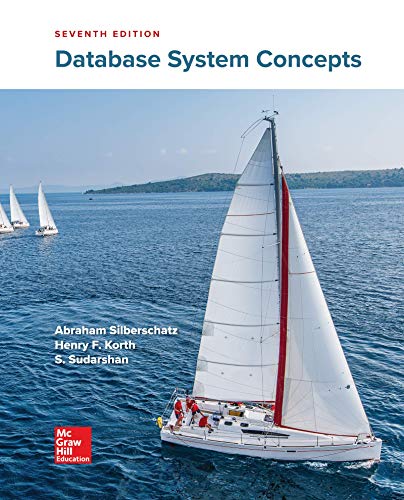
C PROGRAMMING
Implement dijkstras alorithm
Check that the Graph graph, and starting node, id, are valid
• Create the set S containing all the networks (vertices) except the source node (you might want
to use an array for this.
• Create an array to represent the table D and initialise it with the weights of the edges from the
source node, or infinity if no edge exists. You should use the constant DBL_MAX to represent
infinity.
• Create an array to represent the table R and initialise it with the next hops if an edge exists
from the source, or 0 otherwise.
• Then repeatedly follow the remaining rules of Dijkstra’s
R until S is empty.
• Each of the values required to complete the above can be found by calling the various
functions (get_vertices(), get_edge(), edge_destination(), edge_weight(), etc.)
in the supplied graph library.
• Once Dijkstra’s algorithm has run, you will need to create the routing table to be returned by
allocating enough memory for the required number of Path structures and filling each entry of
the array.
• Finally, you should free() any unused memory you have allocated, set *pnEntries to the
correct value, and return the pointer to the routing table you’ve just filled in (obviously, you
shouldn’t free that!)
using the below as a starting point:
/*
* dijkstra.c
* ProgrammingPortfolio
*
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <float.h>
#include "graph.h"
#include "dijkstra.h"
/* find shortest paths between source node id and all other nodes in graph. */
/* upon success, returns an array containing a table of shortest paths. */
/* return NULL if *graph is uninitialised or an error occurs. */
/* each entry of the table array should be a Path */
/* structure containing the path information for the shortest path between */
/* the source node and every node in the graph. If no path exists to a */
/* particular desination node, then next should be set to -1 and weight */
/* to DBL_MAX in the Path structure for this node */
Path *dijkstra(Graph *graph, int id, int *pnEntries)
{
Path *table = NULL;
/* Insert your implementation of Dijkstra's algorithm here */
return table;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- C programming I need a code that asks the user for how many times it'll run and run that many time and ask the user for a number and it puts that number into a binary search tree and an array using diffrent functionsarrow_forwardProblem Description Inversion Count for an array indicates – how far (or close) the array is from being sorted. If array is alreadysorted then inversion count is 0. If array is sorted in reverse order that inversion count is the maximum.Formally speaking, two elements a[i] and a[j] form an inversion if a[i] > a[j] and i < j .Example: The sequence 2, 4, 1, 3, 5 has three inversions (2, 1), (4, 1), (4, 3).Requirement Design should be based on divide and conquer.Running time should NOT be worse than .Must use recursion to solve the subproblems. Input Specification Your program should read from an input file, which starts with a line indicating the number of test cases.Each of the following lines indicates a test case, and each test case includes a sequence of numbers(separated by spaces) for which you need to count inversions. Sample Input 32 4 1 3 51 2 4 8 9 3 5 6 1 20 6 4 5Output Specification For each test case, print one line with a format of The sequence has ? inversions in…arrow_forwardConsider the graph below Can you determine whether this graph is bidirectional or not? Write a program that will store this graph as a matrix ( a 2D array) You don’t have to print out the elements Write a program that will store this graph as a 1D array of vectors and print the elements Extra credit: The subroutine included in the reference below (void printGraph) for the array of vectors ( question c) is not ideal. Consider modifying it to reference each node(vertex) by the size of the vector for each array element. So it would be ideal to replace for( auto x : adj[v]) with something like for (int j = 0; j < adj[v].size(); j++) cout << adj[v][j] << " "; Regardless, add a function to the program that will map the label to the nodes to the following characters: [ 0 → ‘cat’, 1→ ‘dog’, 2 → ‘car’, 3 → ‘dub’, 4 → ‘boo’, 5 → ‘foo’, 6 → ‘doo’ ]. Which means that when you call function, myfunc(4) it should return ‘boo’. And then adding a secondary…arrow_forward
- Function PrintArray(integer array(?) dataList) returns nothing integer i for i = 0; i < dataList.size; i = i + 1 dataList[i] = Get next input Put dataList to output Put "_" to output // Your solution goes here. Modify as needed i = 0 Complete the PrintArray function to iterate over each element in dataList. Each iteration should put the element to output. Then, put "_" to output. Ex: If dataList's elements are 2 4 7, then output is: 2_4_7_ Function Main() returns nothing integer array(3) userNums integer i for i = 0; i < userNums.size; i = i + 1 userNums[i] = Get next input PrintArray(userNums)arrow_forwardC++ code using dynamic programming. In the graph, determine the cycle's length. A cycle is a path that has the same node as its beginning and ending points and only visits each edge of the path once. The number of edges a cycle visits determines its length. After including the edge of the query, the tree may contain many edges connecting two nodes. Return an array answer of lengthm where answer [i] is the answer to the 1th query. Example 1: Input: n = 3, queries = [[5,3], [4,7], [2,3]] Output: [4,5,3]arrow_forwardHow does a link-based implementation of the List differ from an array-based implementation? Select one: a. All of these b. A link-based implementation does not need to shift entries over to make room when adding a new entry to the List c. A link-based implementation is sized dynamically so it takes up only the memory to hold the current entries d. A link-based implementation does not need to shift entries up to remove a gap when removing an entry from the Listarrow_forward
- Using Fundamental Data Structures Purpose: The purpose of this: Design and develop Applications that incorporate fundamental data structures such as: Singly Linked Lists Doubly Linked Lists Circularly Linked Lists Exercise 3 If your first name starts with a letter from A-J inclusively: Implement the clone() method for the CircularlyLinkedList class. Make sure to properly link the new chain of nodes. If your first name starts with a letter from K-Z inclusively: Let L1 and L2 be two circularly linked lists created as objects of CircularlyLinkedList class from Lesson. Write a method that returns true if L1 and L2 store the same sequence of elements (but perhaps with different starting points). Write the main method to test the new method. Hint: Try to find a matching alignment for the first node of one list.arrow_forwardHippity hoppity, abolish loopity def frog_collision_time(frog1, frog2): A frog hopping along on the infinite two-dimensional lattice grid of integers is represented as a 4- tuple of the form (sx, sy, dx, dy) where (sx, sy) is its starting position at time zero, and (dx, dy) is its constant direction vector for each hop. Time advances in discrete integer steps 0, 1, 2, 3, ... so that each frog makes one hop at every tick of the clock. At time t, the position of that frog is given by the formula (sx+t*dx, sy+t*dy) that can be nimbly evaluated for any t. Given two frogs frog1 and frog2 that are guaranteed to initially stand on different squares, return the time when both frogs hop into the same square. If these two frogs never simultaneously arrive at the same square, return None. This function should not contain any loops whatsoever. The result should be calculated using conditional statements and integer arithmetic. Perhaps the best way to get cracking is to first solve a simpler…arrow_forwardBackground: This assignment will help you further learn to use multidimensional arrays. You will need a 10x2 matrix for this problem. Consider 10 points on a map, You need to identify the distance between each point (or vertex). A vertex requires two pieces of information. An x,y coordinate (think longitude and latitude.) This is why you have a matrix of 10x2, each entry is the two values you will need. You can think of these points a cities, or friend's houses. We're only looking for a direct route (as the crow flies) not taking roads to make it simpler. You can find the distance between any two points by use of: sqr_root((x1 - x2)^2 + (y1 - y2)^2) You will need to fill your matrix with some random values. Then you will need to calculate the distance between each possible point, eventually displaying it on a matrix in output. Think something like: 1 2 3 4 5 1 0 8 12 4 6 2 8 0 7 .... 3 12 7 .... 4 4 ... 5 6 ... Hint:…arrow_forward
- Data Structure and algorithms ( in Java ) Please solve it urgent basis: Make a programe in Java with complete comments detail and attach outputs image: Question is inside the image also: a). Write a function to insert elements in the sorted manner in the linked list. This means that the elements of the list will always be in ascending order, whenever you insert the data. For example, After calling insert method with the given data your list should be as follows: Insert 50 List:- 50 Insert 40 List:- 40 50 Insert 25 List:- 25 40 50 Insert 35 List:- 25 35 40 50 Insert 40 List:- 25 35 40 40 50 Insert 70 List:- 25 35 40 50 70 b). Write a program…arrow_forwardDouble linked list in Java Double linked list Textbook reference Data structures and algorithms Micheal Goodricharrow_forwardCode in Python:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
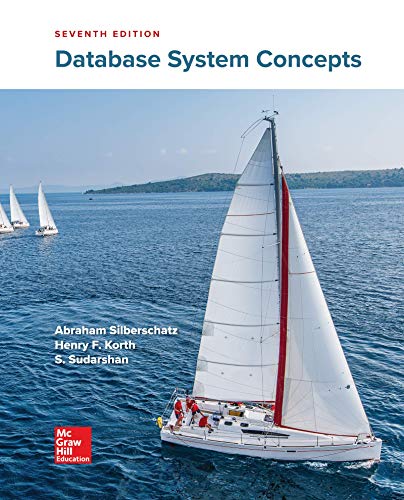
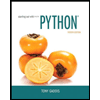
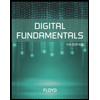
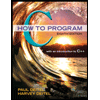
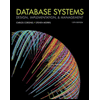
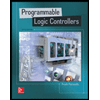