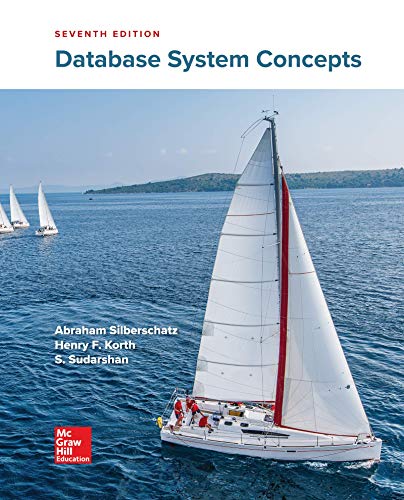
Can someone help me with C++? I have to implement a priorityQueue Linked List.
//////////////////////////////////////////
driver.cpp
//////////////////////////////////////////
#include <iostream>
#include <string>
#include "stackLL.h"
#include "queueLL.h"
#include "priorityQueueLL.h"
using namespace std;
int main()
{
//////////Test code for priority queue/////
priorityQueueLL<int> pQueue;
const int SIZE = 20;
//insert a bunch of random numbers
for (int i = 0; i < SIZE; i++)
{
pQueue.insert(rand());
}
//pull them back out..
//They must come out in order from smallest to largest
while (!pQueue.empty())
{
cout << pQueue.extractMin() << endl;
}
priorityQueueLL<string> pqs;
pqs.insert("whale");
pqs.insert("snake");
pqs.insert("buffalo");
pqs.insert("elmo");
pqs.insert("fire");
pqs.insert("waffle");
//buffalo elmo fire snake waffle whale
while (!pqs.empty())
{
cout << pqs.extractMin() << endl;
}
return 0;
}
//////////////////////////////////////////
priorityQueueLL.h
//////////////////////////////////////////

Answer:
I have done code and also I have attached code.
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- What is the implementation of each instruction located in the main function of the following code? This is a sample program, i am trying to teach myself linked list and am having trouble ------------------------------------------------------------------------------- #include <iostream>#include <string> /*** node* * A node class used to make a linked list structure*/ template<typename type>struct node {node() : data(), next(nullptr) {}node(const type & value, node * link = nullptr) : data(value), next(link) {}type data;node * next;}; /*** print_list* @param list - the linked-list to print out* * Free function that prints out a linked-list space separated.* Templated so works with any node.* Use this for testing your code.*/ template<typename type>void print_list(node<type> * list) { for(node<type> * current = list; current != nullptr; current = current->next) {std::cout << current->data << ' ';}std::cout << '\n';} /***…arrow_forwardCan you implement the StackADT.cpp, Stack.ADT , and StackMain.cpp files, please?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
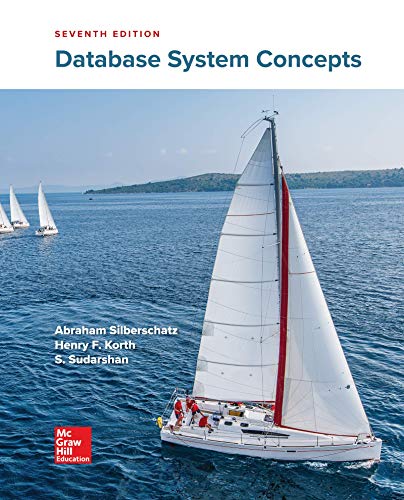
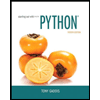
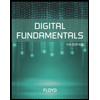
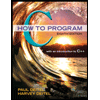
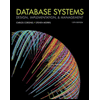
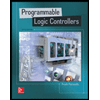