class WordPair{ public: WordPair (String first, String second) String getFirst() String getSecond() } class WordPairList { private: Vector allPairs public: WordPairList (String[] words) int numMatches () void display() } int main() { String wordNums [3] = {"one", "two", "three"}; WordPairList exampleOne = new WordPairList (wordNums, 3); exampleOne.display(); // print ("one", "two"), ("one", "three"), ("two", "three") String phrase [4] = {"the", "more", "the", "merrier"}; WordPairList exampleTwo = new WordPairList (phrase, 4); exampleTwo.display(); // print ("the", "more"), ("the", "the"), ("the", "merrier"), // ("more", "the"), ("more", "merrier"), ("the", "merrier") String moreWords [5] = {"the", "red", "fox", "the", "red"}; WordPairList exampleThree = new WordPairList (moreWords,5); cout<< exampleThree.numMatches () << endl; // print 2 exampleThree.display(); // print ("the", "red"), ("the", "fox"), ("the", "the"), // ("the", "red"), ("red", "fox"), ("red", "the"), // ("red", "red"), ("fox", "the"), ("fox", "red"), // ("the", "red") }
class WordPair{ public: WordPair (String first, String second) String getFirst() String getSecond() } class WordPairList { private: Vector allPairs public: WordPairList (String[] words) int numMatches () void display() } int main() { String wordNums [3] = {"one", "two", "three"}; WordPairList exampleOne = new WordPairList (wordNums, 3); exampleOne.display(); // print ("one", "two"), ("one", "three"), ("two", "three") String phrase [4] = {"the", "more", "the", "merrier"}; WordPairList exampleTwo = new WordPairList (phrase, 4); exampleTwo.display(); // print ("the", "more"), ("the", "the"), ("the", "merrier"), // ("more", "the"), ("more", "merrier"), ("the", "merrier") String moreWords [5] = {"the", "red", "fox", "the", "red"}; WordPairList exampleThree = new WordPairList (moreWords,5); cout<< exampleThree.numMatches () << endl; // print 2 exampleThree.display(); // print ("the", "red"), ("the", "fox"), ("the", "the"), // ("the", "red"), ("red", "fox"), ("red", "the"), // ("red", "red"), ("fox", "the"), ("fox", "red"), // ("the", "red") }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Help with c++... please paste indented code plzz and keep output same as given
![class WordPair{
public:
WordPair (String first, String second)
String getFirst()
String getSecond()
}
class WordPairList {
private:
Vector<WordPair> allPairs
public:
WordPairList (String[] words)
int numMatches ()
void display()
}
int main() {
String wordNums [3] = {"one", "two", "three"};
WordPairList exampleOne = new WordPairList (wordNums, 3);
exampleOne.display();
// print ("one", "two"), ("one", "three"), ("two", "three")
String phrase [4] = {"the", "more", "the", "merrier"};
WordPairList exampleTwo = new WordPairList (phrase, 4);
exampleTwo.display();
// print ("the", "more"), ("the", "the"), ("the", "merrier"),
// ("more", "the"), ("more", "merrier"), ("the", "merrier")
String moreWords [5] = {"the", "red", "fox", "the", "red"};
WordPairList exampleThree = new WordPairList (moreWords, 5);
cout<< exampleThree.numMatches () << endl; // print 2
exampleThree.display();
// print ("the", "red"), ("the", "fox"), ("the", "the"),
// ("the", "red"), ("red", "fox"), ("red", "the"),
// ("red", "red"), ("fox", "the"), ("fox", "red"),
// ("the", "red")
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3ed45464-784a-45df-bdff-9eb55f3d2668%2F17b2c953-0a61-4d64-88ac-1f1d4ea18864%2Fxq34ff5_processed.jpeg&w=3840&q=75)
Transcribed Image Text:class WordPair{
public:
WordPair (String first, String second)
String getFirst()
String getSecond()
}
class WordPairList {
private:
Vector<WordPair> allPairs
public:
WordPairList (String[] words)
int numMatches ()
void display()
}
int main() {
String wordNums [3] = {"one", "two", "three"};
WordPairList exampleOne = new WordPairList (wordNums, 3);
exampleOne.display();
// print ("one", "two"), ("one", "three"), ("two", "three")
String phrase [4] = {"the", "more", "the", "merrier"};
WordPairList exampleTwo = new WordPairList (phrase, 4);
exampleTwo.display();
// print ("the", "more"), ("the", "the"), ("the", "merrier"),
// ("more", "the"), ("more", "merrier"), ("the", "merrier")
String moreWords [5] = {"the", "red", "fox", "the", "red"};
WordPairList exampleThree = new WordPairList (moreWords, 5);
cout<< exampleThree.numMatches () << endl; // print 2
exampleThree.display();
// print ("the", "red"), ("the", "fox"), ("the", "the"),
// ("the", "red"), ("red", "fox"), ("red", "the"),
// ("red", "red"), ("fox", "the"), ("fox", "red"),
// ("the", "red")
}
![Q1: Write the WordPairList class. The constructor takes an array
of strings words as a parameter and initializes the instance
variable allPairs to an ArrayList of WordPair objects. A
WordPair object consists of a word from the array paired with a
word that appears later in the array.
The allPairs list contains WordPair objects (words[i], words [j])
for every i and j, where 0 < i < j < words.length. Each
WordPair object is added exactly once to the list. The following
examples illustrate two different WordPairList objects.
Example 1
String wordNums [3] = {"one", "two", "three"};
WordPairList exampleOne = new WordPairList (wordNums, 3);
After the code segment has executed, the allPairs instance variable of
exampleOne will contain the following WordPair objects in some order.
("one", "two"), ("one", "three"), ("two", "three")
Example 2
String phrase [4] = {"the", "more", "the", "merrier"};
WordPairList example Two = new WordPairList (phrase, 4);
After the code segment has executed, the allPairs instance variable of
example Two will contain the following WordPair objects in some order.
("the", "more"), ("the", "the"), ("the", "merrier"),
("more", "the"), ("more", "merrier"), ("the", "merrier")
Write the WordPairList method numMatches. This method returns the
number of WordPair objects in allPairs for which the two strings
match. For example, the following code segment creates a WordPairList
object.
String moreWords [5] = {"the", "red", "fox", "the", "red"};
WordPairList exampleThree = new WordPairList (moreWords, 5);
After the code segment has executed, the allPairs instance variable of
exampleThree will contain the following WordPair objects in some
order. The pairs in which the first string matches the second string
are shaded for illustration.
("the", "red"), ("the", "fox"), ("the", "the"),
("the", "red"), ("red", "fox"), ("red", "the"),
("red", "red"), ("fox", "the"), ("fox", "red"),
("the", "red")
The call exampleThree.numMatches () should return 2.
Class information for this question](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3ed45464-784a-45df-bdff-9eb55f3d2668%2F17b2c953-0a61-4d64-88ac-1f1d4ea18864%2F3jom0ri_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Q1: Write the WordPairList class. The constructor takes an array
of strings words as a parameter and initializes the instance
variable allPairs to an ArrayList of WordPair objects. A
WordPair object consists of a word from the array paired with a
word that appears later in the array.
The allPairs list contains WordPair objects (words[i], words [j])
for every i and j, where 0 < i < j < words.length. Each
WordPair object is added exactly once to the list. The following
examples illustrate two different WordPairList objects.
Example 1
String wordNums [3] = {"one", "two", "three"};
WordPairList exampleOne = new WordPairList (wordNums, 3);
After the code segment has executed, the allPairs instance variable of
exampleOne will contain the following WordPair objects in some order.
("one", "two"), ("one", "three"), ("two", "three")
Example 2
String phrase [4] = {"the", "more", "the", "merrier"};
WordPairList example Two = new WordPairList (phrase, 4);
After the code segment has executed, the allPairs instance variable of
example Two will contain the following WordPair objects in some order.
("the", "more"), ("the", "the"), ("the", "merrier"),
("more", "the"), ("more", "merrier"), ("the", "merrier")
Write the WordPairList method numMatches. This method returns the
number of WordPair objects in allPairs for which the two strings
match. For example, the following code segment creates a WordPairList
object.
String moreWords [5] = {"the", "red", "fox", "the", "red"};
WordPairList exampleThree = new WordPairList (moreWords, 5);
After the code segment has executed, the allPairs instance variable of
exampleThree will contain the following WordPair objects in some
order. The pairs in which the first string matches the second string
are shaded for illustration.
("the", "red"), ("the", "fox"), ("the", "the"),
("the", "red"), ("red", "fox"), ("red", "the"),
("red", "red"), ("fox", "the"), ("fox", "red"),
("the", "red")
The call exampleThree.numMatches () should return 2.
Class information for this question
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
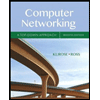
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
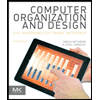
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
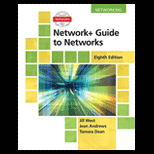
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
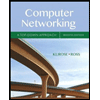
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
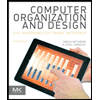
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
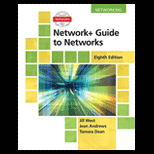
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
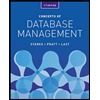
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
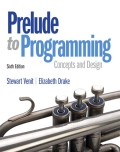
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
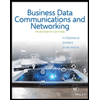
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY