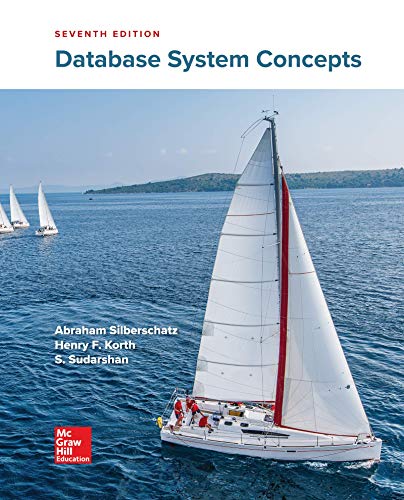
Concept explainers
Code the following in Python.
1.Assume each of the variables set1 and set2 references a set. Write code that creates another set containing the elements that are not shared by set1 and set2, and assigns the resulting set to the variable set3.
2.Assume the following list exists:
numbers = [1, 2, 3, 4, 5]
Write a statement that uses a dictionary comprehension to create a dictionary in which each element contains a number from the numbers list as its key, and the product of that number times 10 as its value. In other words, the dictionary should contain the following elements:
{1: 10, 2: 20, 3: 30, 4: 40, 5: 50}
3.Assume the following dictionary exists:
test_averages = {'Janelle':98, 'Sam': 87, 'Jennifer':92, 'Thomas':74, 'Sally':89, 'Zeb':84}
Write a statement that uses a dictionary comprehension to create a second dictionary named high_scores. The high_scores dictionary should contain all the elements of the test_averages dictionary in which the value is 90 or greater.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Code in pyhton.
2.Assume the following list exists:
numbers = [1, 2, 3, 4, 5]
Write a statement that uses a dictionary comprehension to create a dictionary in which each element contains a number from the numbers list as its key, and the product of that number times 10 as its value. In other words, the dictionary should contain the following elements:
{1: 10, 2: 20, 3: 30, 4: 40, 5: 50}
Code in pyhton.
2.Assume the following list exists:
numbers = [1, 2, 3, 4, 5]
Write a statement that uses a dictionary comprehension to create a dictionary in which each element contains a number from the numbers list as its key, and the product of that number times 10 as its value. In other words, the dictionary should contain the following elements:
{1: 10, 2: 20, 3: 30, 4: 40, 5: 50}
- This program will store roster and rating information for a football team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers and the ratings in a dictionary. Output the dictionary's elements with the jersey numbers in ascending order (i.e., output the roster from smallest to largest jersey number). Hint: Dictionary keys can be stored in a sorted list. (3 pts)Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Jersey number: 4, Rating: 5 Jersey number: 23, Rating: 4 Jersey number 30, Rating: 2 ... (2) Implement a menu of options for a user to modify the roster. Each…arrow_forwardpython pleasearrow_forwardWrite code that prints out the keys that have a value less than 3 in the following dictionary: d= {'a':5, 'is':3, 'this':2, 'program':1}arrow_forward
- This is question 5 on the same chapter 9arrow_forwardPlease in python, MUST use dictionaries This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers and the ratings in a dictionary. Output the dictionary's elements with the jersey numbers in ascending order (i.e., output the roster from smallest to largest jersey number). Hint: Dictionary keys can be stored in a sorted list. (3 pts)Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Jersey number: 4, Rating: 5 Jersey number: 23, Rating: 4 Jersey number 30, Rating: 2 ... (2) Implement a menu of options for…arrow_forwardCode the following directions in python. 1.Assume the variable dct references a dictionary. Write an if statement that determines whether the key 'Jim' exists in the dictionary. If so, delete 'Jim' and its associated value. 2.Write code to create a set with the following integers as members: 10, 20, 30, and 40. 3.Assume each of the variables set1 and set2 references a set. Write code that creates another set containing all the elements of set1 and set2, and assigns the resulting set to the variable set3.arrow_forward
- Implement the following: Given: person = {'name': 'bob'}1) Add the key/value pair 'emplStatus': 'Retired' to the dictionary person.2) Access the values in the dictionary person to print the following. Example OutputBob's employment status is Retired. NEED HELP WITH PYTHONarrow_forwardImplement the following: Given: names = ['ann', 'bill', 'calvin', 'david']ids = [12, 34, 56, 78] 1) Create a dictionary, stuInfo by using the two given lists above.2) Use a loop to print all keys and values in the dictionary stulfno. 3) Print the dictionary, stuInfo. Example Output12 ANN34 BILL 56 CALVIN78 DAVID{'ann': 12, 'bill': 34, 'calvin': 56, 'david': 78} NEED HELP WITH PYTHONarrow_forwardThis section has been set as optional by your instructor. OBJECTIVE: Write a program in Python that prints the middle value of a dictionary's values, using test_dict from the test_data.py module. NOTE: test_dict is created & imported from the module test_data.py into main.py Example 1: test dict = {'a':1,'b':9,'c':2,'d':5} prints 5 Example 2: test_dict = {'b':9,'d':5} prints 5 Hint: You may(or may not) find the following function useful: list.sort() which sorts the elements in a list. Note: the result is automatically stored in the original list, and return value from the function is None Туре list = [1, 2,3,2,1] After calling: list = [1,1,2,2,3] list.sort(), 339336.2266020 x3zgy7 LAB 13.11.1: Middlest Value (Structured Types) АCTIVITY 0/11 File is marked as read only Current file: test_data.py 1 #This module creates the test_dict to be used in main.py 2 #Reads input values from the user & places them into test_dict 3 dict_size = int(input()) #Stores the desired size of test_dict. 4…arrow_forward
- In Python languagearrow_forwardpython3 coding Problem 17 You create a dictionary and then turn that into a Pandas dataframe. Suppose you have three lists that contain the height in meter, the corresponding weight in kilogram, and the body mass index (bmi) of the height and weight as follow: height = [1.47, 1.5, 1.52, 1.55, 1.57, 1.6, 1.63, 1.65, 1.68, 1.7, 1.73, 1.75, 1.78, 1.8, 1.83] weight = [52.21, 53.12, 54.48, 55.84, 57.2, 58.57, 59.93, 61.29, 63.11, 64.47, 66.28, 68.1, 69.92, 72.19, 74.46] bmi = [24.16, 23.61, 23.58, 23.24, 23.21, 22.88, 22.56, 22.51, 22.36, 22.31, 22.15, 22.24, 22.07, 22.28, 22.23] Store the three lists in a pandas DataFrame. Call the DataFrame bmi_table. Call the columns height, weight, bmi. Show the first 6 rows of the table 2) You will use the dataframe you created in Problem 17 (more Pandas this shows me if you are good enough in basic Python At this point that you actually can tackle something new and not fall apart). Write a function get_sample_mean that takes in two input arguments:…arrow_forwardCode in pyhton. 2.Assume the following list exists: numbers = [1, 2, 3, 4, 5] Write a statement that uses a dictionary comprehension to create a dictionary in which each element contains a number from the numbers list as its key, and the product of that number times 10 as its value. In other words, the dictionary should contain the following elements: {1: 10, 2: 20, 3: 30, 4: 40, 5: 50}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
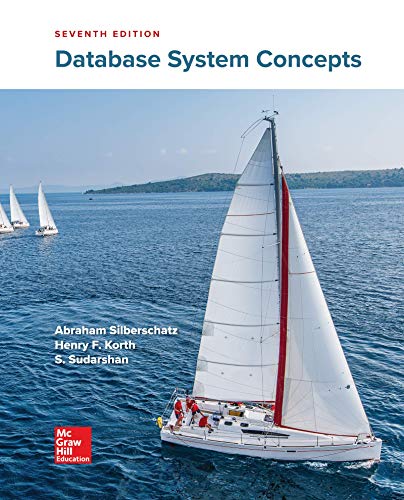
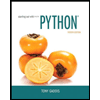
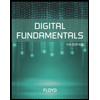
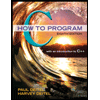
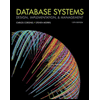
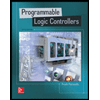