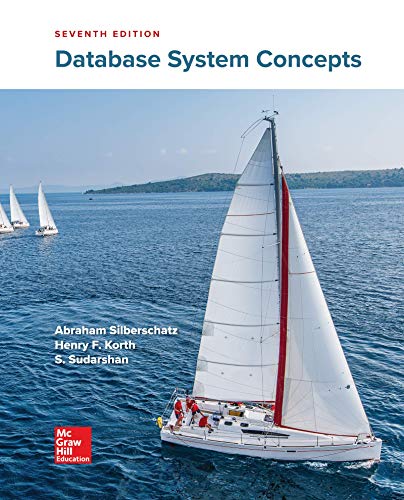
// Fix this program that works
// Use welcome function that display the message in diffreent color
// Use Draw function to diplay table in gree/yellow
// Remove, add, any code that you believe is not useful
#include <iostream>
#include <windows.h>
using namespace std;
char mat[3][3];
void table(); //function to print the table
void welcome(); //function for welcome screen
int main()
{
int i, j, m, n, sum = 0;
char ch;
welcome();
system("pause");
for (m = 0; m < 3; m++)
for (n = 0; n < 3; n++)
mat[m][n] ='W';
table();
while (sum < 10)
{
//================== for player 1 ===================
cout << "Player 1 is X Choose the position : ";
cout << "Row:";
cin >> i;
cout << "Coloumn:";
cin >> j;
//============== if position is wrong ============
for (; i > 3 || i < 1 || j>3 || j < 1 || ('x' == mat[i - 1][j - 1] || 'o' == mat[i - 1][j - 1]);)
{
cout << "Sorry!!!!wrong position, Choose the position again";
cout << "Row:";
cin >> i;
cout << "Coloumn:";
cin >> j;
}
mat[i - 1][j - 1] = 'x';
sum++;
//=============== to check if player 1 wins or not===================
if (mat[0][0] == 'x' && mat[0][0] == mat[0][1] && mat[0][0] == mat[0][2])
{
table();
cout << "Player 1 wins…….!!!";
cout << "You have played Great…..!!!";
//
break;
}
if (mat[1][0] == 'x' && mat[1][0] == mat[1][1] && mat[1][0] == mat[1][2])
{
table();
cout << "Player 1 wins…….!!!";
cout << "You have played Great…..!!!"<<endl;
break;
}
if (mat[2][0] == 'x' && mat[2][0] == mat[2][1] && mat[2][0] == mat[2][2])
{
table();
cout << "Player 1 wins…….!!!";
cout << "You have played Great…..!!!"<<endl;
break;
}
if (mat[0][0] == 'x' && mat[0][0] == mat[1][0] && mat[0][0] == mat[2][0])
{
table();
cout << "Player 1 wins…….!!!";
cout << "You have played Great…..!!!";
break;
}
if (mat[0][1] == 'x' && mat[0][1] == mat[1][1] && mat[0][1] == mat[2][1])
{
table();
cout << "Player 1 wins…….!!!";
cout << "You have played Great…..!!!";
break;
}
if (mat[0][2] == 'x' && mat[0][2] == mat[1][2] && mat[0][2] == mat[2][2])
{
table();
cout << "Player 1 wins…….!!!"<<endl;
cout << "You have played Great…..!!!"<<endl;
break;
}
if (mat[0][0] == 'x' && mat[0][0] == mat[1][1] && mat[0][0] == mat[2][2])
{
table();
cout << "Player 1 wins…….!!!"<<endl;
cout << "You have played Great…..!!!"<<endl;
break;
}
if (mat[0][2] == 'x' && mat[0][2] == mat[1][1] && mat[0][0] == mat[2][0])
{
table();
cout << "Player 1 wins…….!!!"<<endl;
cout << "You have played Great…..!!!"<<endl;
break;
}
if (sum == 9) //to check the chances
{
table();
cout << "The game is over…….no one wins…HaHaHa…..!!!";
break;
}
//for player 2
cout << "Player 2 is’o’nChoose the position : " << endl;
cout << "Row:";
cin >> i;
cout << "Coloumn:";
cin >> j;
//if position is wrong
for (; i > 3 || i < 1 || j>3 || j < 1 || ('x' == mat[i - 1][j - 1] || 'o' == mat[i - 1][j - 1]);)
{
cout << "Sorry!!!!wrong position, Choose the position again" << endl;
cout << "Row:"<<endl;
cin >> i;
cout << "Coloumn:" << endl;
cin >> j;
}
mat[i - 1][j - 1] = 'o';
sum++;
table();
![```cpp
cout << "Would you like to play more.... ? (y / n) : " << endl;
cin >> ch;
if (ch == 'y' || ch == 'Y')
system("cls");
else
{
cout << "Thanks for Playing.........:)" << endl;
exit(0);
}
return 0;
}
//======================== draw table ====================
void table()
{
system("cls");
cout << " 1 2 3 " << endl;
cout << "\t" << mat[0][0] << "|" << mat[0][1] << "|" << mat[0][2] << endl;
cout << "\t_ _ _ " << endl;
cout << "\t" << mat[1][0] << "|" << mat[1][1] << "|" << mat[1][2] << endl;
cout << "\t_ _ _ " << endl;
cout << "\t" << mat[2][0] << "|" << mat[2][1] << "|" << mat[2][2] << endl;
}
//========================
void welcome()
{
/* Change the color here for welcome message*/
cout << "Welcome To Tic - Tac - Toe Game" << endl;
cout << "Press any key to continue.....!!";
}
```
The code is a part of a console-based Tic-Tac-Toe game written in C++. It includes functions to display the welcome message, draw the game table, and ask the user if they want to play again.
**Key Components:**
1. **Replay Functionality:**
- Prompts the user with "Would you like to play more....? (y / n) :".
- If the user inputs 'y' or 'Y', the game screen refreshes; otherwise, it displays a thank you message and exits.
2. **Draw Table Function:**
- Clears the screen and prints the Tic-Tac-Toe board structure.
- The board is displayed with a 3x3 grid, showing current gameplay positions.
3. **Welcome Function:**
- Displays a welcome message for the Tic-Tac-Toe game.
- Prompts the player to press any key to start the game.
```](https://content.bartleby.com/qna-images/question/2c30b2c3-62fe-421c-b864-63a8dde6d2d9/fada8e8c-ef25-425a-a995-d77097886159/5zh4f78_thumbnail.png)
![```cpp
// to check player 2 wins or not
if (mat[0][0] == 'o' && mat[0][0] == mat[0][1] && mat[0][0] == mat[0][2])
{
cout << "Player 2 wins……!!!" << endl;
cout << "You have played Great…..!!!" << endl;
break;
}
if (mat[1][0] == 'o' && mat[1][0] == mat[1][1] && mat[1][0] == mat[1][2])
{
cout << "Player 2 wins……!!!" << endl;
cout << "You have played Great…..!!!" << endl;
break;
}
if (mat[2][0] == 'o' && mat[2][0] == mat[2][1] && mat[2][0] == mat[2][2])
{
cout << "Player 2 wins……!!!" << endl;
cout << "You have played Great…..!!!" << endl;
break;
}
if (mat[0][0] == 'o' && mat[0][0] == mat[1][0] && mat[0][0] == mat[2][0])
{
cout << "Player 2 wins……!!!" << endl;
cout << "You have played Great…..!!!" << endl;
break;
}
if (mat[0][1] == 'o' && mat[0][1] == mat[1][1] && mat[0][1] == mat[2][1])
{
cout << "Player 2 wins……!!!" << endl;
cout << "You have played Great…..!!!" << endl;
break;
}
if (mat[0][2] == 'o' && mat[0][2] == mat[1][2] && mat[0][2] == mat[2][2])
{
cout << "Player 2 wins……!!!" << endl;
cout << "You have played Great…..!!!" << endl;
break;
}
if (mat[0][0] == 'o' && mat[0][0] == mat[1][1] && mat[0][0] == mat[2][2])
{
cout << "Player 2 wins](https://content.bartleby.com/qna-images/question/2c30b2c3-62fe-421c-b864-63a8dde6d2d9/fada8e8c-ef25-425a-a995-d77097886159/f8wjgrj_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- This is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for (int i = 0; i < 9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees *e) { printf("%s", e->name); printf(" %d%d%d-%d%d-%d%d%d%d",…arrow_forward// MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing #include <iostream> #include <string> using namespace std; int main() { // Declare variables string inCity; // name of city to look up in array const int NUM_CITIES = 10; // Initialized array of cities string citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"}; bool foundIt = false; // Flag variable int x; // Loop control variable // Get user input cout << "Enter name of city: "; cin >> inCity; // Write your loop here // Write your test statement here to see if there is // a match. Set the flag to true if city is found. // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed.…arrow_forwardC programming:you are to design and write a menu driven program as shown by the sample menu below. should the user select [G] the program will ask the user to enter a single deposit into the bank account(this would be a 1D array) the rest of the menu options are self-explanatory. demonstrate a bubble sort, the usee of user preventive coding, switch statement, demonstrate the use of functions along will call by value and call by reference where necessary. Program Menu System (Your solution should display this menu) **** BANKING MAIN MENU*** [G]et a new deposit [S]um of all deposits…arrow_forward
- /courses/46018/assignments/296094 >>> sum_of_greatest two(3, 100.900) 1000 4. pet_shopping_list For the purposes of this question, if you have a pet of a certain type, that pet needs food, treats, a bed and a house -- but multiple animals of the same kind can share. This function takes what kind of animal or animals you have and returns a shopping list for your pet or pets. PLAN FOR THIS FUNCTION: determine the word for a single kind of the animal. if the input is "mice", then we want the output to contain "mouse" (don't worry about any other unusual plurals). Otherwise, look for words that end with "es" and remove the final "es", so "foxes" goes to "fox". Otherwise, remove a final "s". Otherwise, assume that the word is singular. build and return a shopping list, which will be a list of strings. shopping lists should be in the shape: ["mouse food", "mouse treats", "mouse bed", "mouse house"] Sample calls should look like: >>> pet_shopping list("mice") I'mouse food', 'mouse treats',…arrow_forward/*Line 1:*/ doublevalues[6] = {10, 20, 30, 40, 50, 60}; /*Line 2:*/ double* yalptr= values; /*Line 3:*/ cout << 3*values[1] + yalptr[3] + *(valptr + 2); A. What is 3*values[1] equal to in Line 3? (Explain) B. What is yalptr[3] equal to in Line 3? (Explain)arrow_forwardmain.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forward
- Common Time Zones Function Name: commonTimeZones() Parameters: code1( str ), code2( str) Returns: list of common time zones ( list ) Description: You and your friend live in different countries, but you figure there's a chance that both of you might be in the same time zone. Thus, you want to find out the list of possible time zones that you and your friend could both be in. Given two country codes, write a function that returns a list of the time zones the two countries have in common. Be sure not to include any duplicate time zones. If the two country codes do not have any common time zones, return the string 'No Common Time Zones' instead. Note: You can assume that the codes will always be valid. example test cases: >>> commonTimeZones('can', 'usa') [UTC-08:00', 'UTC-07:00', 'UTC-06:00', 'UTC-05:00', 'UTC-04:00] >>> commonTimeZones('rus', 'chn') [UTC+08:00] For this assignment, use the REST countries API (https://restcountries.com/#api-endpoints-v2). For all of your requests, make…arrow_forwardC++ Given code is #pragma once#include <iostream>#include "ourvector.h"using namespace std; ourvector<int> intersect(ourvector<int> &v1, ourvector<int> &v2) { // TO DO: write this function return {};}arrow_forwardThis is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees…arrow_forward
- >> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardC++ programming task. main.cc file: #include <iostream>#include <map>#include <vector> #include "plane.h" int main() { std::vector<double> weights{3.2, 4.7, 2.1, 5.5, 9.8, 7.4, 1.6, 9.3}; std::cout << "Printing out all the weights: " << std::endl; // ======= YOUR CODE HERE ======== // 1. Using an iterator, print out all the elements in // the weights vector on one line, separated by spaces. // Hint: see the README for the for loop syntax using // an iterator, .begin(), and .end() // ========================== std::cout << std::endl; std::map<std::string, std::string> abbrevs{{"AL", "Alabama"}, {"CA", "California"}, {"GA", "Georgia"}, {"TX", "Texas"}}; std::map<std::string, double> populations{ {"CA", 39.2}, {"GA", 10.8}, {"AL", 5.1}, {"TX", 29.5}}; std::cout <<…arrow_forwardC++ This program does not compile! Spot the error and give the line number. Presume that the header file is error free and // Implementation file for the Rectangle class.1. #include "Rectangle.h" // Needed for the Rectangle class2. #include <iostream> // Needed for cout3. #include <cstdlib> // Needed for the exit function4. using namespace std; //***********************************************************// setWidth sets the value of the member variable width. *//*********************************************************** 5. void Rectangle::setWidth(double w){6. if (w >= 0)7. width = w;8. else{9. cout << "Invalid width\n";10. exit(EXIT_FAILURE);}} //***********************************************************// setLength sets the value of the member variable length. *//*********************************************************** 11. void Rectangle::setLength(double len){12. if (len >= 0)13. length = len;14. else{15. cout << "Invalid length\n";16.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
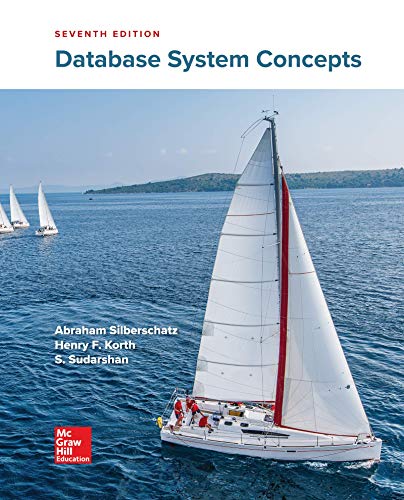
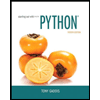
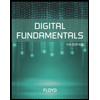
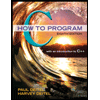
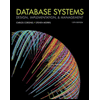
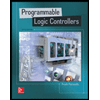