Can someone help me modify the below program to include the expiration date, then give the users a search choice. Provide two search options: 1) item, the way it is done in the program, and 2) expiration date #include #include #include using namespace std; struct Node { string item; int count; Node *link; }; typedef Node* NodePtr; NodePtr search(NodePtr head, string an_item); void head_insert(NodePtr& head, string an_item, int a_number); void show_list(NodePtr& head); int main() { NodePtr head = NULL; head_insert(head, "Tea", 2); head_insert(head, "Jam", 3); head_insert(head, "Rolls", 10); cout << "List contains:" << endl; show_list(head); string target; cout << "Enter an item to search for" << endl; cin >> target; NodePtr result = search(head, target); if (result == NULL) cout << target << " is not on the list." << endl; else cout << target << " is on the list." << endl; return 0; } NodePtr search(NodePtr head, string target) { // Point to the head node NodePtr here = head; // If the list is empty nothing to search if (here == NULL) { return NULL; } // Search for the item else { // while you have still items and you haven't found the target yet while (here-> item != target && here->link != NULL) here = here->link; // Found the target, return the pointer at that location if (here-> item == target) return here; // Search unsuccessful, return Null else return NULL; } } void head_insert(NodePtr& head, string an_item, int a_number) { NodePtr temp_ptr; temp_ptr = new Node; temp_ptr -> item = an_item; temp_ptr -> count = a_number; temp_ptr->link = head; head = temp_ptr; } void show_list(NodePtr& head) { NodePtr here = head; while (here != NULL) { cout << here -> item << endl; here = here ->link; } }
Can someone help me modify the below program to include the expiration date, then give the users a search choice. Provide
two search options:
1) item, the way it is done in the program, and
2) expiration date
#include <iostream>
#include <cstddef>
#include <string>
using namespace std;
struct Node
{
string item;
int count;
Node *link;
};
typedef Node* NodePtr;
NodePtr search(NodePtr head, string an_item);
void head_insert(NodePtr& head, string an_item, int a_number);
void show_list(NodePtr& head);
int main()
{
NodePtr head = NULL;
head_insert(head, "Tea", 2);
head_insert(head, "Jam", 3);
head_insert(head, "Rolls", 10);
cout << "List contains:" << endl;
show_list(head);
string target;
cout << "Enter an item to search for" << endl;
cin >> target;
NodePtr result = search(head, target);
if (result == NULL)
cout << target << " is not on the list." << endl;
else
cout << target << " is on the list." << endl;
return 0;
}
NodePtr search(NodePtr head, string target)
{
// Point to the head node
NodePtr here = head;
// If the list is empty nothing to search
if (here == NULL)
{
return NULL;
}
// Search for the item
else
{
// while you have still items and you haven't found the target yet
while (here-> item != target && here->link != NULL)
here = here->link;
// Found the target, return the pointer at that location
if (here-> item == target)
return here;
// Search unsuccessful, return Null
else
return NULL;
}
}
void head_insert(NodePtr& head, string an_item, int a_number)
{
NodePtr temp_ptr;
temp_ptr = new Node;
temp_ptr -> item = an_item;
temp_ptr -> count = a_number;
temp_ptr->link = head;
head = temp_ptr;
}
void show_list(NodePtr& head)
{
NodePtr here = head;
while (here != NULL)
{
cout << here -> item << endl;
here = here ->link;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

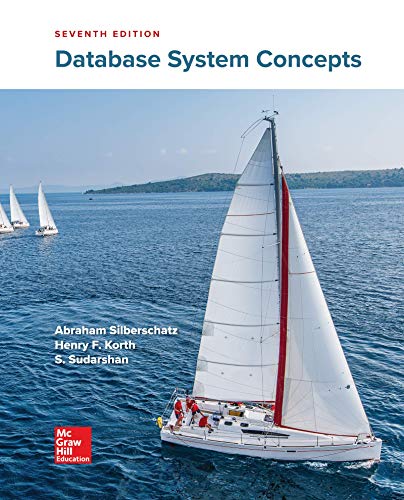
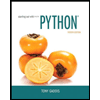
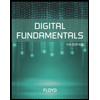
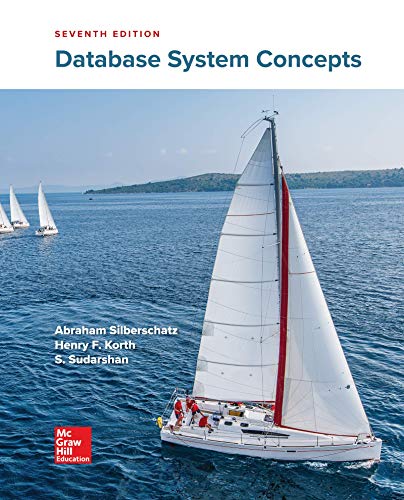
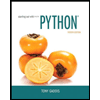
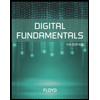
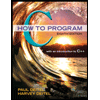
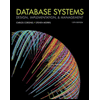
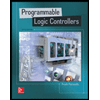