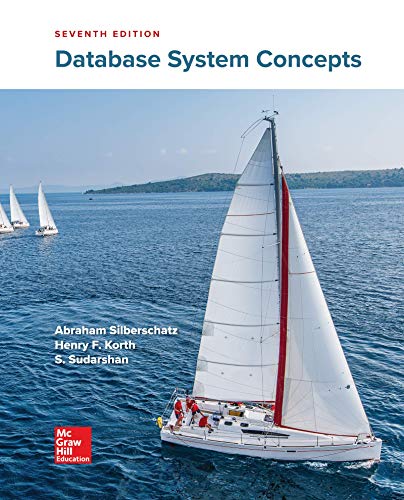
Concept explainers
Create a Person class for a user of the system. Create person.h and person.cpp files for this class.
The class should contain the following data of a person in the system:
• first name,
• last name,
• user ID, and
• email address.
These data should be accessible in its derived class. (Consider should the data be private, public,
or protected?)
This class should contain:
• a default constructor,
• a value constructor that receives all of the data to be placed into the data members as its
parameters,
• a copy constructor.
The class should also contain:
• “get” methods to allow the client to obtain information about a person.
o getFirstName, getLastName, getID, getEmail
• “set” methods to allow the client to assign information to a seller.
o setFirstName, setLastName, setID, setEmail, and optionally setAllInfo
• overloaded operators
o overloaded == method that returns true if two sellers have identical names and
email address; otherwise it returns false.
o overloaded !=, <, and > operators (for > and < operators, comparisons are based
on last names)
• An overloaded assignment = operator.
• Overloaded << and >> operators (consider should these be friend functions?)
• print() – a virtual function that displays on screen all the information of a person with
appropriate messages
• read() – a virtual function that reads in the information of a Person from an input file stream
Consider which of these methods should be const method?

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Which statement of the following is the most appropriate? Group of answer choices When you design a class, always make an explicit statement of the rules that dictate how the member variables are used. These rules are called the invariant of the class, and should be written at the botttom of the implementation file for easy reference. When you design a class, always make an explicit statement of the rules that dictate how the member variables are used. These rules are called the variant of the class, and should be written at the botttom of the implementation file for easy reference. When you design a class, always make an explicit statement of the rules that dictate how the member variables are used. These rules are called the invariant of the class, and should be written at the top of the implementation file for easy reference. When you design a class, always make an explicit statement of the rules that dictate how the member variables are used. These rules are called the…arrow_forwardProgram: Circle.h and newCircle.cpp 1: Create a class specification file, Circle.h, that contains the Circle class declaration 2: Create a class implementation file, Circle.cpp, that contains the member function definition, with a constructor that can accept arguments 3. Based on Program 13-8 in the textbook, create a program, newCircle.cpp, that generate two instances of the Circle class, pizza1 and pizza2, which can have different size (radius). Hint: Use the example source code in “Rectangle Version 4.zip” to create a multi-file project. Step 1: In Dev-C++, go to file à New à Project to create an empty project Setp 2: Go to ProjectàAdd to project, add new cpp files (e.g. Rectangle.cpp and Pr13-8.cpp files). Step 3: Compile and run Problem 2: Read the example source code in “HW2Problem2.zip” and modify it as follows: 1. Modify the code and create three rectangle objects, box1, box2, and box3. 2. Modify the code and create some static member function(s) that can count…arrow_forward// Declare data fields: a String named customerName, // an int named numItems, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of customerName to "no name"// and use zero for the other data fields.// Your code here... // Implement the overloaded constructor that// passes new values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method buyItem.//// Adds itemCost to the total cost and increments// (adds 1 to) the number of items in the cart.//// Parameter: a double itemCost indicating the cost of the item.public void buyItem(double itemCost){// Your code here... }// Implement method applyCoupon.//// Apply a coupon to the total cost of the cart.// - Normal coupon: the unit discount is subtracted ONCE// from the total cost.// - Bonus coupon: the unit discount is subtracted TWICE// from the total cost.// - HOWEVER, a bonus coupon only applies if the…arrow_forward
- Problem Statement: Develop an Inventory Management System (IMS) for a small retail business that allows the user to manage their product inventory. The system should enable the user to add, edit, update, and delete product information stored in a .csv file. The IMS should be console-based with a menu-driven interface. Requirements: 1. Classes and Objects: - Create a `Product` class with attributes such as `productID`, `productName`, `price`, `quantity`. - Implement a `InventoryManager` class that will handle operations like adding, editing, updating, and deleting products. - Use a `Main` class with the `main` method to run the program and display the menu. 2. File Operations: - Store product information in a .csv file named `inventory.csv`. - Implement methods in `InventoryManager` for reading and writing to the .csv file. 3. Menu-Driven Interface: - Implement a menu in the `Main` class that allows the user to select operations like Add, Edit, Update, Delete, and View Inventory. - Use…arrow_forwardDouble Bubble For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters. The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI. Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function…arrow_forwardCreate three files: Student.java - Abstract Class definition ENGRStudent.java - Derived Class definition Client.java - Contains main() method (1) Implement the abstract Student class with the following specifications: 3 private members String name int ID int yearAdmitted 1 constructors (public) A constructor with that accepts the student’s name, ID, and yearAdmitted as arguments. These values should be assigned to the object’s name, ID, and yearAdmitted fields. 2 public member methods A method named toString() that returns the values of name, ID, and yearAdmitted with String data type A abstract method name getRemainingHrs with int data type (2) Implement the derived class ENGRStudent class with the following specifications: 3 final private members with the following values : int MATHSCHRS=32 int MAJOR_HRS=66 int GenEdHrs=29 3 private members: int mathScHrs; int majorHrs; int GenEdHrs; 1 constructors (public) A constructor that accepts the student’s name, ID, and yearAdmitted…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
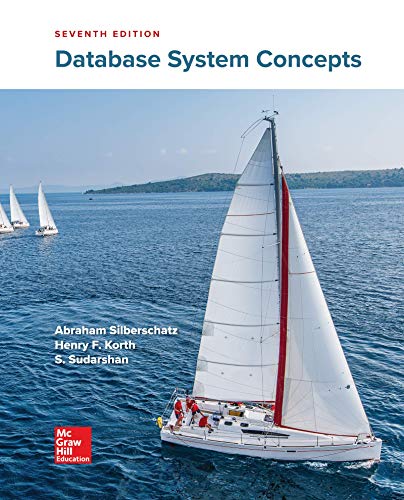
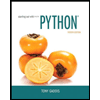
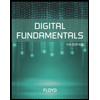
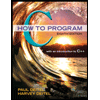
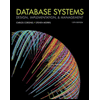
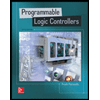