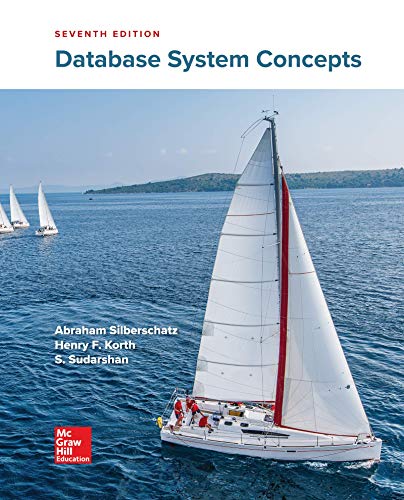
Concept explainers
modify the java
instead of aborting the program.
import javax.swing.*;
public class Main {
public static void main(String[] args) {
String len, wid;
Double dblLen, dblWid, area;
len = JOptionPane.showInputDialog("Enter a value for the length:");
wid = JOptionPane.showInputDialog("Enter a value for the width:");
dblLen=Double.parseDouble(len);
dblWid=Double.parseDouble(wid);
area=dblLen*dblWid;
JOptionPane.showMessageDialog(null,"The area is "+area);
}
}

Given code:
import javax.swing.*;
public class Main {
public static void main(String[] args) {
String len, wid;
Double dblLen, dblWid, area;
len = JOptionPane.showInputDialog("Enter a value for the length:");
wid = JOptionPane.showInputDialog("Enter a value for the width:");
dblLen=Double.parseDouble(len);
dblWid=Double.parseDouble(wid);
area=dblLen*dblWid;
JOptionPane.showMessageDialog(null,"The area is "+area);
}
}
Step by stepSolved in 4 steps with 7 images

- Given string inputStr on one line and integers idx1 and idx2 on a second line, output "Match found" if the character at index idx1 of inputStr is equal to the character at index idx2. Otherwise, output "Match not found". End with a newline. Ex: If the input is: eerie 4 1 then the output is: Match found Note: Assume the length of string inputStr is greater than or equal to both idx1 and idx2.arrow_forwardthis needs to be corrected The file provided in the code editor to the right contains syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors. java import java.util.Scanner; public class DebugThree3 { public static void main(String args[]) { String name; name = getName() displayGreeting(namme); } public static String getName(void) { String name; Scanner input = new Scanner(System.in); System.in.print("Enter name "); name = input.nexlLine(); return namer; } public static displayGreeting(String name) { System.outprintln("Hello, " + name + "!"); } }arrow_forwardimport java.util.Scanner; public class CircleAndSphereWhileLoop{ public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and…arrow_forward
- Java - How can I output the following statement as a single line with a newline? Program below. import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); if(n>=11 && n<=100) { while(n>=11 && n<=100) { System.out.print(n+ " "); (the problem line. When I use System.out.println, it makes the line vertical when I want it horizontal.) if(n ==11||n==22||n==33||n==44||n==55||n==66||n==77||n==88||n==99) break; n--; } } else System.out.println("Input must be 11-100"); }}arrow_forwardJava - How to make this a horizontal statement with a newline. Whenever I use System.out.println, it makes the output vertical instead of horizontal. What am I doing wrong? Program below. import java.util.Scanner; public class LabProgram { public static void main(String[] args) { int i; String InputString; Scanner sc = new Scanner(System.in); InputString = sc.nextLine(); char[] strArray = new char[InputString.length()]; for(i = 0; i < InputString.length(); i++){ strArray[i] = InputString.charAt(i); } for(i = 0 ; i< InputString.length() ; i++) if(strArray[i] >= 'a' && strArray[i] <= 'z' || strArray[i] >= 'A' && strArray[i] <= 'Z') System.out.print(strArray[i]); (the problem line) }}arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- How do I code: public static void rotateElements(int[] arr, int rotationCount) public static void reverseArray(int[] arr) In Java?arrow_forwardThe following java code segments causes infinite loop state where the case occurrence and update the code to solve it : int i; int r=2; for(i =1;i>0;i++) { System.out.println(i); ++r; }arrow_forwardJAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new…arrow_forward
- Convert the following java code to C++ //LabProgram.javaimport java.util.Scanner;public class LabProgram {public static void main(String[] args) {//defining a Scanner to read input from the userScanner input = new Scanner(System.in);//reading the value for Nint N = input.nextInt();//creating a 1xN matrixint[] m1 = new int[N];//creating an NxN matrixint[][] m2 = new int[N][N];//creating a 1xN matrix to store the resultint[] result = new int[N];//looping and reading N integers into m1for (int i = 0; i < N; i++) {m1[i] = input.nextInt();}//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//reading an integer and storing it into m2 at position i,jm2[i][j] = input.nextInt();}}//multiply m1 and m2, store result in result//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//multiplying value at index j in m1 with value at j,i in m2, adding to current value at index i// on…arrow_forwardIdentify the errors in the following code segment * provide the correction for each error. Import Java.util.scanner; public class Readable { public Static void main(String[] args) { Scanner KB ; int shares; double averagePrice = 14.67; Shares = KB.nextDouble(); System.out.println("There were " , shares + " shares sold at $" + averagePrice + " per share."); } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
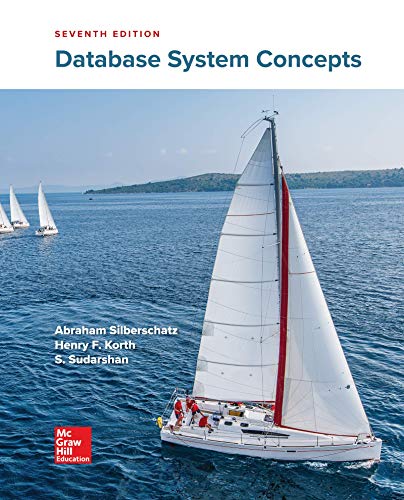
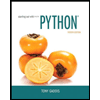
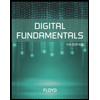
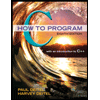
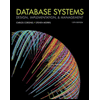
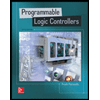