Compile the program classes.cpp, run it. Make sure that the program compiles and runs. Question 1. In the main function, add an object b of class B. Set the pointer ptr to point to b. Call increment() and decrement() on the object pointed to by ptr, print the object after each call. Which method gets called in each case? Why? Question 2. Add a protected variable int y to B and a constructor to initialize y to 0. Add a print() method in B so that both x and y are printed. Which print method is called on the object B referenced by ptr? If needed, change method declarations so that the print method of B is called for the B object. What did you need to change and why? Question 3. Assuming that a is a variable of type A, b is an object of type B, and ptr is the pointer of type A, what would you expect to be printed by the following statement: a = b; a.print(); ptr = &a; ptr -> print(); What gets printed? Why? Please explain the difference in behavior between this example and Question 2. Question 4. Write a function that takes an object of class A and prints it (don't forget to add the function prototype at the top of the file). Can you pass an object of class B to this function? Question 5. Write a function that takes an object of class A and returns it. Can you pass an object of class B to this function? Paste your modified file: classes.cpp and the answers to all questions as the comments in the file online in one document file. Include the questions, program outputs, and your answers in the comment section in the classes.cpp file for each question separately. No screenshots. I need to be able to copy and paste your code to run it. NOTE THAT YOU CAN SUBMIT THE ASSIGNMENT ONLY ONCE WHEN USING Turnitin.. See below the layout format (more consistency for grading): /* Question 1. In the main function, add an object b of class B. Set the pointer ptr to point to b. Call increment() and decrement() on the object pointed to by ptr, print the object after each call. Which method gets called in each case? Why? */ < Submit only the changed program code for question 1, in this case, the main()> /* Quest 2 ........ ....... */ and so on.
Save the following C++ program into a file classes.cpp:
#include <iostream>
using namespace std;
class A {
public:
A() { x = 0;}
void increment() { x++; }
virtual void decrement() { x--; }
void print() {cout << "x = " << x << endl;}
protected:
int x;
};
class B : public A {
public:
void increment() { x = x + 2; }
virtual void decrement() { x = x - 2; }
};
int main() {
A a; // a is an object of class A
A * ptr; // ptr is a pointer to an object of class A
ptr = &a;
ptr -> increment();
ptr -> print();
ptr -> decrement();
ptr -> print();
}
Compile the program classes.cpp, run it. Make sure that the program compiles and runs.
Question 1. In the main function, add an object b of class B. Set the pointer ptr to point to b. Call increment() and decrement() on the object pointed to by ptr, print the object after each call. Which method gets called in each case? Why?
Question 2. Add a protected variable int y to B and a constructor to initialize y to 0. Add a print() method in B so that both x and y are printed. Which print method is called on the object B referenced by ptr? If needed, change method declarations so that the print method of B is called for the B object. What did you need to change and why?
Question 3. Assuming that a is a variable of type A, b is an object of type B, and ptr is the pointer of type A, what would you expect to be printed by the following statement:
a = b;
a.print();
ptr = &a;
ptr -> print();
What gets printed? Why? Please explain the difference in behavior between this example and Question 2.
Question 4. Write a function that takes an object of class A and prints it (don't forget to add the function prototype at the top of the file). Can you pass an object of class B to this function?
Question 5. Write a function that takes an object of class A and returns it. Can you pass an object of class B to this function?
Paste your modified file: classes.cpp and the answers to all questions as the comments in the file online in one document file. Include the questions, program outputs, and your answers in the comment section in the classes.cpp file for each question separately. No screenshots. I need to be able to copy and paste your code to run it. NOTE THAT YOU CAN SUBMIT THE ASSIGNMENT ONLY ONCE WHEN USING Turnitin.. See below the layout format (more consistency for grading):
/*
Question 1. In the main function, add an object b of class B. Set the pointer ptr to point to b. Call increment() and decrement() on the object pointed to by ptr, print the object after each call. Which method gets called in each case? Why?
<program output>
<Your answers to the question>
*/
< Submit only the changed program code for question 1, in this case, the main()>
/*
Quest 2 ........
.......
*/
and so on.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

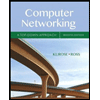
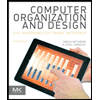
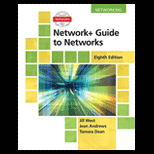
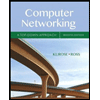
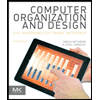
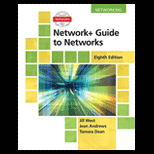
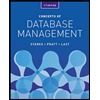
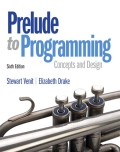
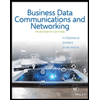