Use Java Implement a game of Pig where the user plays against a "hold at 20 or goal" computer player that rolls until a 1 ("pig") is rolled, or the turn total is greater than or equal to 20, or the score plus the turn total is greater than or equal to 100. The first player is chosen randomly. An empty input (i.e., Enter) indicates that the user wishes to roll. Any entered line of non-zero length indicates that the user wishes to hold. Before the game, randomly select which player the user will be, and print the line "You will be player #.", where # is the user's player number. Then, print an instruction line "Enter nothing to roll; enter anything to hold." Before each turn, print a line with "Player 1 score: " and player 1's score. Print another line with "Player 2 score: " and player 2's score. Finally, print a line with "It is player #'s turn.", where "#" is replaced by the current player number. Play starts with player 1 and then alternates. For each roll, print a line with "Roll: " and the random die roll value (1-6). For each non-"pig" roll 2-6 on the user's turn, prompt the user with "Turn total: ", the turn total, a tab, and "Roll/Hold? ". After a "pig" roll of 1, or a "hold", print a line with "Turn total: " followed by the turn total. In the case of a "pig", this turn total is 0. Then, print a line with "New score: " followed by the new score for the current player
Use Java
Implement a game of Pig where the user plays against a "hold at 20 or goal" computer player that rolls until a 1 ("pig") is rolled, or the turn total is greater than or equal to 20, or the score plus the turn total is greater than or equal to 100. The first player is chosen randomly.
An empty input (i.e., Enter) indicates that the user wishes to roll. Any entered line of non-zero length indicates that the user wishes to hold.
- Before the game, randomly select which player the user will be, and print the line "You will be player #.", where # is the user's player number. Then, print an instruction line "Enter nothing to roll; enter anything to hold."
- Before each turn, print a line with "Player 1 score: " and player 1's score. Print another line with "Player 2 score: " and player 2's score. Finally, print a line with "It is player #'s turn.", where "#" is replaced by the current player number. Play starts with player 1 and then alternates.
- For each roll, print a line with "Roll: " and the random die roll value (1-6).
- For each non-"pig" roll 2-6 on the user's turn, prompt the user with "Turn total: ", the turn total, a tab, and "Roll/Hold? ".
- After a "pig" roll of 1, or a "hold", print a line with "Turn total: " followed by the turn total. In the case of a "pig", this turn total is 0. Then, print a line with "New score: " followed by the new score for the current player
Sample Transcript (input underlined):
You will be player 2.
Enter nothing to roll; enter anything to hold.
Player 1 score: 0
Player 2 score: 0
It is player 1's turn.
Roll: 5 Roll: 3 Roll: 5 Roll: 1 Turn total: 0 New score: 0
Player 1 score: 0 Player 2 score: 0
It is player 2's turn.
Roll: 6
Turn total: 6
Roll/Hold? (Enter)
Roll: 5 Turn total: 11
Roll/Hold? (Enter)
Roll: 6
Turn total: 17
Roll/Hold? (Enter)
Roll: 2
Turn total: 19
Roll/Hold? (Enter)
Roll: 2
Turn total: 21
Roll/Hold? h
Turn total: 21
New score: 21
Player 1 score: 0
Player 2 score: 21
It is player 1's turn.
Roll: 5 Roll: 6 Roll: 3 Roll: 5 Roll: 1
Turn total: 0 New score: 0
Player 1 score: 0 Player 2 score: 21
It is player 2's turn.
Roll: 6 Turn total: 6
Roll/Hold? (Enter)
Roll: 6 Turn total: 12
Roll/Hold? (Enter)
Roll: 2
Turn total: 14
Roll/Hold? (Enter)
Roll: 6
Turn total: 20
Roll/Hold? h
Turn total: 20
New score: 41
Player 1 score: 0
Player 2 score: 41
It is player 1's turn.
Roll: 3 Roll: 3 Roll: 6 Roll: 4 Roll: 4 Turn total: 20
New score: 20
Player 1 score: 20 Player 2 score: 41 It is player 2's turn. Roll: 3 Turn total: 3 Roll/Hold? (Enter) Roll: 3 Turn total: 6 Roll/Hold? (Enter) Roll: 2 Turn total: 8 Roll/Hold? (Enter) Roll: 2 Turn total: 10 Roll/Hold? (Enter) Roll: 4 Turn total: 14 Roll/Hold? (Enter) Roll: 2 Turn total: 16 Roll/Hold? (Enter) Roll: 4 Turn total: 20 Roll/Hold? h Turn total: 20 New score: 61 Player 1 score: 20 Player 2 score: 61 It is player 1's turn. Roll: 5 Roll: 1 Turn total: 0 New score: 20 Player 1 score: 20 Player 2 score: 61 It is player 2's turn. Roll: 3 Turn total: 3 Roll/Hold? (Enter) Roll: 3 Turn total: 6 Roll/Hold? (Enter) Roll: 5 Turn total: 11 Roll/Hold? (Enter) Roll: 2 Turn total: 13 Roll/Hold? (Enter) Roll: 6 Turn total: 19 Roll/Hold? h Turn total: 19 New score: 80 Player 1 score: 20 Player 2 score: 80 It is player 1's turn. Roll: 3 Roll: 1 Turn total: 0 New score: 20 Player 1 score: 20 Player 2 score: 80 It is player 2's turn. Roll: 2 Turn total: 2 Roll/Hold? (Enter) Roll: 2 Turn total: 4 Roll/Hold? (Enter) Roll: 4 Turn total: 8 Roll/Hold? (Enter) Roll: 2 Turn total: 10 Roll/Hold? (Enter) Roll: 3 Turn total: 13 Roll/Hold? (Enter) Roll: 6 Turn total: 19 Roll/Hold? (Enter) Roll: 5 Turn total: 24 Roll/Hold? h Turn total: 24 New score: 104

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

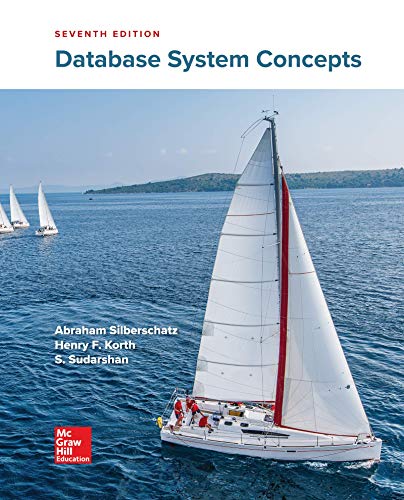
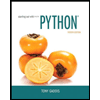
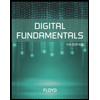
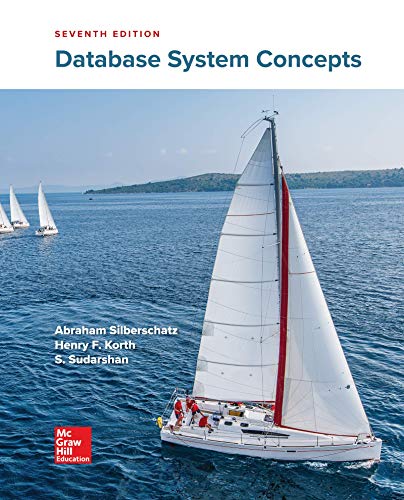
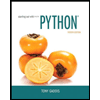
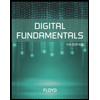
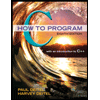
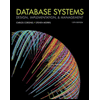
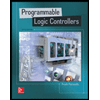