CONVERT TO PYTHON LANGUAGE ONLY import java.util.InputMismatchException; import java.util.Scanner; public class CandyMachine { public static void main(String[] args) { System.out.println("*** Welcome to Shelly's Candy Shop ***"); // Creating dispenser objects: ProductDispenser Candy = new ProductDispenser(0.50, 12); ProductDispenser Chips = new ProductDispenser(0.65, 12); ProductDispenser Gum = new ProductDispenser(0.45, 12); ProductDispenser Cookies = new ProductDispenser(0.85, 12); // Creating cash register object: CashRegister CR = new CashRegister(); // For selection of item int select = 0; do { select = getIntInput("\n To select an item, enter \n" + "1 for Candy\n" + "2 for Chips\n" + "3 for Gum\n" + "4 for Cookies\n" + "9 to exit \n"); switch (select) { case 1: purchase(Candy, CR); break; case 2: purchase(Chips, CR); break; case 3: purchase(Gum, CR); break; case 4: purchase(Cookies, CR); break; case 9: break; default: System.out.println("Invalid Selection"); } } while (select != 9); } // Method to execute a purchase: public static void purchase(ProductDispenser product, CashRegister cashreg) { Scanner input = new Scanner(System.in); if (product.getProdQty() > 0) { double pmt = 0; // Collection of coin to purchase product. do { System.out.print("Please deposit " + (product.getProdCost() * 100 - pmt) + " cents."); pmt += input.nextDouble(); } while (pmt < product.getProdCost() * 100); product.setProdQty(1); cashreg.setCashOnHand(product.getProdCost()); System.out.println("Collect your item at the bottom and enjoy."); } } // Method for Input Selection public static int getIntInput(String prompt) { Scanner input = new Scanner(System.in); System.out.print(prompt); int intValue = 0; try { intValue = input.nextInt(); } catch (InputMismatchException e) { System.out.println("*** That is not a number. ***"); } return intValue; } } // CashRegister method class CashRegister { private double cashOnHand; CashRegister() { cashOnHand = 0; } double getCashOnHand() { return cashOnHand; } void setCashOnHand(double purchase) { cashOnHand += purchase; } } class ProductDispenser { private double prodCost; private int prodQty; ProductDispenser(double cost, int qty) { prodCost = cost; prodQty = qty; } double getProdCost() { return prodCost; } int getProdQty() { return prodQty; } void setProdQty(int qty) { prodQty -= qty; } }
CONVERT TO PYTHON LANGUAGE ONLY
import java.util.InputMismatchException;
import java.util.Scanner;
public class CandyMachine {
public static void main(String[] args) {
System.out.println("*** Welcome to Shelly's Candy Shop ***");
// Creating dispenser objects:
ProductDispenser Candy = new ProductDispenser(0.50, 12);
ProductDispenser Chips = new ProductDispenser(0.65, 12);
ProductDispenser Gum = new ProductDispenser(0.45, 12);
ProductDispenser Cookies = new ProductDispenser(0.85, 12);
// Creating cash register object:
CashRegister CR = new CashRegister();
// For selection of item
int select = 0;
do {
select = getIntInput("\n To select an item, enter \n" + "1 for Candy\n"
+ "2 for Chips\n" + "3 for Gum\n" + "4 for Cookies\n"
+ "9 to exit \n");
switch (select) {
case 1:
purchase(Candy, CR);
break;
case 2:
purchase(Chips, CR);
break;
case 3:
purchase(Gum, CR);
break;
case 4:
purchase(Cookies, CR);
break;
case 9:
break;
default:
System.out.println("Invalid Selection");
}
} while (select != 9);
}
// Method to execute a purchase:
public static void purchase(ProductDispenser product, CashRegister cashreg) {
Scanner input = new Scanner(System.in);
if (product.getProdQty() > 0) {
double pmt = 0;
// Collection of coin to purchase product.
do {
System.out.print("Please deposit "
+ (product.getProdCost() * 100 - pmt) + " cents.");
pmt += input.nextDouble();
} while (pmt < product.getProdCost() * 100);
product.setProdQty(1);
cashreg.setCashOnHand(product.getProdCost());
System.out.println("Collect your item at the bottom and enjoy.");
}
}
// Method for Input Selection
public static int getIntInput(String prompt) {
Scanner input = new Scanner(System.in);
System.out.print(prompt);
int intValue = 0;
try {
intValue = input.nextInt();
} catch (InputMismatchException e) {
System.out.println("*** That is not a number. ***");
}
return intValue;
}
}
// CashRegister method
class CashRegister {
private double cashOnHand;
CashRegister() {
cashOnHand = 0;
}
double getCashOnHand() {
return cashOnHand;
}
void setCashOnHand(double purchase) {
cashOnHand += purchase;
}
}
class ProductDispenser {
private double prodCost;
private int prodQty;
ProductDispenser(double cost, int qty) {
prodCost = cost;
prodQty = qty;
}
double getProdCost() {
return prodCost;
}
int getProdQty() {
return prodQty;
}
void setProdQty(int qty) {
prodQty -= qty;
}
}

Step by step
Solved in 2 steps with 4 images

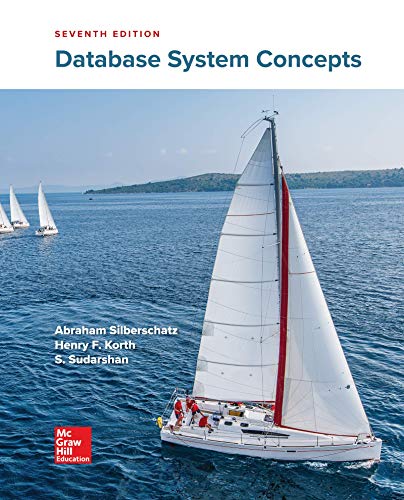
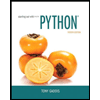
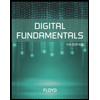
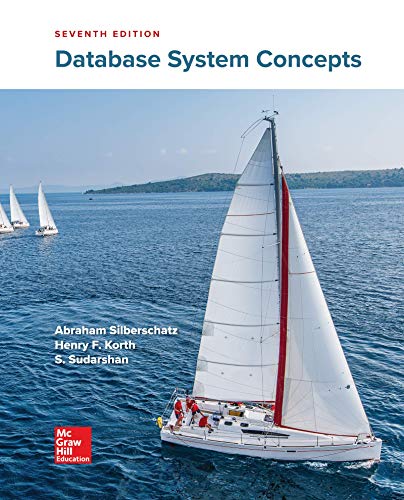
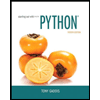
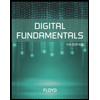
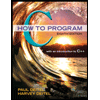
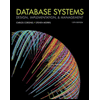
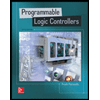