C++ (C plusplus) Requirements: Use the given class “User” (below) All data members must be declared as “private” No multiple return statements within a function or method No global variable is allowed to be declared and used (constants are ok) Methods within the class and the requested functions cannot have “cin” or “cout” but they should make use of parameters and return value instead unless it is explicitly stated. “cin” and “cout” should be used in main() and testing functions You are required to provide main() and testing functions if any that contains testing code to show how you have tested your classes, methods, and functions. You must show at least one call for each method and function and print out its return values and results correctly. Define a new exception class named “BadValueException” that must inherit from the exception class. It will manage and capture the general invalid integer and double value. It must be able to describe the reason for the error and save away the erroneous value. In another word, when the caller catches the exception and receives this object, it must know what the reason for the exception is and the actual bad value that caused the exception. Define a new class named “Student” that must inherit from the given User class. This class manages student info: id (integer), name (string), and gpa (double). It must prevent the creation of a Student object with a negative ID or a GPA that is not between the range of 0.0 and 4.0 by generating a BadValueException error. This class will provide at least the following methods: toString method to return a string representation of the Student object in the format of “ID( ) NAME( ) GPA( )” Such as: ID(1234) NAME(John Smith) GPA(4.00) Note: the GPA must have 2 decimal places. isGPAGreater method that compares its gpa with another student’s gpa. It returns true if its gpa is greater than the other and false otherwise. Define another new class named “GradStudent” that must inherit from the Student class. This GradStudent class will manage the following info: id, name, gpa, and graduation year (integer). This class should allow the creation of a GradStudent object with or without a given graduation year. If the year is not given, it will default to 2022. Its toString() method should return a string with the graduation year info such as: ID(3000) NAME(Nancy Brown) GPA(3.50) GRADUATION-YEAR(2022) Write a function named “getLowest” that accepts a vector of pointers to Student objects. It will go through the list and return pointers to the Student or GradStudent with the lowest student ID and GPA. Please note that the vector contains pointers to either Student or GradStudent objects. Please show that you have tested with a vector of pointers of mixed Student and GradStudent objects (in the same vector). For example, if these students and grad students are in the list: Student(2000, "John Smith", 4.0) GradStudent(3000,"Nancy Brown", 3.5, 2021) ; Student(1000, "Bob Johnson", 3.0) ; GradStudent(4000, "Tim Jackson", 2.0, 2020)) ; It will return pointers to these two objects: Lowest ID: ID(1000) NAME(Bob Johnson) GPA(3.00) Lowest GPA: ID(4000) NAME(Tim Jackson) GPA(2.00) GRADUATIONYEAR(2020) Write a function named “createOneStudent” that will read from the user the student info: id, name, and gpa. It will use try-catch to handle the exception if the user provides a negative id or gpa that is out of bound (< 0.0 or > 4.0) by asking the user to re-enter them. It must provide the correct reason for the error and the actual error value. Then it will allow the user to try up to 3 times. This function will return the pointer of a newly created Student object or nullptr if the info is still incorrect. Note that this function can and will use cin and cout to read in values from the user. ///////////////////////////////////////// Given class “User”: class User { private: int m_id ; string m_name ; public: User(int id, string name): m_id(id), m_name(name) { } virtual string toString() { stringstream ss; ss << "ID(" << m_id << ") NAME("<< m_name << ")" ; return ss.str() ; } bool isIDLarger(User & another) { return m_id > another.m_id ; } }; Note: you cannot modify this given User class. ///////////////////////////////////////// Expected result example (attached as a screenshot).
C++ (C plusplus)
Requirements:
- Use the given class “User” (below)
- All data members must be declared as “private”
- No multiple return statements within a function or method
- No global variable is allowed to be declared and used (constants are ok)
- Methods within the class and the requested functions cannot have “cin” or “cout” but they should make use of parameters and return value instead unless it is explicitly stated.
- “cin” and “cout” should be used in main() and testing functions
- You are required to provide main() and testing functions if any that contains testing code to show how you have tested your classes, methods, and functions. You must show at least one call for each method and function and print out its return values and results correctly.
Define a new exception class named “BadValueException” that must inherit from the exception class. It will manage and capture the general invalid integer and double value. It must be able to describe the reason for the error and save away the erroneous value. In another word, when the caller catches the exception and receives this object, it must know what the reason for the exception is and the actual bad value that caused the exception.
Define a new class named “Student” that must inherit from the given User class. This class manages student info: id (integer), name (string), and gpa (double). It must prevent the creation of a Student object with a negative ID or a GPA that is not between the range of 0.0 and 4.0 by generating a BadValueException error.
This class will provide at least the following methods:
- toString method to return a string representation of the Student object in the format of “ID( <id> ) NAME( <name> ) GPA( <gpa> )” Such as:
ID(1234) NAME(John Smith) GPA(4.00)
Note: the GPA must have 2 decimal places.
- isGPAGreater method that compares its gpa with another student’s gpa. It returns true if its gpa is greater than the other and false otherwise.
Define another new class named “GradStudent” that must inherit from the Student class. This GradStudent class will manage the following info: id, name, gpa, and graduation year (integer). This class should allow the creation of a GradStudent object with or without a given graduation year. If the year is not given, it will default to 2022. Its toString() method should return a string with the graduation year info such as:
ID(3000) NAME(Nancy Brown) GPA(3.50) GRADUATION-YEAR(2022)
Write a function named “getLowest” that accepts a
For example, if these students and grad students are in the list:
Student(2000, "John Smith", 4.0)
GradStudent(3000,"Nancy Brown", 3.5, 2021) ;
Student(1000, "Bob Johnson", 3.0) ;
GradStudent(4000, "Tim Jackson", 2.0, 2020)) ;
It will return pointers to these two objects:
Lowest ID: ID(1000) NAME(Bob Johnson) GPA(3.00)
Lowest GPA: ID(4000) NAME(Tim Jackson) GPA(2.00) GRADUATIONYEAR(2020)
Write a function named “createOneStudent” that will read from the user the student info: id, name, and gpa. It will use try-catch to handle the exception if the user provides a negative id or gpa that is out of bound (< 0.0 or > 4.0) by asking the user to re-enter them. It must provide the correct reason for the error and the actual error value. Then it will allow the user to try up to 3 times. This function will return the pointer of a newly created Student object or nullptr if the info is still incorrect. Note that this function can and will use cin and cout to read in values from the user.
/////////////////////////////////////////
Given class “User”:
class User
{
private:
int m_id ;
string m_name ;
public:
User(int id, string name): m_id(id), m_name(name)
{
}
virtual string toString()
{
stringstream ss;
ss << "ID(" << m_id << ") NAME("<< m_name << ")" ;
return ss.str() ;
}
bool isIDLarger(User & another)
{
return m_id > another.m_id ;
}
};
Note: you cannot modify this given User class.
/////////////////////////////////////////
Expected result example (attached as a screenshot).
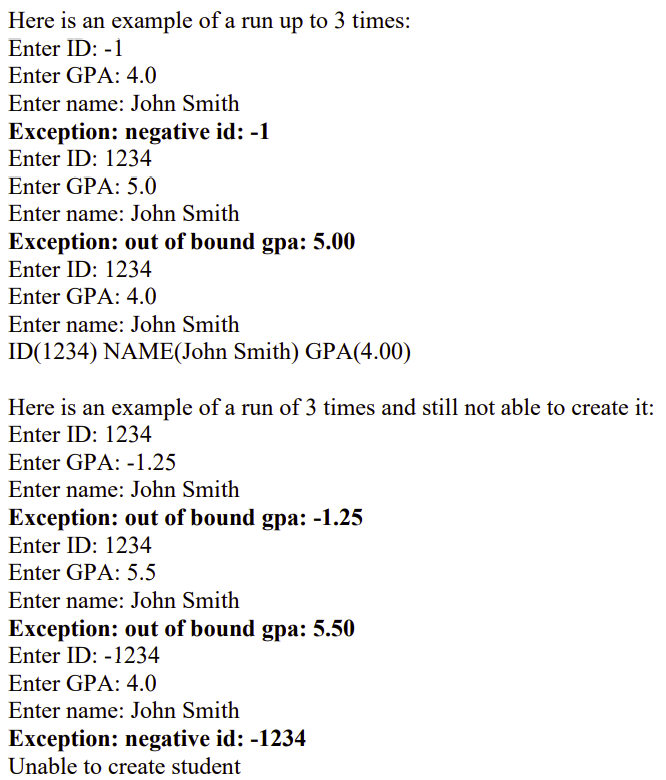

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

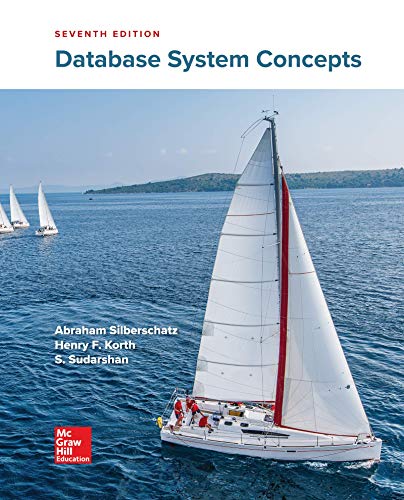
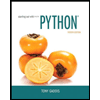
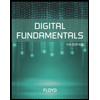
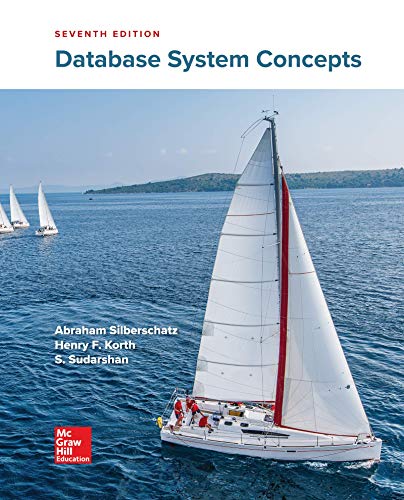
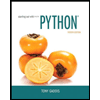
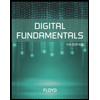
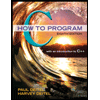
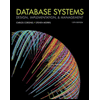
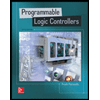