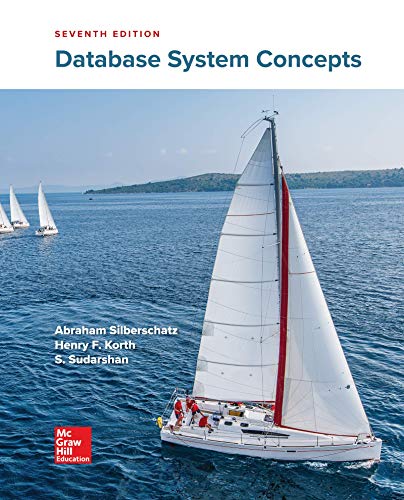
Im trying to get the program to out put this information
// ===== Code from file Person.java =====
public class Person {
private int ageYears;
private String lastName;
public void setName(String userName) {
lastName = userName;
}
public void setAge(int numYears) {
ageYears = numYears;
}
// Other parts omitted
public void printAll() {
System.out.print("Name: " + lastName);
System.out.print(", Age: " + ageYears);
}
}
// ===== end =====
// ===== Code from file Student.java =====
public class Student extends Person {
private int idNum;
public void setID(int studentId) {
idNum = studentId;
}
public int getID() {
return idNum;
}
}
// ===== end =====
// ===== Code from file StudentDerivationFromPerson.java =====
public class StudentDerivationFromPerson {
public static void main(String[] args) {
Student courseStudent = new Student();
/* Your solution goes here */
//Person a = new Person();
courseStudent.setName("Smith");
courseStudent.setAge(20);
courseStudent.setID(9999);
courseStudent.printAll();
System.out.print(",ID: "+ getID());
}
}
// ===== end =====

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Program - Python Make a class.The most important thing is to write a simple class, create TWO different instances, and then be able to do something. For example, create a pizza instance for Suzie, ask her for the toppings from the choices, and store her pizza order. Then create a second pizza instance for Larry, ask for his toppings and store his pizza order. Then print both of their orders back out. You do not need to make your class very complicated. (Shouldn't be the pizza example but something similar)arrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forwardAnalyze the following code. public class Test { public static void main(String[] args){ System.out.printlin( m(2) ); public static int m( int num ) { return num; } public static void m( int num ) { System.out.printin( num ); } The program has a compile error because the second m method is def method. The program runs and prints 2 once.arrow_forward
- 8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardFIX THE CODE PROVIDED BELOW Part 2 - Syntax Errors and Troubleshooting The code below has many syntax (and other) errors. Tasks:Compile the code and fix the errors one at a time. // Lab1a.java // This short class has several bugs for practice. // Authors: Carol Zander, Rob Nash, Clark Olson, you public class Lab1a { public static void main(String[] args) { compareNumbers(); calculateDist(); } publicstatic void compareNumbers() { int firstNum = 5; int secondNum; System.out.println( "Sum is: + firstNum + secondNum ); System.out.println( "Difference is: " + (firstNum - secondNum ); System.out.println( "Product is: " + firstNun * secondNum ); } public static void calculateDistance() { int velocity = 10; //miles-per-hour int time = 2, //hoursint distance = velocity * timeSystem.out.println( "Total distance is: " distanace); } } Part 3 - Print Statements and Simple Methods Use "print" and "println" statements, but use method calls to reduce the number of repeated "print"and "println"…arrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward
- Write a Fraction class that works with the FractionMath program above. It should use your Fraction.java file to run.Main Program:public class FractionMath{public static void main(String[] arguments){// create four fractions accountsFraction w = new Fraction();Fraction x = new Fraction(3,4);Fraction y = new Fraction(2,5);Fraction z = new Fraction(6,0);System.out.println("*** Does the toStringMethod work?"); System.out.println("First fraction : " + w); System.out.println("Second fraction : " + x); System.out.println("Third fraction : " + y); System.out.println("Forth fraction : " + z); System.out.println(); // Does the get() method work? System.out.println("*** Does the get() methods work?"); System.out.println("w = " + w.getNumerator() + " over " + w.getDenominator()); System.out.println();// Can we change the values correctly nameSystem.out.println("*** Does the set() methods work?");System.out.println("w fraction Before : " + w); w.setNumerator(22);System.out.println("w fraction After…arrow_forwardUsing the Python language answer the following questions below. Please tell me what program you use if it is IDLE or Atom or a python website please provide the website you use.arrow_forwardI have this code: import java.util.Scanner; public class CalcTax { public static void main(String[] args) { Scanner sc = new Scanner(System.in); // Welcome message System.out.println("Welcome to my tax calculation program."); // Declare the variables int i, pinCode, maxRetries = 3; String choice; // Get the PIN code for (i = 0; i < maxRetries; i++) { System.out.print("Please enter the PIN code: "); pinCode = sc.nextInt(); if (pinCode == 5678) { break; } System.out.println("Invalid pin code. Please try again."); } if (i == maxRetries) { System.out.println("Invalid pin code. You have reached the maximum number of retries."); System.exit(0); } // Get the income, interest, deduction, and paid tax amount double income, interest, deduction, paidTax; do { System.out.print("Please…arrow_forward
- In C++ class rectangleType { public: void setLengthWidth(double x, double y); //Sets the length = x; width = y; void print() const; //Output length and width double area(); //Calculate and return the area of the rectangle (length*width) double perimeter(); //Calculate and return the perimeter (length of outside boundary of the rectangle) private: double length; double width; }; Print out the area of the rectangle.arrow_forwardJava Questions - Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you. Part 1 - 6. Given the following code, the output is __. class Apple { int x = 15; }class Banana extends Apple { int x = 25; }public class Sample {public static void main(String[] args) {Apple a1 = new Banana();System.out.println(a1.x);}} A. Compiler errorB. 25C. 15D. 0E. None of the options Part 2 - 7. Which is possibly a composite class? class Seed { ... }class Fruit { Seed s1 = new Seed(); }class Apple extends Fruits { ... }class Orange extends Apple { ... }class ApplePie { ApplePie a1 = new ApplePie(); } A. FruitB. AppleC. ApplePieD. OrangeE. Seed Part 3 - 10. Given the following code, which statement is true? class Water() { ... }class Juice() extends Water { ... }class Apple() { ... }class ApplePie() { Apple a1 = new Apple(); }class AppleJuice() { Apple a1 = new Apple(); Water w1 = newWater(); } A. Juice is a superclass and Water is a…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
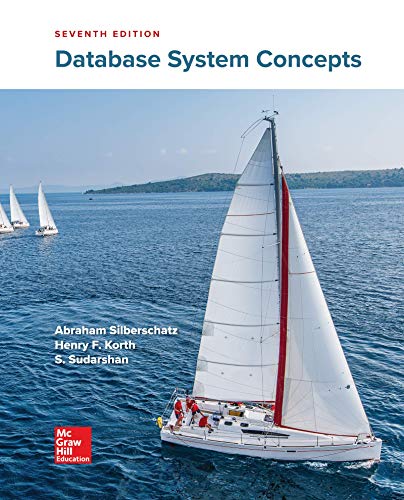
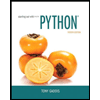
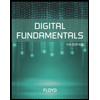
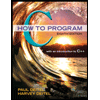
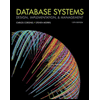
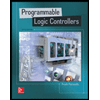