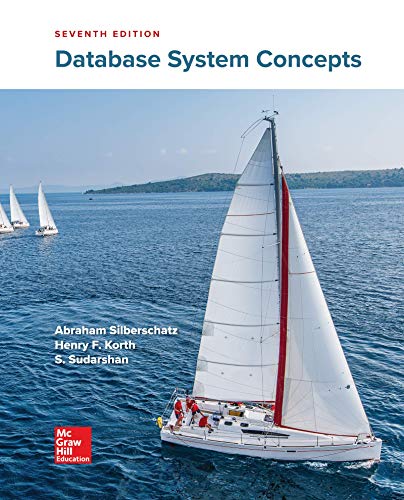
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
5. Construct a function C(V) that counts the number of basic operations for the following algorithm . Then place the algorithm in a time efficiency class. State any assumptions you make.
public static ArrayList topologicalSort(Graph dag) { Queue q = new LinkedList();
ArrayList sortOrder = new ArrayList();
int[] inEdgeCounts = new int[dag.size()];
for(int i = 0; i < inEdgeCounts.length; i++) { inEdgeCounts[i] = 0;
}
for(int i = 0; i < inEdgeCounts.length; i++) { dag.getAdjList(i).stream().forEach((x) -> inEdgeCounts[x]++);
}
// Identify sources
for(int i = 0; i < inEdgeCounts.length; i++) { if(inEdgeCounts[i] == 0) {
q.add(i);
}
}
while(!q.isEmpty()) { int currVertex = q.remove();
sortOrder.add(currVertex);
ArrayList adjList = dag.getAdjList(currVertex);
// Remove edges for this node for(Integer adjVertex: adjList) {
inEdgeCounts[adjVertex]--;
if(inEdgeCounts[adjVertex] == 0) {
q.add(adjVertex);
}
}
}
return sortOrder;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Complete the below function (using recursion)arrow_forwardNote: javaarrow_forwardImplement a simple linked list in Python (Write source code and show output) with basic linked list operations like: (a) create a sequence of nodes and construct a linear linked list. (b) insert a new node in the linked list. (b) delete a particular node in the linked list. (c) modify the linear linked list into a circular linked list. Use this template: class Node:def __init__(self, val=None):self.val = valself.next = Noneclass LinkedList:"""TODO: Remove the "pass" statements and implement each methodAdd any methods if necessaryDON'T use a builtin list to keep all your nodes."""def __init__(self):self.head = None # The head of your list, don't change its name. It shouldbe "None" when the list is empty.def append(self, num): # append num to the tail of the listpassdef insert(self, index, num): # insert num into the given indexpassdef delete(self, index): # remove the node at the given index and return the deleted value as an integerpassdef circularize(self): # Make your list circular.…arrow_forward
- Suppose a node of a linked list is defined as follows in your program: typedef struct{ int data; struct Node* link; } Node; Write the definition of a function, printOdd(), which displays all the odd numbers of a linked list. What will be the time complexity of printOdd()? Express the time complexity in terms of Big-O notation.arrow_forwardTrace insertion sort for list ={18,57,8,89,7}arrow_forwardQ3 QuickSort Given the QuickSort implementation from class, provide an 18-element list that will take the least number of recursive calls of QuickSort to complete. As a counter-example, here is a list that will cause QuickSort to make the MOST number of recursive calls: public static List input() { return Accaxsaslist(0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0); } wwwwwww And here's the QuickSort algorithm, for convenience: algorithm QuickSart Input: lists of integers 1st of size N Output: new list with the elements of lat in sorted order if N < 2 return st pivot Jat[N-1] left = new empty list right = new empty list for index = 0, 1, 2, if lst[i]<= pivot left.add(lat[i]) = else N-2 right add(lat[i]) return QuickSort(left) + [pivot] + QuickSort (right)arrow_forward
- Consider the implementation of the Ordered Linked list class, implement the following functions as part of the class definition: ▪ index(item) returns the position of item in the list. It needs the item and returns the index. Assume the item is in the list. ▪ pop() removes and returns the last item in the list. It needs nothing and returns an item. Assume the list has at least one item. ▪ pop_pos(pos) removes and returns the item at position pos. It needs the position and returns the item. Assume the item is in the list. ▪ a function that counts the number of times an item occurs in the linked list ▪ a function that would delete the replicate items in the linked list (i.e. leave one occurrence only of each item in the linked list) Your main function should do the following: ▪ Generate 15 random integer numbers in the range from 1 to 5.▪ Insert each number (Item in a node) in the appropriate position in a linked list, so you will have a sorted linked list in ascending order.▪…arrow_forwardConsidering a singly linked list of integers implementation, assuming that elements of the list are always in ascending order, write a function (find_median) that finds and returns median value. The function cannot scan the list more than ONCE. class SinglyList: class _Node: def __init__(self, e, next): self._element = e self._next = next def __init__(self): self._head = self._tail = None def find_median(self):arrow_forwardCode in Python:arrow_forward
- 5. Given an efficient circular bent array-based queue q capable of holding 7 objects. Show the final contents of the array after the following code is executed: for (int k = 1; k <= 6; k++) q.enqueue(k); for (int k = 1; k <= 3; k++) { q.dequeue(); q.enqueue(q.dequeue()); } 0 1 2 3 4 5 6arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 3. Define TestSLinkedList Classa. Declare an instance of the Single List class.b. Test all the methods of the Single List class.arrow_forwardPlease help with this Java program, and include explanations and commentsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
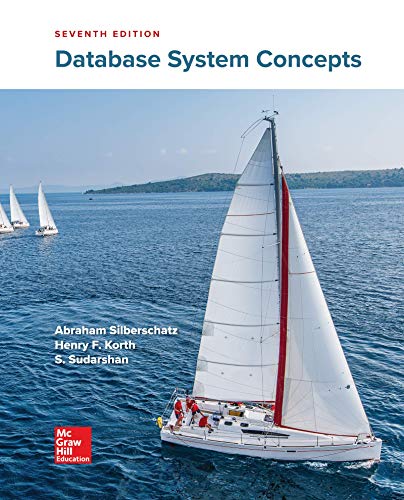
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
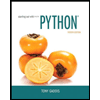
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
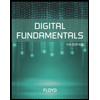
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
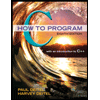
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
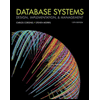
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
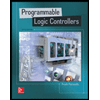
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education