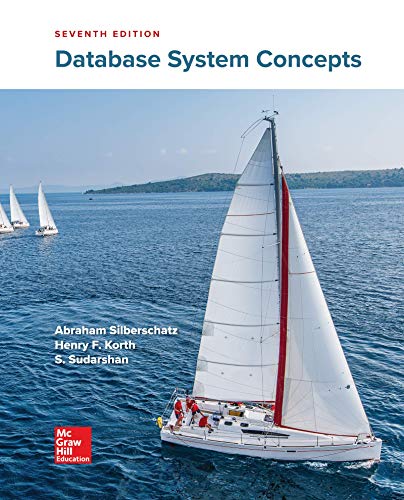
Concept explainers
CODE IN JAVA
design a program that grades arithmetic quizzes as follows: (Use the below startup code for Quizzes.java and provide code as indicated by the comments)
1. Ask the user how many questions are in the quiz.
2. Use a for loop to load the array. Ask the user to enter the key (that is, the correct answers). There should be one answer for each question in the quiz, and each answer should be an integer. They can be entered on a single line, e.g., 34, 7, 13, 100, 8 might be the key for a 5-question quiz. You will need to store the key in an array called "key".
3. Ask the user to enter the student's answers for the quiz to be graded. There needs to be one answer for each question. Note that each answer can simply be compared to the key as it is entered. If the answer is correct, add 1 to a correct answer counter. need this in a separate for loop from the loop used to load the array.
4. When the user has entered all of the answers to be graded, print the number correct and the percent correct.
5. When this works, nest your for loop and output statements in a do....while loop so that the user can grade any number of quizzes with a single key. After the results have been printed for each quiz, ask "Grade another quiz? (y/n)."
//******************************************************************
// Quizzes.java
// Grades multiple choice quizzes.
//******************************************************************
import java.util.Scanner;
import java.text.NumberFormat;
public class Quizzes
{
//----------------------------------------------
// Read in the number of questions followed by
// the key, then read in each student's answers
// and calculate the number and percent correct.
//----------------------------------------------
public static void main(String[] args)
{
int numQuestions;
int numCorrect;
String anotherQuiz;
int answer;
NumberFormat percent = NumberFormat.getPercentInstance();
Scanner scan = new Scanner (System.in);
System.out.println ("Quiz Grading");
System.out.println ();
System.out.print ("Enter the number of questions on the quiz: ");
numQuestions = scan.nextInt();
//CREATE THE ARRAY FOR THE KEY
System.out.print ("Enter the answer key: ");
//LOAD THE ARRAY FOR THE ANSWER KEY WITH INPUT FROM THE USER
//OUTER LOOP TO ALLOW THE USER TO ENTER GRADES
// FOR ANY NUMBER OF QUIZZES
System.out.print("Enter the student answers: ");
//INNER LOOP TO GET ANSWERS FROM THE USER AND COUNT THE NUMBER OF
// CORRECT ANSWERS
//DISPLAY THE NUMBER OF CORRECT ANSWERS AND PERCENT
//ASK USER IF THEY WISH TO GRADE ANOTHER QUIZ

Step by stepSolved in 2 steps

- Question Write Java code to do the following: Read from the keyboard an unknown number of values each of which is between 1 and 100. If a number entered is not between 1 and 100 (except for -1 which ends the input) print an error message. When a -1 is read in, stop the loop and print which of the numbers between 1 and 100 were not read in. (Hint: Use a boolean or counter array to keep track)arrow_forwardprogramming used: javaarrow_forwardTopic: Array covered in Chapter 7 Do not use any topic not covered in lecture. Write a C++ program to store and process an integer array. The program creates an array (numbers) of integers that can store up to 10 numbers. The program then asks the user to enter up to a maximum of 10 numbers, or enter 999 if there are less than 10 numbers. The program should store the numbers in the array. Then the program goes through the array to display all even (divisible by 2) numbers in the array that are entered by the user. Then the program goes through the array to display all odd (not divisible by 2) numbers in the array that are entered by the user. At the end, the program displays the total sum of all numbers that are entered by the user.arrow_forward
- I could really use some help with a problem I got for coding. A screenshot showing an example would help.arrow_forwardVariables Primitive Write a java program that asks the user to enter 3 integers. Add the integers and display “The total is “ the result Reference Write a java program that asks the user for their first name and last name. Display “Your full name is “ the first name and last name Arrays One-dimensional Write a java program that creates an array of integers called numbers and contains five elements. Then use a for loop to print them out using println(). Two-dimensional submit your code for the total of rows and total of columns herearrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forward
- PrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forwardc++ language using just one for looparrow_forwarduse C#programmingarrow_forward
- Exercise #1: Cumulative sum: Write a Java program that prompts the user to enter an integer number (n) that indicates the number of elements of an array (the maximum value for n is 100). Then the user enters n double numbers. The program creates another array of cumulative sums of the first array and outputs it. One decimal place should be used to output the cumulative array. Sample inputs/outputs: Enter the array size: 4 Enter the array elements: 5.0 6.5 7.3 10.2 Cumulative Array: 5.0 11.5 18.8 29.0 Enter the array size: 101 The maximum size is 100! Enter the array size: 5 Enter the array elements: 2.34 5.61 4.67 0.897 Cumulative Array: 2.3 8.0 12.6 19.6 20.4arrow_forwardHello, Stuck on this question. All programming is in Python. 2.) Design a program that generates a 7-digit lottery number. The program should have an INTEGER array with 7 elements. Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element. Then write another loop that displays the contents of the array. All programming is in Python.arrow_forwardCreate a Console application that does the following:This program must use a single array to store integers and find the maximum value.1. Create an array and initialize it internally with the values 5, 8, 9, 4, and 2.2. Use a loop to iterate through the array and determine the maximum value.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
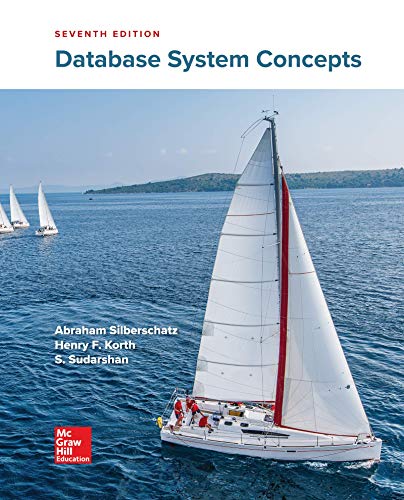
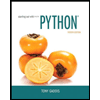
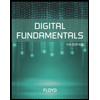
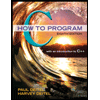
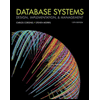
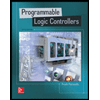