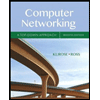
The function to be built, amino_acids, must return a list of a tuple and an integer when given a string of mRNA code. The first tuple must contain all the amino acids and the integer must be the number of distinct amino acids. You can use the dictionary below to help with your function. The function must also not include the stop codon codes.
NOTE : For this piece of code, we'll assume that there's only one stop codon in the sequence
{'CUU': 'Leu', 'UAG': '---', 'ACA': 'Thr', 'AAA': 'Lys', 'AUC': 'Ile', 'AAC': 'Asn','AUA': 'Ile', 'AGG': 'Arg', 'CCU': 'Pro', 'ACU': 'Thr', 'AGC': 'Ser','AAG': 'Lys', 'AGA': 'Arg', 'CAU': 'His', 'AAU': 'Asn', 'AUU': 'Ile','CUG': 'Leu', 'CUA': 'Leu', 'CUC': 'Leu', 'CAC': 'His', 'UGG': 'Trp','CAA': 'Gln', 'AGU': 'Ser', 'CCA': 'Pro', 'CCG': 'Pro', 'CCC': 'Pro', 'UAU': 'Tyr', 'GGU': 'Gly', 'UGU': 'Cys', 'CGA': 'Arg', 'CAG': 'Gln', 'UCU': 'Ser', 'GAU': 'Asp', 'CGG': 'Arg', 'UUU': 'Phe', 'UGC': 'Cys', 'GGG': 'Gly', 'UGA':'---', 'GGA': 'Gly', 'UAA': '---', 'ACG': 'Thr', 'UAC': 'Tyr', 'UUC': 'Phe', 'UCG': 'Ser', 'UUA': 'Leu', 'UUG': 'Leu', 'UCC': 'Ser', 'ACC': 'Thr', 'UCA': 'Ser', 'GCA': 'Ala', 'GUA': 'Val', 'GCC': 'Ala', 'GUC': 'Val', 'GGC':'Gly', 'GCG': 'Ala', 'GUG': 'Val', 'GAG': 'Glu', 'GUU': 'Val', 'GCU': 'Ala', 'GAC': 'Asp', 'CGU': 'Arg', 'GAA': 'Glu', 'AUG': 'Met', 'CGC': 'Arg'}
'---' represents the stop codons
Example would be :
'AUGUCGGCACAUUUAUGCUCCUAAUCC'
To give : [('Met', 'Ser', 'Ala', 'His', 'Leu', 'Cys', 'Ser'), 6]
This is the summary of what is required from the challenge:
- The output must be a list of a tuple of strings (amino acid) and a single integer (number of distinct amino acids)
- Must use the dictionary to call the amino acid or stop codon
- Must convert the three codes into an amino acid and stop at the stop codon (the function assumes all the codes have only one stop codon)
### START FUNCTION
def amino_acids(mrna):
### END FUNCTION
test : amino_acids('AUGUCGGCACAUUUAUGCUCCUAAUCC')
Expected Outputs
amino_acids('AUGUCGGCACAUUUAUGCUCCUAAUCC') == [('Met', 'Ser', 'Ala', 'His', 'Leu', 'Cys', 'Ser'), 6] amino_acids('AUGCCACCUUGA') == [('Met', 'Pro', 'Pro'), 2] amino_acids('AUGUCCCCUGCACGAGGGCCAUCCUGAUCCCCCCAU') == [('Met', 'Ser', 'Pro', 'Ala', 'Arg', 'Gly', 'Pro', 'Ser'), 6] amino_acids('AUGUGGCGACUCUAUCGCGUUGCCCGGUACUGAAUGCCACCU') == [('Met', 'Trp', 'Arg', 'Leu', 'Tyr', 'Arg', 'Val', 'Ala', 'Arg', 'Tyr'), 7]

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1-9). Store the jersey numbers and the ratings in a dictionary. Output the dictionary's elements with the jersey numbers in ascending order (i.e., output the roster from smallest to largest jersey number). Hint: Dictionary keys can be stored in a sorted list. (3 pts) Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Jersey number: 4, Rating: 5 Jersey number: 23, Rating: 4. Jersey number 30, Rating: 2 (2) Implement a menu of options for a user to modify the roster. Each option is…arrow_forwardPython code please help, indentation would be greatly appreciatedarrow_forwardNotice that all of the data in the inner lists are represented as strings. You are to write the function convert_data, which should make modifications to the inner lists according to the following rules: If and only if a string represents a whole number (ex: '3' or '3.0'), convert the string to an int. If and only if a string represents a number that is not a whole number (ex: '3.14'), convert the string to a float. Otherwise, leave the string as a str.HINT: The provided helper function is_number may be used (attached image). QUESTION: Complete the docstring below.def convert_data(data: List[list]) -> None: """Convert each string in data to an int if and only if it represents a whole number, and a float if and only if it represents a number that is not a whole number. >>> d = [['abc', '123', '45.6', 'car', 'Bike']] >>> convert_data(d) >>> d [['abc', 123, 45.6, 'car', 'Bike']] >>> d = [['ab2'], ['-123'], ['BIKES', '3.2'],…arrow_forward
- In python answer wuickarrow_forwardSearch closet 711 Complete the following function according to its docstring using a for loop. String method startswith may come in handy here. The lists we test will not all be shown; instead, some will be described in English. If you fail any test cases, you will need to read the description and make your own test. 1 from typing import List 2 3 4 5 7 8 9 10 11 12 13 14 15 16 17 18 19 20 def search_closet (items: List[str], colour: str) -> List[str]: """items is a list containing descriptions of the contents of a closet where every description has the form 'colour item', where each colour is one word and each item is one or more word. For example: ['grey summer jacket', 'orange spring jacket', 'red shoes', green hat'] colour is a colour that is being searched for in items. Return a list containing only the items that match the colour. >>>search_closet (['red summer jacket', 'orange spring jacket', 'red shoes', 'green hat'], 'red') ['red summer jacket', 'red shoes'] >>> search_closet (…arrow_forwardUse the function design recipe to develop a function named count_odds. The function takes a list of integers, which may be empty. The function returns the number of odd integers in the list. Automated testing is required Your solutions can use:• the [] operator to get and set list elements (e.g., lst[i] and lst[i] = a)• the in and not in operators (e.g., a in lst)• the list concatenation operator (e.g., lst1 + lst2)• the list replication operator (e.g., lst * n or n * lst)• Python's built-in len, min and max functions, unless otherwise noted.Your solutions cannot use:• list slicing (e.g., lst[i : j] or (lst[i : j] = t)• the del statement (e.g., del lst[0])• Python's built-in reversed and sorted functions.• any of the Python methods that provide list operations, e.g., sum, append, clear, copy, count, extend, index, insert, pop, remove, reverse and sort• list comprehensionsarrow_forward
- This program will store roster and rating information for a football team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers and the ratings in a dictionary. Output the dictionary's elements with the jersey numbers in ascending order (i.e., output the roster from smallest to largest jersey number). Hint: Dictionary keys can be stored in a sorted list. (3 pts)Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Jersey number: 4, Rating: 5 Jersey number: 23, Rating: 4 Jersey number 30, Rating: 2 ... (2) Implement a menu of options for a user to modify the roster. Each…arrow_forwardPyhton code please help, indentation would be greatly appreciatedarrow_forwardI am trying to write a function using python that receives a list and builds a dictionary containing the position in the list where every item last appeared.arrow_forward
- # Using the routes dictionary defining places your airline can go with #with mileage to each, create a function that determines the total #distance travelled by going to the airports in sequence defined by this # list itinerary and the routes dictionary. (No published answer... def summary (routesMap) : # Please do not change any code below... airports = list (routesMap.keys ()) for airport in airports: destinations = routesMap [airport] for n in range (0,len (destinations)): destinationInfo = destinations [n] destination = destinationInfo [0] mileage = destinationInfo [1] print (f"Leaving {airport} to {destination} for (mileage} miles.") def distanceUsing (routesMap, itinerary): milesTravelled = 0 ### Your code goes here. return milesTravelled def main (): # Please do not change this dictionary... routes = { main () "Austin": "Chicago": "Dallas": "Denver": "El Paso": "Houston": "Nashville": summary (routes) [["Chicago", 500], ["Houston", 180], ["Nashville", 86011, [["Austin", 500],…arrow_forwardCan you use Python programming language to to this question? Thanksarrow_forwardPython Complete the get_2_dimensional_list() function that takes two parameters: 1. An integer num_rows. 2. An integer num_columns. The function should return a 2D list with num_rows rows and num_columns columns. Each element is set to a random value between 1 and 99 included. If both parameters num_rows and num_columns are set 0, the function returns an empty list. You MUST use a for...in.range loop to create the 2D list. You do not need to import the random module as it has already been done for you. Some examples of the function being called are shown below. For example: Test random.seed (15) num_rows - 2 num columns 5 matrix = get_2_dimensional_list(num_rows, num_columns) print(matrix) random.seed (132) num_rows=2 num_columns 2 matrix = get_2_dimensional_list(num_rows, num_columns) print(matrix) Result [[27, 2, 67, 95, 5], [21, 31, 3, 8, 88]] [[53, 20], [53, 8]]arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
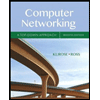
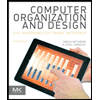
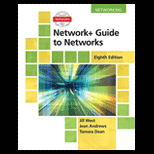
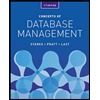
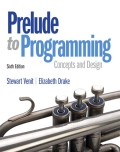
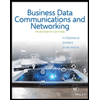