In the example above you required the user to input the values for the array. Modify the program in Part 1 so that instead of asking the user for the values of array elements, they are all initialized using a single statement.
The example :
#include<stdio.h>
int main(){
/* 2D array declaration*/
int disp[2][3];
/*Counter variables for the loop*/
int i, j;
for(i=0; i<2; i++) {
for(j=0;j<3;j++) {
printf("Enter value for disp[%d][%d]:", i, j);
scanf("%d", &disp[i][j]);
}
}
//Displaying array elements
printf("Two Dimensional array elements:\n");
for(i=0; i<2; i++) {
for(j=0;j<3;j++) {
printf("%d ", disp[i][j]);
if(j==2){
printf("\n");
}
}
}
return 0;
}
question :
In the example above you required the user to input the values for the array.
Modify the program in Part 1 so that instead of asking the user for the values of array elements, they are all initialized using a single statement.

Step by step
Solved in 3 steps with 2 images

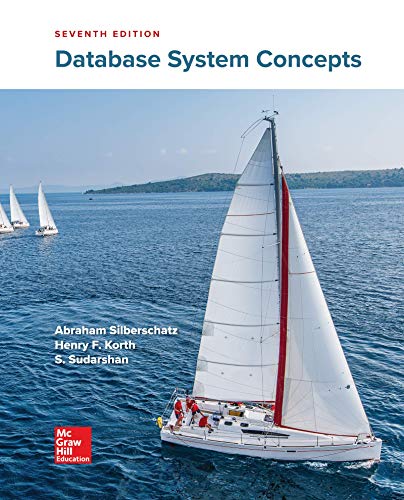
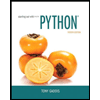
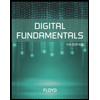
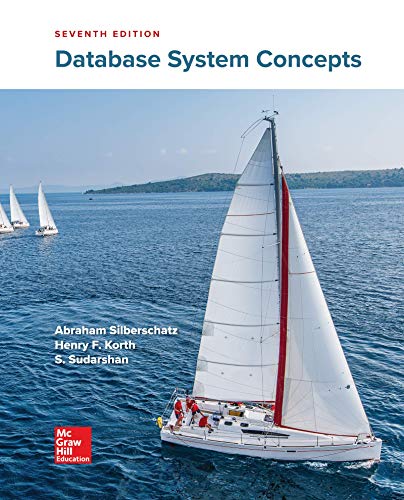
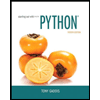
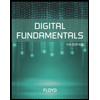
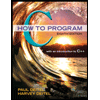
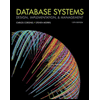
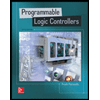