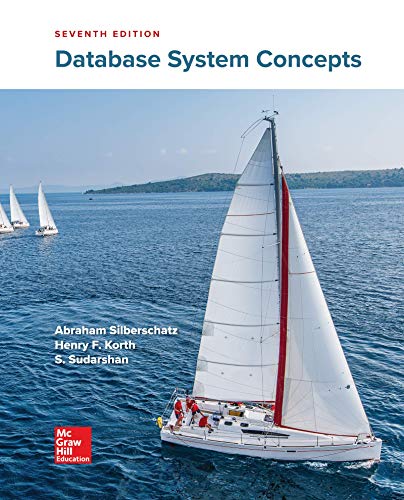
Concept explainers
C++
This program will store structure data in a file called Customers.dat.
For this assignment, create a menu with the following tasks:
1. Add DataX
. Exit Program
This menu needs to display "Invalid choice" if '1' or 'X' is not the given choice. The menu should redisplay options until item 'X' is selected.
Prior to this menu loop, the program needs to create the Customers.dat file if it does not existThe file "Customers.dat" must be opened in Binary mode.
The file is opened for processing prior to the menu.
This program may be written to automatically reset an existing Customers.dat file if it already exists.
For option '1', prompt to enter the highlighted data items in the structure below. This is every item except customerNumber , isDeleted and newLine;
const int NAME_SIZE = 20;
const int STREET_SIZE = 30;
const int CITY_SIZE = 20;
const int STATE_CODE_SIZE = 3;
struct Customers {
long customerNumber;
char name[NAME_SIZE];
char streetAddress_1[STREET_SIZE];
char streetAddress_2[STREET_SIZE];
char city[CITY_SIZE];
char state[STATE_CODE_SIZE];
int zipCode;
char isDeleted;
char newLine;
};
Always set the item isDeleted to 'N' and newline to '\n'. The item newLine is a convenient item that is there to assist in viewing the contents of the file using "type filename" in the cmd window.
The item customerNumber should start at 0 and increase by 1 for every record written. This data item is not viewable in the file via notepad because it is stored in a binary format.
Notepad will show the binary chars and will not line up the data as expected. You may see some odd characters after the expected data for the character arrays. That is normal for C/C++.
Once the data in the structure is loaded, append the structure to the file "Customers.dat" and return to the menu.
For option 'X':
Close the data file
display "Done!"
Let the program terminate.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- C++ Visual 2019 A file called num.txt. write a C++ program that opens a file, reads all the numbers from the file and calculates the following: The number of numbers in the file The sum of all the numbers in the file (a running total) The average of all the numbers in the file The program should display the number of numbers found in the file, the sum of the numbers and the average of the numbers. These are the numbers in the num.txt file :…arrow_forwardC++arrays, c-strings, functions with arrays as parameters 1. Create a file that contains 20 integers or download the attached file twenty_integers.txt Create a program that: 2. Declares a c-style string array that will store a filename. 3. Prompts the user to enter a filename. Store the file name declared in the c-string. 4. Opens the file. Write code to check the file state. If the file fails to open, display a message and exit the program. 5. Declare an array that holds 20 integers. Read the 20 integers from the fileinto the array.6. Write a function that accepts the filled array as a parameter and determines the MAXIMUM value in the array.Return the maximum value from the function (the function will be of type int).7. Print ALL the array values AND print the maximum value in the array using a range-based for loop. Use informational messages.Ensure the output is readable.arrow_forwardFile Handling Assignment -2 Write a program to generate a file numbers.txt that contains the first 50 odd natural numbers and each line in the file should contain 5 numbers only. Attach the screenshot of numbers.txt. In C or C++arrow_forward
- Add comments for Program 04arrow_forwardC programarrow_forwardC lanaguge Managing Student Information! C LANGUAGE We're going to build a student information management system. The user should be shown a menu with four options: display student initials, display student GPAs, add students, or exit the program. If the user chooses to exit, the program should end. Otherwise, if the user chooses to display the student initials, the contents of the students.txt file should be read, and just the student number and initials should be displayed to the user in a tabular format. If the user chooses to display the student GPAs, the contents of the students.txt file should be read, and just the student number and GPAs should be displayed to the user in a tabular format. If the user chooses to add students, they should be prompted for the number of studnets they’d like to add. They should then be prompted that many times for a studnet number, a GPA, a first initial, and a last initial. Each of the new items should be saved to the students.txt file.…arrow_forward
- File redirection allows you to redirect standard input (keyboard) and instead read from a file specified at the command prompt. It requires the use of the < operator (e.g. java MyProgram < input_file.txt) True or Falsearrow_forward// This pseudocode should create a list that describes annual profit // statistics for a retail store. Input records contain a department // name (for example, ìCosmeticsî) and profits for each quarter for // the last two years. The program should determine whether // the profit is higher, lower, or the same // for this full year compared to the last full year. start Declarations string department num salesQuarter1ThisYear num salesQuarter2ThisYear num salesQuarter3ThisYear num salesQuarter3ThisYear num salesQuarter1LastYear num salesQuarter2LastYear num salesQuarter3ThisYear num salesQuarter4LastYear num totalThisYear num totalLastYear string status num QUIT = "ZZZZ" housekeeping() while department <> QUIT compareProfit() endwhile finishUp() stop housekeeping()DEBUG04-02.txt output "Enter department name or ", QUIT, " to quit " input dept return compareProfit()…arrow_forward#function read file and display reportdef displayAverage(fileName):#open the fileinfile = open(fileName, "r")#variable declaration and initializationtotal = 0count = 0#read filefor num in infile:total = total + float(num)count = count + 1#close the fileinfile.close()#calculate averageaverage = total / count#display the resultprint("Average {:.1f}".format(average)) #method callingdisplayAverage("numbers.txt")arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
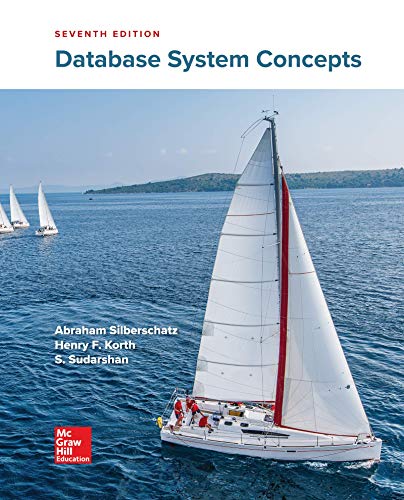
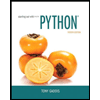
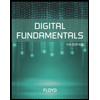
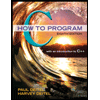
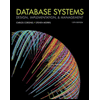
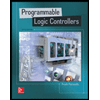