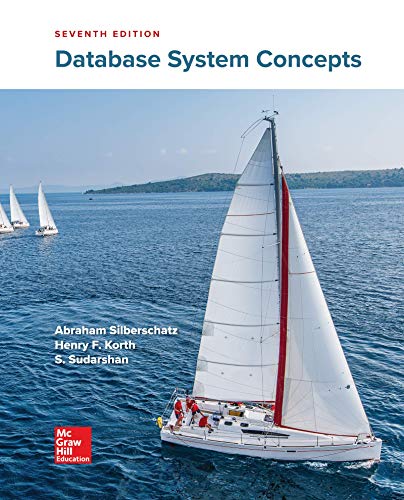
Create a new file (in Dev C++) and save it as lab12_XYZ.cpp (replace XYZ with your initials).
Create ANOTHER new file (in Dev C++) and save it as lab12_XYZ.h (replace XYZ with your initials).
Use the H (header) file to declare constants and define functions. Make sure to #include "lab12_XYZ.h", and place H file in same location as your main program.
Consider rolling two six-sided dice. While the result of each throw is independent of all rolls that have come before, we can still calculate the odds of rolling any given number between 2 and 12. This program will calculate those odds by generating a large number (100,000) of random values (i.e. "rolling the dice") and storing the results in an array. When all values have been rolled, we will display the results (counts and odds to 2 decimal places) to the user.
x x 2 3 4 5 6 7 8 9 10 11 12 //value for sum of 2 dice rolls+---+---+---+---+---+---+---+---+---+---+---+---+---+| | | | | | | | | | | | | | //counters for number of times value is rolled+---+---+---+---+---+---+---+---+---+---+---+---+---+
0 1 2 3 4 5 6 7 8 9 10 11 12 //array index
We need some constants
- int constants - NUM_ROLLS, NUM_SIDES, NUM_DICE, PRECISION, COLW1, COLW2, COLW3, etc.
- string constants - HELLO, GOODBYE, etc.
We need some functions:
- void displayMessage(string); //HELLO, GOODBYE, etc. (constants declared in your lab12_XYZ.h file...)
- int rollDice(); //returns a number between 2 and 12 (by rolling two dice and summing the result)
- void displayResults(int[]); //displays results in the array
In Lab 5, sample code was provided for generating a random number. Seed the random number generator in main(), then use rand() in the rollDice() function.
#include <cstdlib>#include <ctime>unsigned seed = time(0);srand(seed); //seed the RNGint num = (rand() % NUM_SIDES) + 1;
For this assignment:
- Header comments must be present in both CPP and H files
- Hello and goodbye messages must be shown
- Array(s) must be used for implementation
- DO NOT make arrays global variables
- Results should be formatted neatly (2 decimal places for odds, decimals lined up, etc.)
- Use comments and good style practices
HINT: Make sure to initialize counter array before starting loop
HINT: To calculate odds percentage: odds = count * 100 / NUM_ROLLS;
SAMPLE OUTPUT:Welcome! This program will calculate the odds of rolling 2 - 12 with two 6-sided dice.
Number of 2s rolled: 2776 ( 2.78%)
Number of 3s rolled: 5518 ( 5.52%)
Number of 4s rolled: 8234 ( 8.23%)
Number of 5s rolled: 11273 (11.27%)
Number of 6s rolled: 13884 (13.88%)
Number of 7s rolled: 16594 (16.59%)
Number of 8s rolled: 13848 (13.85%)
Number of 9s rolled: 11102 (11.10%)
Number of 10s rolled: 8394 ( 8.39%)
Number of 11s rolled: 5580 ( 5.58%)
Number of 12s rolled: 2797 ( 2.80%)
Exiting program. Goodbye!
Lanauge is C++
Please make it as simple as possible.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- You will be writing only the function definition. Write the function definition for AddBonus . The function will take two arguments, a double array called grades and an integer called numGrades. numGrades is the count of how many grades are in the array This function will have a void return type. Inside the AddBonus function: Declare a file pointer and a double variable and connect to a file called "input.txt" read one double value from the file add the double value that was read from the file to all the grades in the array Assume the file has the correct name and dataarrow_forwardIn pythonarrow_forwardWrite a function getQuestions that accepts a single parameter: filename, which is the name of a txt file. Your function should assume that the text file is formatted in the following way, as a series of questions and answers, with a question on one line, and the answer to the question on the following line, as below ---Sample File--- Why is the sky blue? Because air is blue. How long does it take light to reach Earth from the Sun? About eight minutes. What is the dumbest question you've ever been asked? That one. ---End Sample File--- Your function should create and return a dictionary where the keys are the questions and the values are respective answers (so the above sample file would result in a dictionary with three key-value pairs, one per question).arrow_forward
- FileAttributes.c 1. Create a new C source code file named FileAttributes.c preprocessor 2. Include the following C libraries a. stdio.h b. stdlib.h c. time.h d. string.h e. dirent.h f. sys/stat.h 3. Function prototype for function printAttributes() main() 4. Write the main function to do the following a. Return type int b. Empty parameter list c. Declare a variable of data type struct stat to store the attribute structure (i.e. statBuff) d. Declare a variable of data type int to store an error code (i.e. err) e. Declare a variable of data type struct dirent as a pointer (i.e. de) f. Declare a variable of data type DIR as a pointer set equal to function call opendir() passing explicit text “.” as an argument to indicate the current directory (i.e. dr) g. If the DIR variable is equal to NULL do the following i. Output to the…arrow_forwardWrite a C++ program to do the following: Read the attached file ("TextFile.txt") and the count the number of times each alphabetic letter is used. Do not count punctuation, whitespace, or numbers. Only count letters a-z. When counting, treat lower and upper case characters the same. Use the attached CPP program as a guide to manage the counting. Use the structure and array provided to maintain counts. See examples in the program. When the all processing is completed, display the total for all letter counts. Example Output Below.: This numbers below are not intended to be accurate. No special formatting required but should be readable. A: 103 B: 15 C: 22... // remaining lettersZ: 4 cpp code: #include "stdafx.h" #include <iostream> using namespace std; struct LetterCount { char letter; int count; }; // declare an array of structure const int MAXLETTERS = 26; LetterCount myLetterCount[MAXLETTERS]; int main() { // initialize - MOVE THIS TO A METHOD for…arrow_forwardI need help on my python assignment.arrow_forward
- Write a function in python named "read_words" that declares a parameter for a filename and returns a collection of unique words in the file. Even though words.txt contains a single word per line, you should not assume that this is true of every file. For example, if the line is a sentence, you should split the line into individual words before storing each word in your collection. Handle any errors that occur by printing a detailed error message. Hint: the Python set works like Java's HashSet.arrow_forwardi need help rewriting my code. the question and the errors are screenshotted. I spoke with a TA and they said that i dont need a loop and im supposed to use .urllib.request or whatever IN the function, not as a from statement like shown, Hence the rewrite part. Help would be immensely appreciated. i was told that this function should be pretty small. from urllib.request import urlopenimport json def json_loader(url): response = urllib.request json_data = json.loads(response.read()) for data, value in json_data.items():print(data , ":" , value)arrow_forwardWrite a Python function ‘AVGCSV’ that reads a .csv file that includes ‘n’ rows and ‘m’ columns, it calculates the average of each row and writes it at the end of the row, and it calculates the average of each column and writes it at the end of the column. The function should modify the .csv file and save it after adding all the averages.arrow_forward
- PYTHON cannot use numpy, opencv, array, readline( ), or enumerate. can only use open, read, write, and close methods for files. please utilize split and append functionsarrow_forwardConsider the data contained in the text file data1.txt. The first row of this file contains the column names. The remaining rows have one number for each column. The numbers are separated by a tab character. You can work in your command window. No need to create a matlab file for this question. Download the data file. Use importdata() function to load data into Matlab. What is the average of the numbers contained in the third column of this data? Data1: col1 col2 col3 col4 col5 906 552 743 348 45 676 229 425 661 558 469 642 430 384 773 913 485 125 628 312 105 152 25 22 179 746 782 291 911 339 737 101 318 801 211 562 295 654 746 511 185 238 957 814 907 598 531 936 384 629 300 92 458 618 102 135 406 241 576 391 213 105 764 531 55 895 113 760 276 502 72 785 741 249 432 243 292 744 452 998 54 604 106 228 812 442 965 682 805 486 14 433 464 987 895 898 695 213 30 138 197 759 99 536 391 94 433 824 88 928 308 656 176 803 918 457 110 164 990 714 102 934 666 67 619 996 188 895 940 344 333 267…arrow_forwardUSING PYTHON: Create a function that takes a filename("string") of a PGM image file as input and loads in the image in the file, and returns it as an image matrix. Rules: Cannot make any input calls in the main body, use basic python (nothing like .shape or .width) I have attached an example of what the function should do, as well as what the PGM image file looks like.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
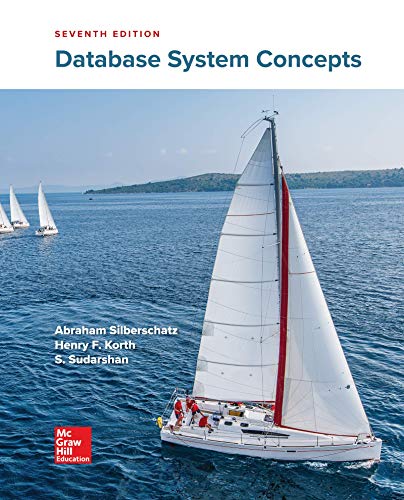
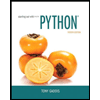
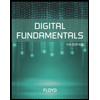
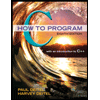
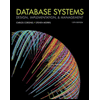
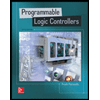