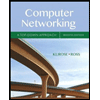
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
C programming:
Fill Out the TODO'S IN THE PROGRAM :
#include <stdlib.h> // EXIT_ codes
#include <stdbool.h> // bool
#include <stdio.h> // printf, scanf
#include <string.h> // strlen, strcmp
#include "udp.h" // udp library
#define CLIENT_LISTENER_PORT 4097
#define SERVER_LISTENER_PORT 4098
#define MAX_CHARS_PER_MESSAGE 80
//-----------------------------------------------------------------------------
// Main
//-----------------------------------------------------------------------------
int main(int argc, char* argv[])
{
char *remoteIp;
char *mode;
int remotePort;
char str[MAX_CHARS_PER_MESSAGE+1];
bool quit = false;
bool sendMode;
// Verify arguments are good
bool goodArguments = (argc == 3);
if (goodArguments)
{
remoteIp = argv[1];
mode = argv[2];
goodArguments = (strcmp(mode, "server") == 0) || (strcmp(mode, "client") ==
0);
}
if (!goodArguments)
{
printf("usage: chat IPV4_ADDRESS MODE\n");
printf(" where:\n");
printf(" IPV4_ADDRESS is address of the remote machine\n");
printf(" MODE is either client or server\n");
exit(EXIT_FAILURE);
}
// Determine if client or server
#include <stdbool.h> // bool
#include <stdio.h> // printf, scanf
#include <string.h> // strlen, strcmp
#include "udp.h" // udp library
#define CLIENT_LISTENER_PORT 4097
#define SERVER_LISTENER_PORT 4098
#define MAX_CHARS_PER_MESSAGE 80
//-----------------------------------------------------------------------------
// Main
//-----------------------------------------------------------------------------
int main(int argc, char* argv[])
{
char *remoteIp;
char *mode;
int remotePort;
char str[MAX_CHARS_PER_MESSAGE+1];
bool quit = false;
bool sendMode;
// Verify arguments are good
bool goodArguments = (argc == 3);
if (goodArguments)
{
remoteIp = argv[1];
mode = argv[2];
goodArguments = (strcmp(mode, "server") == 0) || (strcmp(mode, "client") ==
0);
}
if (!goodArguments)
{
printf("usage: chat IPV4_ADDRESS MODE\n");
printf(" where:\n");
printf(" IPV4_ADDRESS is address of the remote machine\n");
printf(" MODE is either client or server\n");
exit(EXIT_FAILURE);
}
// Determine if client or server
bool client = strcmp(mode, "client") == 0;
// TODO: Open udp listener port number dependent on client/server role
// TODO: Determine remote port that you will send data to
// If you are server, send to client port, and vice versa
// TODO: Determine if it is your turn to send data or not,
// based on server or client mode
// sendMode = ...
// Display warning to not use spaces
printf("NOTE: When sending messages, DO NOT include spaces\n");
// Start chat loop, alternating between sending and receiving messages
while(!quit)
{
// send text
if (sendMode)
{
// TODO: Add code to get string from user and send
// TODO: Handle an error is sendUdpMessage returns false
// TODO: Leave while loop if QUIT was sent
}
// wait for text
else
{
// TODO: Add code to wait for a string from remote user
// TODO: Leave while loop if QUIT is received
}
// alternate between send and receive mode
sendMode = !sendMode;
}
// TODO: Close udp listener port
return EXIT_SUCCESS;
}
// TODO: Open udp listener port number dependent on client/server role
// TODO: Determine remote port that you will send data to
// If you are server, send to client port, and vice versa
// TODO: Determine if it is your turn to send data or not,
// based on server or client mode
// sendMode = ...
// Display warning to not use spaces
printf("NOTE: When sending messages, DO NOT include spaces\n");
// Start chat loop, alternating between sending and receiving messages
while(!quit)
{
// send text
if (sendMode)
{
// TODO: Add code to get string from user and send
// TODO: Handle an error is sendUdpMessage returns false
// TODO: Leave while loop if QUIT was sent
}
// wait for text
else
{
// TODO: Add code to wait for a string from remote user
// TODO: Leave while loop if QUIT is received
}
// alternate between send and receive mode
sendMode = !sendMode;
}
// TODO: Close udp listener port
return EXIT_SUCCESS;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

Knowledge Booster
Similar questions
- In C++, using STL algorithms create a program that allows the user to search the text file for a price, and return all items for the inputted price. Console: Enter the command: cin>> search What price do you want to search for? cin>> $10 cout << "The following flavors are $10 " << flavor << flavor << endl; --------------------------------------------------------------------- Strawberry and rainbow should be returned for $10 If no flavor is $10: cout<<"Sorry, that price is not available"; Provided text file: icecream.txt chocolate $15 strawberry $10 vanilla $13 rainbow $10arrow_forwardPython Programming only plz Create a program to perform the following actions: Create a module that will determine if a string is an email address in this pattern XXXX@XXXX.XXX. you cannot use the regex library. Create a module that determines if a string is a phone number in this pattern XXX-XXX-XXXX. you cannot use the regex library. Then prompt the user: Enter an email address, check to see if the e-mail address is in the correct format. If not ask the user to enter an e-mail address in the correct format. If correct print e-mail accepted. Enter a phone number, check to see if the phone number is in the correct format. If not ask the user to enter a phone number in the correct format. If correct print phone accepted. Print the following: The phone number entered The email address entered Describe the numbers and text you print. Do not just print numbers or strings to the screen explain what each number represents.arrow_forwardWhat happens when a programmer attempts to access a node's data fields when the node variable refers to None? How do you guard against it? *PYTHONarrow_forward
- Caesars Cypher in C programming language How do you program an encryption and decryption for a Caesars Cypher that covers all the possible ASCII characters? In this there should be a shift over K = 5 but the example below is K = 3. This should result in actual character and not the control ASCII represenations. For example: >>> $ encrypt(I have a key)N%mf{j%f%pj~>>> $ encrypt(see me at 3)xjj%rj%fy%8>>> $ decrpyt(|jfw%ns%gqzj)wear in blue Assume that the text parsing method has been succesful and it's the encryption/decryption of the char array that needs to be factored. Formula Encryption -> C = E(k, P) = (P + k) (mod 26) Decryption -> P = D(k, C) = (C - k) (mod 26) Where k = 5arrow_forwardample code for the reader-writer: #include <pthread.h>#include <semaphore.h>#include <stdio.h> /*This program provides a possible solution for first readers writers problem using mutex and semaphore.I have used 10 readers and 5 producers to demonstrate the solution. You can always play with these values.*/ sem_t wrt;pthread_mutex_t mutex;int cnt = 1;int numreader = 0; void *writer(void *wno){ sem_wait(&wrt);cnt = cnt*2;printf("Writer %d modified cnt to %d\n",(*((int *)wno)),cnt);sem_post(&wrt); }void *reader(void *rno){ // Reader acquire the lock before modifying numreaderpthread_mutex_lock(&mutex);numreader++;if(numreader == 1) {sem_wait(&wrt); // If this id the first reader, then it will block the writer}pthread_mutex_unlock(&mutex);// Reading Sectionprintf("Reader %d: read cnt as %d\n",*((int *)rno),cnt); // Reader acquire the lock before modifying numreaderpthread_mutex_lock(&mutex);numreader--;if(numreader == 0) {sem_post(&wrt); //…arrow_forwardPython Programmingarrow_forward
- C PROGRAMMING C90 modify the following code so it connects to a tcp port #include <stdio.h>#include <stdlib.h>#include <string.h>#include <sys/types.h>#include <sys/socket.h>#include <netinet/in.h>#include <unistd.h> #include "graph.h" // Include the graph library#include "dijkstra.h" // Include the Dijkstra's algorithm implementation #define MAX_LINE_LENGTH 512 // Maximum length of a line of text#define MAX_NETWORKS 255 // Maximum number of networks#define MAX_CONNECTIONS ((MAX_NETWORKS * (MAX_NETWORKS - 1)) / 2) // Maximum number of connections between networks#define TIMEOUT_SECS 10 // Timeout in seconds for waiting for greeting and QUIT response // Struct to represent a graphstruct graph { int num_vertices; // Number of vertices in the graph int num_edges; // Number of edges in the graph int *vertices; // Array of vertices int *edges; // Array of edges}; // Function to create a graphstruct graph *create_graph(int num_vertices, int…arrow_forwardC programming: Fill Out the TODO'S IN THE PROGRAM : #include <stdlib.h> // EXIT_ codes#include <stdbool.h> // bool#include <stdio.h> // printf, scanf#include <string.h> // strlen, strcmp#include "udp.h" // udp library#define CLIENT_LISTENER_PORT 4097#define SERVER_LISTENER_PORT 4098#define MAX_CHARS_PER_MESSAGE 80//-----------------------------------------------------------------------------// Main//-----------------------------------------------------------------------------int main(int argc, char* argv[]){char *remoteIp;char *mode;int remotePort;char str[MAX_CHARS_PER_MESSAGE+1];bool quit = false;bool sendMode;// Verify arguments are goodbool goodArguments = (argc == 3);if (goodArguments){remoteIp = argv[1];mode = argv[2];goodArguments = (strcmp(mode, "server") == 0) || (strcmp(mode, "client") ==0);}if (!goodArguments){printf("usage: chat IPV4_ADDRESS MODE\n");printf(" where:\n");printf(" IPV4_ADDRESS is address of the remote machine\n");printf(" MODE is…arrow_forward#ifndef NODES_LLOLL_H#define NODES_LLOLL_H #include <iostream> // for ostream namespace CS3358_SP2023_A5P2{ // child node struct CNode { int data; CNode* link; }; // parent node struct PNode { CNode* data; PNode* link; }; // toolkit functions for LLoLL based on above node definitions void Destroy_cList(CNode*& cListHead); void Destroy_pList(PNode*& pListHead); void ShowAll_DF(PNode* pListHead, std::ostream& outs); void ShowAll_BF(PNode* pListHead, std::ostream& outs);} #endif #include "nodes_LLoLL.h"#include "cnPtrQueue.h"#include <iostream>using namespace std; namespace CS3358_SP2023_A5P2{ // do breadth-first (level) traversal and print data void ShowAll_BF(PNode* pListHead, ostream& outs) { cnPtrQueue queue; CNode* currentNode; queue.push(lloLLPtr->getHead()); while (!queue.empty()) { currentNode = queue.front(); queue.pop(); if…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
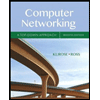
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
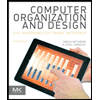
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
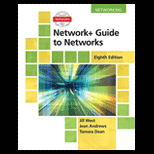
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
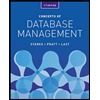
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
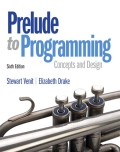
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
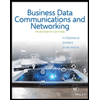
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY