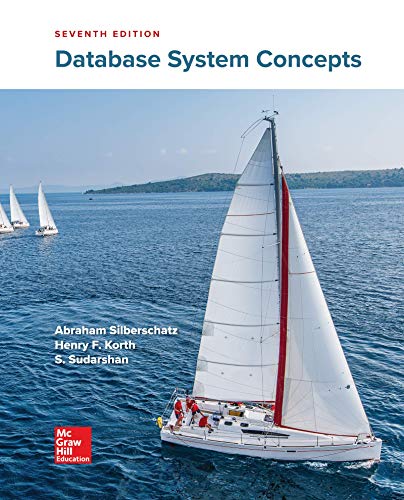
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
C program:
fill out TODO'S IN THE PROGRAM
#include <stdlib.h> // EXIT_ codes
#include <stdbool.h> // bool
#include <stdio.h> // printf, scanf
#include <string.h> // strlen, strcmp
#include <poll.h> // poll
#include <unistd.h> // usleep
#include "sysfs_gpio.h" // custom virtual file system gpio library
#include "udp.h" // custom udp library
#define SERVER_LISTENER_PORT 4096
#define DOOR 17
#define MOTION 27
#define TIMEOUT_SECONDS 25
bool isAnyKeyPressed()
{
struct pollfd pfd;
pfd.fd = 1;
pfd.events = POLLIN;
poll(&pfd, 1, 1);
return pfd.revents == POLLIN;
}
//-----------------------------------------------------------------------------
// Main
//-----------------------------------------------------------------------------
int main(int argc, char* argv[])
{
char *remoteIp;
int remotePort;
bool armed = false;
bool quit = false;
int timeout = TIMEOUT_SECONDS;
// Verify arguments are good
bool goodArguments = (argc == 2);
if (goodArguments)
remoteIp = argv[1];
if (!goodArguments)
{
printf("usage: alarm IPV4_ADDRESS\n");
printf(" where:\n");
printf(" IPV4_ADDRESS is address of the remote machine\n");
exit(EXIT_FAILURE);
}
// Set remote port
remotePort = SERVER_LISTENER_PORT;
// Setup pins as inputs
// TODO: Add code to configure the door button
// and motion sensor pins are inputs
// Send disarmed message
sendUdpMessage(remoteIp, remotePort, "disarmed");
// Loop until 'q' is pressed on keyboard
while (!quit)
{
// TODO: Add code to read the door and motion inputs
// and send messages and display on screen
// If key pressed on keyboard, get key and process
// If 'a', arm system
// If 'd', disarm system
// If 'q', quit
if (isAnyKeyPressed())
{
char c = fgetc(stdin);
switch(c)
{
// TODO: Add code to look for q input and exit loop
// TODO: Add code to look for a or d and handle arm/disarm
}
}
if (!quit)
{
// Sleep for 1 second
usleep(1000000);
// TODO: Add to code decrement the timeout by one
// TODO: When the timeout reaches zero:
// - send a pulse message to keep connection alive
// - reload the timeout variable with TIMEOUT_SECONDS
}
}
return EXIT_SUCCESS;
}
}
// Set remote port
remotePort = SERVER_LISTENER_PORT;
// Setup pins as inputs
// TODO: Add code to configure the door button
// and motion sensor pins are inputs
// Send disarmed message
sendUdpMessage(remoteIp, remotePort, "disarmed");
// Loop until 'q' is pressed on keyboard
while (!quit)
{
// TODO: Add code to read the door and motion inputs
// and send messages and display on screen
// If key pressed on keyboard, get key and process
// If 'a', arm system
// If 'd', disarm system
// If 'q', quit
if (isAnyKeyPressed())
{
char c = fgetc(stdin);
switch(c)
{
// TODO: Add code to look for q input and exit loop
// TODO: Add code to look for a or d and handle arm/disarm
}
}
if (!quit)
{
// Sleep for 1 second
usleep(1000000);
// TODO: Add to code decrement the timeout by one
// TODO: When the timeout reaches zero:
// - send a pulse message to keep connection alive
// - reload the timeout variable with TIMEOUT_SECONDS
}
}
return EXIT_SUCCESS;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C++ Programmingarrow_forwardCOMPLETE TO DO's (do not copy other responses to this question if you do ill downvote) recv.cpp #include <stdio.h>#include <stdlib.h>#include <string.h>#include <fcntl.h>#include <mqueue.h>#include <sys/stat.h>#include <sys/types.h>#include <sys/mman.h>#include <unistd.h>#include <signal.h> // The name of the shared memory segment#define MSQ_NAME "/cpsc351queue" // The name of the file where to save the received file#define RECV_FILE_NAME "file__recv" #define MQ_MSGSIZE 4096 /*** Receive the file from the message queue*/void recvFile(){ // TODO: Define a data structure // of type mq_attr to specify a // queue that can hold up to 10 // messages with the maximum message // size being 4096 bytes // The buffer used to store the message copied // copied from the shared memory char buff[MQ_MSGSIZE]; // The total number of bytes written int totalBytesWritten = 0; // The number of bytes written int…arrow_forwardC++ programming task. main.cc file: #include <iostream>#include <map>#include <vector> #include "plane.h" int main() { std::vector<double> weights{3.2, 4.7, 2.1, 5.5, 9.8, 7.4, 1.6, 9.3}; std::cout << "Printing out all the weights: " << std::endl; // ======= YOUR CODE HERE ======== // 1. Using an iterator, print out all the elements in // the weights vector on one line, separated by spaces. // Hint: see the README for the for loop syntax using // an iterator, .begin(), and .end() // ========================== std::cout << std::endl; std::map<std::string, std::string> abbrevs{{"AL", "Alabama"}, {"CA", "California"}, {"GA", "Georgia"}, {"TX", "Texas"}}; std::map<std::string, double> populations{ {"CA", 39.2}, {"GA", 10.8}, {"AL", 5.1}, {"TX", 29.5}}; std::cout <<…arrow_forward
- #include <iostream>#include <sstream>#include <string>using namespace std; int main() { string userItem; ostringstream itemsOSS; cout << "Enter items (type Exit to quit):" << endl; cin >> userItem; while (userItem != "Exit") { cin >> userItem; } cout << itemsOSS.str() << endl; return 0;}arrow_forwardC++ programmingarrow_forwardC++main.cc file #include <iostream>#include <map>#include <vector> #include "bank.h" int main() { // =================== YOUR CODE HERE =================== // 1. Create a Bank object, name it anything you'd like :) // ======================================================= // =================== YOUR CODE HERE =================== // 2. Create 3 new accounts in your bank. // * The 1st account should belong to "Tuffy", with // a balance of $121.00 // * The 2nd account should belong to "Frank", with // a balance of $1234.56 // * The 3nd account should belong to "Oreo", with // a balance of $140.12 // ======================================================= // =================== YOUR CODE HERE =================== // 3. Deactivate Tuffy's account. // ======================================================= // =================== YOUR CODE HERE =================== // 4. Call DisplayBalances to print out all *active* // account…arrow_forward
- C PROGRAMarrow_forwardthis in r languagearrow_forwardPEN and PAPER Debugging the Pseudocode. Hand write the correct pseudocode using pen and paper. for DEBUG03-02.txt: // This pseudocode is intended to display employee net pay values.// All employees have a standard $45 deduction from their checks.// If an employee does not earn enough to cover the deduction,// an error message is displayed.start Declarations string name num hours num rate string DEDUCTION = 45 string EOFNAME = "ZZZ" num gross num net output "Enter first name or ", EOFNAME, " to quit" input name if name not equal to EOFNAME output "Enter hours worked for ", name input hours output "Enter hourly rate for ", name input rate gross = hours * rate net = gross - DEDUCTION while net > 0 then output "Net pay for ", name, " is ", net else output "Deductions not covered. Net is 0." endwhile output "Enter next name or ", EOFNAME, " to quit" input name endif…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
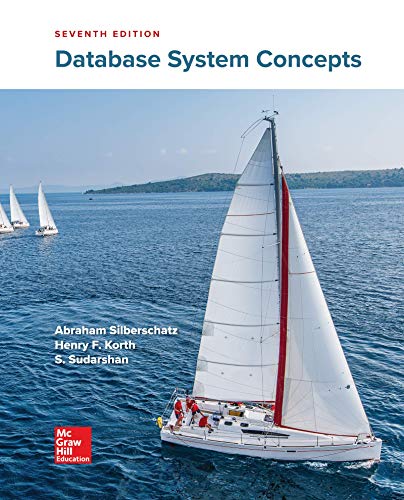
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
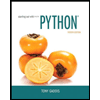
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
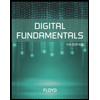
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
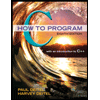
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
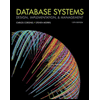
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
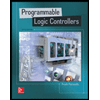
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education