C++ Help converting existing assignment to use classes. Original assignment: Create a program that calculates the hypotenuse of a triangle, the area of a trapezoid, and the volume of a rectangle. The user must input the variables in the formulas. Changes to be made: Convert previous assignment to utilize a class structure in portable .h and .cpp files Exit the program only on user demand code: //dispays menu void displayMenu() { cout << "Please Choose a calculation function" << endl; cout << endl; cout << "Enter 1 to calculate the hypotenuse of a triangle of a triangle" << endl; cout << endl; cout << "Enter 2 to calculate the area of a trapezoid" << endl; cout << endl; cout << "Enter 3 to calculate the volume of a rectangular prism" << endl; cout << endl; cout << "Enter any other letter or number input to exit" << endl; } //hypotenuse of triangle and associated functions double calculateHypotenuse(double sideA, double sideB) { double hypotenuse = sqrt((sideA * sideA) + (sideB * sideB)); return hypotenuse; } void hypotenuseMenuOption() { double side1; double side2; cout << "Enter the measurement of the base of the triangle" << endl; cin >> side1; cout << "Enter the measurement of the height of the triangle" << endl; cin >> side2; cout << endl; cout << " The hypotenuse of the triangle is: " << calculateHypotenuse(side1, side2); } //trapezoid area and associated functions double calculateTrapezoidArea(double base1, double base2, double height) { double area = ((base1 + base2) / 2) * height; return area; } void trapezoidAreaMenuOption() { double b1; double b2; double h; cout << "Enter the first base of the trapezoid" << endl; cin >> b1; cout << "Enter the second base of the trapezoid" << endl; cin >> b2; cout << "Enter the height of the trapezoid" << endl; cin >> h; cout << endl; cout << "The area of the trapezoid is: " << calculateTrapezoidArea(b1, b2, h); } //volume of a rectangular prism and associated functions double calculateRectangleVolume(double length, double width, double height) { double volume = length * width * height; return volume; } void rectangleVolumeMenuOption() { double rectangleLength; double rectangleWidth; double rectangleHeight; cout << "Enter the length of the rectangle" << endl; cin >> rectangleLength; cout << "Enter the width of the rectangle" << endl; cin >> rectangleWidth; cout << "Enter the height of the rectangle" << endl; cin >> rectangleHeight; cout << endl; cout << "The volume of the rectangular prism is: " << calculateRectangleVolume(rectangleLength, rectangleWidth, rectangleHeight); } //driver int main() { displayMenu(); int menuChoice; cin >> menuChoice; if (menuChoice == 1) { hypotenuseMenuOption(); } else if (menuChoice == 2) { trapezoidAreaMenuOption(); } else if (menuChoice == 3) { rectangleVolumeMenuOption(); } else { exit(0); }
C++ Help converting existing assignment to use classes.
Original assignment:
Create a program that calculates the hypotenuse of a triangle, the area of a trapezoid, and the volume of a rectangle. The user must input the variables in the formulas.
Changes to be made:
Convert previous assignment to utilize a class structure in portable .h and .cpp files
Exit the program only on user demand
code:
//dispays menu
void displayMenu() {
cout << "Please Choose a calculation function" << endl;
cout << endl;
cout << "Enter 1 to calculate the hypotenuse of a triangle of a triangle" << endl;
cout << endl;
cout << "Enter 2 to calculate the area of a trapezoid" << endl;
cout << endl;
cout << "Enter 3 to calculate the volume of a rectangular prism" << endl;
cout << endl;
cout << "Enter any other letter or number input to exit" << endl;
}
//hypotenuse of triangle and associated functions
double calculateHypotenuse(double sideA, double sideB) {
double hypotenuse = sqrt((sideA * sideA) + (sideB * sideB));
return hypotenuse;
}
void hypotenuseMenuOption() {
double side1;
double side2;
cout << "Enter the measurement of the base of the triangle" << endl;
cin >> side1;
cout << "Enter the measurement of the height of the triangle" << endl;
cin >> side2;
cout << endl;
cout << " The hypotenuse of the triangle is: " << calculateHypotenuse(side1, side2);
}
//trapezoid area and associated functions
double calculateTrapezoidArea(double base1, double base2, double height) {
double area = ((base1 + base2) / 2) * height;
return area;
}
void trapezoidAreaMenuOption() {
double b1;
double b2;
double h;
cout << "Enter the first base of the trapezoid" << endl;
cin >> b1;
cout << "Enter the second base of the trapezoid" << endl;
cin >> b2;
cout << "Enter the height of the trapezoid" << endl;
cin >> h;
cout << endl;
cout << "The area of the trapezoid is: " << calculateTrapezoidArea(b1, b2, h);
}
//volume of a rectangular prism and associated functions
double calculateRectangleVolume(double length, double width, double height) {
double volume = length * width * height;
return volume;
}
void rectangleVolumeMenuOption() {
double rectangleLength;
double rectangleWidth;
double rectangleHeight;
cout << "Enter the length of the rectangle" << endl;
cin >> rectangleLength;
cout << "Enter the width of the rectangle" << endl;
cin >> rectangleWidth;
cout << "Enter the height of the rectangle" << endl;
cin >> rectangleHeight;
cout << endl;
cout << "The volume of the rectangular prism is: " << calculateRectangleVolume(rectangleLength, rectangleWidth, rectangleHeight);
}
//driver
int main() {
displayMenu();
int menuChoice;
cin >> menuChoice;
if (menuChoice == 1) {
hypotenuseMenuOption();
}
else if (menuChoice == 2) {
trapezoidAreaMenuOption();
}
else if (menuChoice == 3) {
rectangleVolumeMenuOption();
}
else {
exit(0);
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 8 images

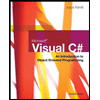
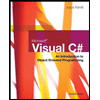