comments numbered 2 to 5. // 2. input the value of member a scanf("%d", ); // 3. assign 3.1416 as the value of member b = 3.1416; // 4. print the value of the last element of array C. printf("%lf\n", |); // 5. copy "Hello" into string s strcpy( return ptr; , "Hello");arrow_forward#include using namespace std; struct ListNode { string data; ListNode *next; }; int main() { ListNode *p, *list; list = new ListNode; list->data = "New York"; p new ListNode; p->data = "Boston"; list->next = p; p->next = new ListNode; p->next->data = "Houston"; p->next->next = nullptr; // new code goes here Which of the following code correctly deletes the node with value "Boston" from the list when added at point of insertion indicated above? O list->next = p; delete p; O p = list->next; %3D list->next = p->next; delete p; p = list->next; list = p->next; delete p; O None of these O p = list->next; %3D list->next = p; %3D delete p;arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
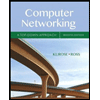
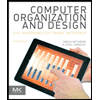
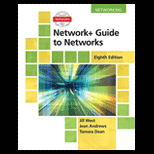
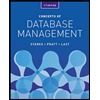
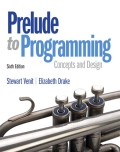
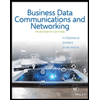