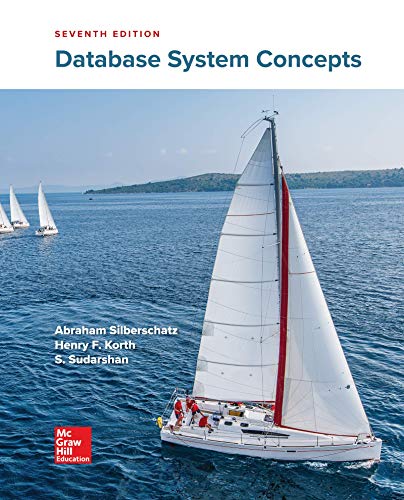
Create a Java Program using the scenario below.
You made a $6,000 purchase for a car using an approved Visa credit card. The annual interest rate for the card is eighteen percent. The minimum monthly payment rate is two percent. How much is the remaining balance after a year?
Equations for the program.
1: Minimum monthly payment (mmp) = mmp x balance
2: Interest paid = annual interest rate divided by 12 months times balance.
3: Principal paid = mmp minus interest paid(IP)
4: Remaining balance = balance minus principal paid.
Compute the first month of the MMP by taking two percent of the balance like below.
MMP = .02 times %6000 = $120
Then compute how much will apply to interest payment, and how much can pay for the principal amount. The annual interest rate divided by 12 to get monthly rate
IP = .18 divided bt 12.0 times $6000 = 90
Principal paid = 120 minus 90 = 30
Remaining balance will be principal paid minus the balance at the start of the month.(6000 minus 30 = 5970).
Finally, calculate the next eleven months by repeating the steps above. After twelve months(a year) show amount paid and outstanding balance. How many years will it take to pay off the debt.
Use java

Step by stepSolved in 2 steps with 2 images

- Writing a Modular Program in Java Summary In this lab, you add the input and output statements to a partially completed Java program. When completed, the user should be able to enter a year, a month, and a day to determine if the date is valid. Valid years are those that are greater than 0, valid months include the values 1 through 12, and valid days include the values 1 through 31. Instructions Notice that variables have been declared for you. Write the simulated housekeeping() method that contains input statements to retrieve a year, a month, and a day from the user. Add statements to the simulated housekeeping()method that convert the String representation of the year, month, and day to ints. Include the output statements in the simulated endOfJob()method. The format of the output is as follows:month/day/year is a valid date. or month/day/year is an invalid date. Execute the program entering the following date: month = 5, day = 32, year =2014Observe the output of this…arrow_forwardWrite a Java program to calculate a gamer's total XP score with a bonus per level. The program should: 1. Prompt and read the user’s input for the gamer's name, Level 1 XP (L1), Level 2 XP (L2), Level 3 XP (L3), and Engagement score (ES). User Scanner to read input. 2. Each XP score input should be in whole numbers between 10-100 and in increments of 5. The total XP score with bonuses should be calculated as follows: L1+L1*0.20+L2+L2*0.30+L3+L3*0.50+ES+ES*0.60 3. Output the gamer's information and the total calculated XP score (including bonuses). 4. Prompt the user as to whether they want to calculate total XP for another gamer and repeat the input/output processing. 5. Allow the user to exit the program without inputting the gamer's data Documentation o Include header comments that include your name, date, and description of the program. o Include body comments. o Consistent indentations o Consistent white line spaces.arrow_forwardWrite a Java program that can "make change." Your program should take two numbers as input, one that is a monetary amount charged and the other that is a monetary amount given. It should then return the number of each kind of bill and coin to give back as change for the difference between the amount given and the amount charged. The values assigned to the bills and coins can be based on the monetary system of any current or former government. Try to design your program so that it returns the fewest number of bills and coins as possible.arrow_forward
- Write a program in java that completes the following prompt: You are a fundraising distributor who needs to to pre-sell a limited number of doughnut coupon books. Each buyer can buy as many as 4 coupon books. No more than 100 coupon books can be sold. Implement a program called Fundraiser that prompts the user for the desired number of coupon books and then displays the number of remaining coupon books. Repeat until all coupon books have been sold, and then display the total number of buyers.arrow_forwardWrite a Java program that allows user to play Rock, Paper and Scissors game with a computer based on the exercise we did in class (which is posted on Moodle). You can refer to Powerpoint Slide #46 to use integers 0, 1, and 2 to represent rock, paper and scissors. At the beginning your program displays a welcome message "Welcome to Rock, Paper and Scissors game with my Computer", it then asks the user to enter his/her choice (only one of the choices: rock, paper and scissors is allowed). You can use a do... while loop to check the validity of the user input. Then your program use a random number generator to pick rock, or paper or scissors for the computer and displays computer’s pick. After that your program displays the result (either you win, or lose or a draw). After one game, your program asks user if he/she wants to continue. If the user decides to stop playing, your program displays how many games you played and how many time you win, lose and tie, and displays a "Thank you for…arrow_forwardI have been working on writing a java program (that goes with another I just got finished- see below CollegeCourse & Student) that prompts user to put in letter grades (A-F) for 5 different courses for a total of 10 different students. So I need to prompt user to input a student ID, then the 1st course ID, then the grade, then the 2nd course ID- then grade, the the 3rd course ID then grade, then the 4th course ID then grade, then finally the 5th course ID then grade. Then it needs to go through the same thing 10 times (for a total of 10 students). It also says the user is asked to "Enter ID for student #s" where s is an integer from 1 through 10, indicating the student (and I don't really know how to do that but I tried as I googled java); AND "Enter course ID #n, where n is an integer from 1 through 5, indicating the course number. And last it needs to verify for grade entry that only the A, B, C, D, or F are entered.I started writing notes to try to keep track of where thing are…arrow_forward
- Create a Java program that can be used to calculate the average weekly and monthly grocery bill for a family of four: Prompt the user for the coupon amount as a decimal (example, .10). Ensure the value is set to 10% if the value exceeds 100% or is less than or equal to zero. Prompt the user for 2-4 weeks of grocery bills. Calculate the monthly and weekly average for groceries. Display monthly total and weekly average without the coupon. Display monthly total and weekly average with the coupon.arrow_forwardin a java program. Imagine that you own a flower store and you need to display a menu of all the item categories, items and prices you sell for your customers. Display the prices for each product and category. The flowers are {"Rose", "Carnation", "Tulips", "Sunflowers"}, the price in order are {1.15, 1.5, 2.5, 2.75} the second category is vase sizes, {"Small", "Medium", "Large", "XLarge"}, the prices in order are {1.25, 2.5, 3.75, 4.5}. The last category is add ons, {"Teddy Bear" , "note", "ballon", "choclates"}. The prices are in order {10.0, 2.5, 5.75, 8.75} The list of products and the list of prices should be stores in 2 separate arrays. Create a method "display" that accepts 2 arrays as parameters and displays the product and the prices in receipt form. Create another method "sum" that displays the total for each category.arrow_forwardsolve all the program in java 1. Write a Java program to print 'Hello' on screen and then print your name on a separateline.Expected Output:HelloDonald Trump2. A school has following rules for grading system:a. Below 25 - Fb. 25 to 45 - Ec. 45 to 50 - Dd. 50 to 60 - Ce. 60 to 80 - Bf. Above 80 - AAsk user to enter marks and print the corresponding grade.3. Create a function that takes two numbers as arguments and returns the GCD of the twonumbers.Examplesgcd(3, 5) ➞ 1gcd(14, 28) ➞ 14gcd(4, 18) ➞ 24. Given an integer, create a function that returns the next prime. If the number is prime,return the number itself.ExamplesnextPrime(12) ➞ 13nextPrime(24) ➞ 29nextPrime(11) ➞ 11// 11 is a prime, so we return the number itself.5. Write a Java program that takes two numbers as input and display the product of twonumbers.Test Data:Input first number: 25Input second number: 5Expected Output:25 x 5 = 125 6. Write a Java program to print the sum (addition), multiply, subtract, divide and…arrow_forward
- Design a Java program to take user information about t dollar bills. You will do calculations and print the result as below. Note that user can enter any number and your output user's input. Example: Enter nunber of one dollar bills: 769 769 dollar bills will be change to Hundred Fifty Twenty Ten Five Onearrow_forwardWrite a java application that prompts for the number of hours that an employee worked in a weekarrow_forwardWrite a FULL Java procedural program for one human player to play a “Higher or Lower” card game. In this game, each card has a value from 1..10 inclusive. There are 4 of each value in the deck, i.e., 40 cards in total. Cards are not replaced in the deck once drawn, i.e., no more than 4 of each value will be drawn. The program starts by asking the player for a target score. The game proceeds in a series of rounds with the program repeatedly drawing and showing a card from the deck to the player one at a time. Each time, it asks the player to enter "h" (higher) or "l" (lower) to guess whether the next card drawn will be higher or lower in value. The player gains a point if they guess correctly. The game continues until the player guesses incorrectly or the target score is reached. When the game ends, it prints either a "You win!" or a "Nice try, you scored …” message as illustrated below. The image provided shows two examples of the required program behaviour: (bold is keyboard input…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
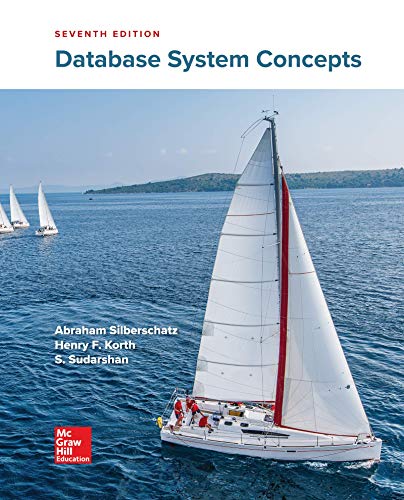
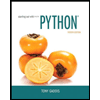
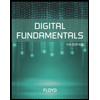
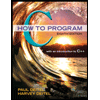
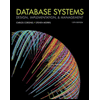
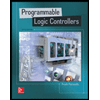