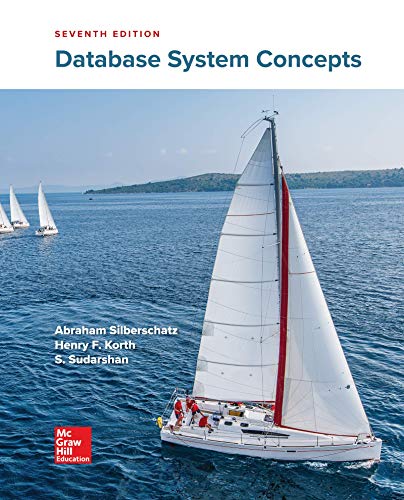
Certificate.java
public class Certificate {
private String holder;
private int level;
public void setHolderAndLevel(String newHolder, int newLevel) {
holder = newHolder;
level = newLevel;
}
public void print() {
System.out.println("Certificate: " + holder + ", Level: " + level);
}
}
-- SOLVE BELOW --
Certificates.java
import java.util.Scanner;
import java.util.ArrayList;
public class Certificates {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
ArrayList<Certificate> certificateList = new ArrayList<Certificate>();
Certificate currCertificate;
String currHolder;
int currLevel;
int certificateCount;
int i;
certificateCount = scnr.nextInt();
for (i = 0; i < certificateCount; ++i) {
currHolder = scnr.next();
currLevel = scnr.nextInt();
currCertificate = new Certificate();
currCertificate.setHolderAndLevel(currHolder, currLevel);
certificateList.add(currCertificate);
}
/* Your code goes here */
}
}
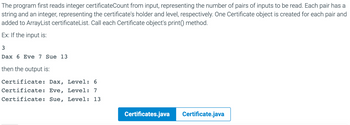

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- JAVA: Briefly describe (1-2 sentences) each of the following object-oriented programming concepts: Attributes Methods Primitive Data Types vs. Objects Static methods/variables vs. non-staticarrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardQuestion: Implement a simple expert system using the forward chaining inference method to diagnose medical conditions based on symptoms. The expert system should consist of a knowledge base containing rules and facts about medical conditions and symptoms. The system should be able to infer the possible medical conditions given a set of symptoms provided by the user. Requirements: 1. Define a set of medical conditions, symptoms, and rules in the knowledge base. 2. Implement the forward chaining inference method to infer medical conditions based on symptoms. 3. Allow users to input their symptoms and display the possible medical conditions.arrow_forward
- Java Programming I have a Java program with a restaurant/store menu. Can you please edit my program when displaying the physical receipt (I'm missing the menu items.) Task: When the user selects the items they would like to order on the physical receipt it should display the items as well. Can this be completed? My program is below: import java.util.Scanner; public class Restaurant2 { publicstaticvoidmain(String[] args) { // Define menu items and prices String[] menuItems= {"Apple", "Orange", "Pear", "Banana", "Kiwi", "Strawberry", "Grape", "Watermelon", "Cantaloupe", "Mango"}; double[] menuPrices= {1.99, 2.99, 3.99, 4.99, 5.99, 6.99, 7.99, 8.99, 9.99, 10.99}; StringusersName; // The user's name, as entered by the user. // Define scanner object Scannerinput=newScanner(System.in); // Welcome message System.out.println("Welcome to AppleStoreRecreation, what is your name:"); usersName = input.nextLine(); System.out.println("Hello, "+ usersName +", my name is Patrick nice to…arrow_forwardin java language... Draw the UML diagram of the following class: public class Employee { String name; double salary; public Employee() { } public double getSalary() { return this.salary; } public String getName() { return this.name; } }arrow_forwardClass field corruption must be prevented.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
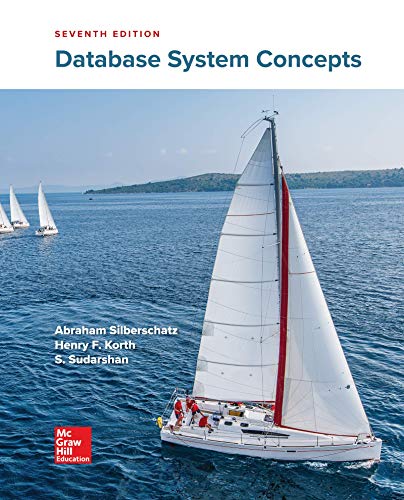
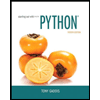
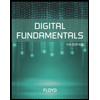
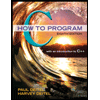
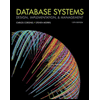
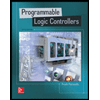