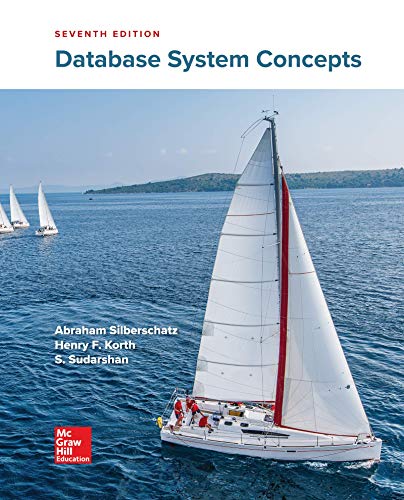
C++ 13.9.2 Pass by reference
Define a function ScaleGrade() that takes two parameters:
- points: an integer, passed by value, for the student's score.
- grade: a char, passed by reference, for the student's letter grade.
ScaleGrade() changes grade to A if the points are greater than or equal to 88 and less than or equal to 100, and grade is not A. Otherwise, grade is not changed. The function returns true if grade has changed, and returns false otherwise.
Ex: If the input is 88 B, then the output is:
Grade is A after curving.
Using the following code
#include <iostream>
using namespace std;
// insert code here
int main() {
int studentPoints;
char studentGrade;
bool isChanged;
cin >> studentPoints;
cin >> studentGrade;
isChanged = ScaleGrade(studentPoints, studentGrade);
if (isChanged) {
cout << "Grade is " << studentGrade << " after curving." << endl;
}
else {
cout << "Grade " << studentGrade << " is not changed." << endl;
}
return 0;
}
* I was trying to use a code block like this:
bool ScaleGrade(int& studentPoints, char& studentGrade){
if(studentPoints>=88 || studentPoints<=100){
studentGrade='A';
return false;
}
else{
return false;
}
}
However I only can get half the checks to actually pass.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Add a function to get the CPI values from the user and validate that they are greater than 0. 1. Declare and implement a void function called getCPIValues that takes two float reference parameters for the old_cpi and new_cpi. 2. Move the code that reads in the old_cpi and new_cpi into this function. 3. Add a do-while loop that validates the input, making sure that the old_cpi and new_cpi are valid values. + if there is an input error, print "Error: CPI values must be greater than 0." and try to get data again. 4. Replace the code that was moved with a call to this new function. - Add an array to accumulate the computed inflation rates 1. Declare a constant called MAX_RATES and set it to 20. 2. Declare an array of double values having size MAX_RATES that will be used to accumulate the computed inflation rates. 3. Add code to main that inserts the computed inflation rate into the next position in the array. 4. Be careful to make sure the program does not overflow the array. - Add a…arrow_forwardC++ Write a void function that has two parameters: a value parameter called num that receives a floating point number from the calling function and a floating point reference parameter called result. The functions should do this calculation: 25.0 * num + 37 and store the answer in result. Do not do any read or write operations in the function.arrow_forwardNested function classwork 2 Write 'taxIncome', a user-defined function that calculates income tax. y = taxincome (income) defines the principal function. The main function calculates adjusted income as income - 6000. Then y = compute Tax is called. For y = 0.28*Adjusted Income, this function uses 'Adjusted Income'. 'Adjusted Income' was defined in the main function. Use $80,000 income.arrow_forward
- Write in C++ 1. Define a struct for a soccer player that stores their name, jersey number, and total points scored. 2.Using the struct in #1, write a function that takes an array of soccer players and its size as arguments and returns the average number of points scored by the players. 3.Using the struct in #1, write a function that takes an array of soccer players and its size as arguments and returns the index of the player who scored the most points. 4.Using the struct in #1, write a function that sorts an array of soccer players by name. 5.Using the struct in #1, write a function that takes an (unsorted) array of soccer players and its size and a number as arguments, and returns the name of the soccer player with that number. It should not do any extra unnecessary work. 6.Define a function to find a given target value in an array, but use pointer notation rather than array notation whenever possible. 7.Write a swap function, that swaps the values of two variables in main, but use…arrow_forwarddef driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon) Your program should call the function three times to determine the gas cost for 10 miles, 50 miles, and 400 miles. 344614.2214230.qx3zqy7 LAB 6.20.1: LAB: Driving costs - functions 0/ 10 ACTIVITY main.py Load default template... 1 # Define your function here. 2 3 if # Type your code here. name main == 4 6arrow_forwardProgramming technique exercise Question 1). Write a complete C++ program that helps the teacher to calculate the result of students in the test of Programming Technique. The program should perform the following tasks: • Write a function named getinput. This is a non-returning function. It takes the score of question 1, score of question 2, and score of question 3 as input parameters. the function should ask the user to enter the score (per 100) for each question. It sends all the values entered by the user in (c) back to the calling module through the use of reference parameters. • Write a function named dispTier. This is a non-returning function. It takes the total marks as an input parameter. The function should display the tier based on the conditions. Tier 3 = below 40%, tier 2 = below75%, tier 1=75% and above • Write a function named calcAverage. I It takes the number of students and total marks as input parameters.arrow_forward
- rografmiming CSC 101 Introduction VB Bau Section21436.02. Sntinn 2022 ta46581 OPRIV NEXTO Workbench Exercise 40443- deadne 04020022 1150 PM WORK AREA toThePowerOf is a function thet accepts two int parameters and returns the value of the first parameter raised to the power of the second. An int variable cubeside has aiready been deciared and initialized. Another int vaciable, cubevolume, has already been declared. Write a statement that calls toThePowerof to compute the value of cubeside raised to the power of 3, and store this value in cubevolume. SUBMIT 1 of 1: Sat Apr 02 2022 23:03:03 GMT-0400 (Eastern Dayilght Time) Type your selution here.arrow_forwardConsider the function definition: void GetNums(int howMany, float& alpha, float& beta) { int i; beta = 0; for (i = 0; i < howMany; i++) { beta = alpha + beta; } } Describe what happens in MEMORY when the GetNums function is called.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
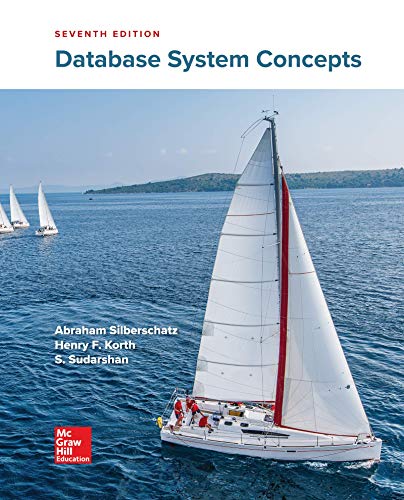
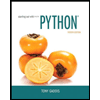
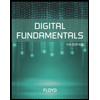
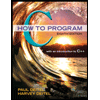
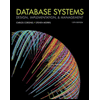
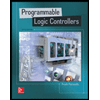