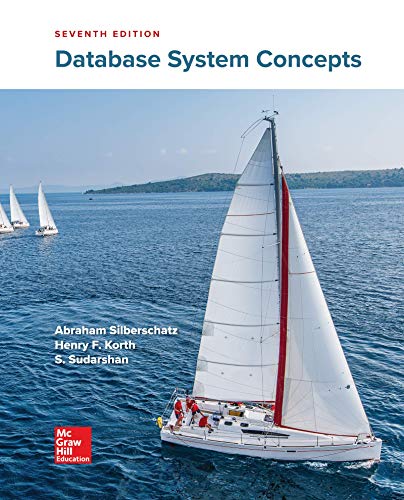
C programming:
Use following code and modify in the end to get median. Sorting is not needed as it already sorted.
#include <stdio.h>
#define MAX_SCORE 20
#define MAX_SIZE 1000
int main() {
int i = 0 ; // used as index into array
int n = 0 ; // number of items in array
int r ; // used to ensure user enters an integer
double average ; // average of numbers entered by user
double num ; // total number of items in array
int A[MAX_SIZE] ; // will hold the scores
n = 0 ;
i = 0 ;
// read in the scores into array A[]
while (1) {
r = scanf("%d", &A[i] ) ;
// end of input?
if ( r <= 0 ) {
break ;
}
i = i + 1 ;
// exceeded array size?
if (i >= MAX_SIZE) {
break ;
}
}
// Make n the number of items stored in A[]
n = i ;
// compute the average
int sum = 0 ;
for (i = 0 ; i < n ; i++) {
sum = sum + A[i] ;
}
num = n ; // num is double
average = sum / num ;
printf ("The average score is: %f\n", average) ;
// *****
// ***** TODO
// *****
// Declare a new count[] array.
int count[MAX_SCORE + 1] ;
// Initialize each element of count[] to 0 using a for loop.
for(i = 0; i < (MAX_SCORE + 1); i++) {
count[i]=0;
}
// Iterate through the elements of the A[] array and update count[] in
// each iteration.
for(i = 0; i < n; i++) {
count[A[i]]++;
}
//
// Iterate through the count[] array and print out the number of times
// that each score appeared.
for(i = 0; i < (MAX_SCORE + 1); i++) {
printf("count[%d] is %d. \n",i,count[i]);
}
// Iterate through the elements of the count[] array to find the maximum
// count and the score with the maximum count.
int mode = 0;
for(i = 0; i < (MAX_SCORE + 1); i++) {
if(count[i] > count[mode]) {
mode = i;
}
}
// Print out the score that has the highest count and the number of
// times that score appeared.
printf("The mode of the scores is %d. It occurred %d times.\n",mode,count[mode]);
return 0 ;
}
Example Compilation and Execution:
The number of scores is 21.
The average score is: 13.047619
count[0] is 0.
count[1] is 1.
count[2] is 2.
count[3] is 0.
count[4] is 0.
count[5] is 0.
count[6] is 0.
count[7] is 1.
count[8] is 1.
count[9] is 1.
count[10] is 0.
count[11] is 1.
count[12] is 2.
count[13] is 1.
count[14] is 0.
count[15] is 1.
count[16] is 2.
count[17] is 2.
count[18] is 1.
count[19] is 2.
count[20] is 3.
The mode of the scores is 20. It occurred 3 times.
The median score is 15.
Notes:
Think about how to calculate the median. You already have the count[ ] array computed.
If you iterate through the count[ ] array, you should be able to determine when half of the scores (n/2 when n is even and (n+1)/2 when n is odd) have been encountered. Then you have the median. Do this by hand on a small example first.
NOTE: There is no need to modify the A array to find the median. Do NOT modify it!

Step by stepSolved in 3 steps with 4 images

- use c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forwardA1: File Handling with struct C++ This program is to read a given file and display the information about employees according to the type of employee. Specifically, the requirements are as follows. Read the given file of the information of employees. Store the information in an array or arrays. Display all the salaried employees first and then the hourly employees Prompt the user to enter an SSN and find the corresponding employee and display the information of that employee. NOTE : S- salaryemployee , H- Hourly employee S 135-25-1234 Smith Sophia DevOps Developer 70000H 135-67-5462 Johnaon Jacob SecOps Pentester 30 60.50S 252-34-6728 William Emma DevOps DBExpert 100000S 237-12-1289 Miller Mason DevOps CloudArchitect 80000S 581-23-4536 Jones Jayden SecOps Pentester 250000S 501-56-9724 Rogers Mia DevOps Auditor 90000H 408-67-8234 Cook Chloe DevOps QAEngineer 40 45.10S 516-34-6524 Morris Daniel DevOps ProductOwner 300000H 526-47-2435 Smith Natalie DevOps…arrow_forwardWrite a function to determine the resultant force vector R of the two forces F₁ and F2 applied to the bracket, where 0₁ and 0₂. Write R in terms of unit vector along the x and y axis. R must be a vector, for example R = [Rx, Ry]. The coordinate system is shown in the figure below: F₁ y 0₂ 0₁ 1 ►x F2arrow_forward
- In C, in order to do operations to the data being referenced by a pointer you would need to use the (*) operator, which is called ______________ operator.arrow_forwardC++ c++ write a hangman game that randomly generates a word and prompts the user to guess one letter at a time, as shown in the sample run. Each letter in the word is displayed as asterisk. when the user makes a correct guess, the actual letter is then displayed. When the user finishes a word, display the number of misses and ask the user whether to continue to play with another word. Declare an array to store word as follow: Store 20 different words in a text file, read them into an array. Randomly pick a number 1..20 and use this word for the current round.arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- #include using namespace std; int main() { // Declare two dimensional array here // Declare other variables int numDays; int age; int QUIT = 99; // This is the work done in the getReady () function // Perform a priming read to get the age of the child while (age != QUIT) { // This is the work done in the determineRateCharge() function // Ask the user to enter the number of days // Print the weekly rate // Ask the user to enter the next child's age } // This is the work done in the finish() function cout << "End of program" << endl; return 0; } // End of main() functionarrow_forward#include <iostream> #include <string> using namespace std; int main() { // Declare a named constant for array size here // Declare array here // Use this integer variable as your loop index int loopIndex; // Use this variable to store the batting average input by user double battingAverage; // Use these variables to store the minimim and maximum values double min, max; // Use these variables to store the total and the average double total, average; // Write a loop to get batting averages from user and assign to array cout << "Enter a batting average: "; cin >> battingAverage; // Assign value to array // Assign the first element in the array to be the minimum and the maximum min = averages[0]; max = averages[0]; // Start out your total with the value of the first element in the array total = averages[0]; // Write a loop here to access array values starting with…arrow_forwardPointers can be compared using the == operator. A) True B) Falsearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
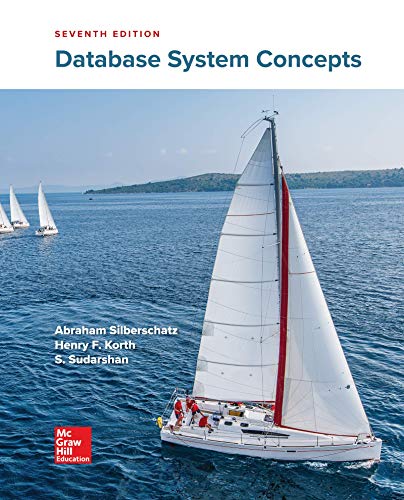
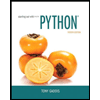
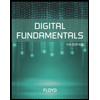
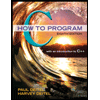
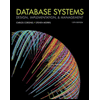
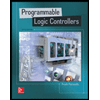