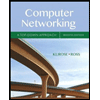
create a python program
Problem Statement
Implement all the functions of a dictionary (ADT) using hashing.
Data:
• Set of (key, value) pairs.
Keys are mapped to values.
Keys must be comparable.
Keys must be unique.
Standard Operations:
• Insert(key, value)
• Find(key),
• Delete(key)
This is the example output of the program.
====== CPE 204L Dictionary =====
[1] : Insert Word
[2] : Find Word
[3] : Delete Word
[4] : Exit
============================
Enter your choice : 1
Enter word: pretty
Enter meaning: beautiful
Pretty is successfully inserted into dictionary
====== CPE 204L Dictionary =====
[1] : Insert Word
[2] : Find Word
[3] : Delete Word
[4] : Exit
============================
Enter your choice : 1
Enter word: pretty
Enter meaning: beautiful
Duplicate Word! Pretty is not successfully inserted into dictionary.
====== CPE 204L Dictionary =====
[1] : Insert Word
[2] : Find Word
[3] : Delete Word
[4] : Exit
============================
Enter your choice : 2
Enter word: pretty
Word is Present in Dictionary
pretty = beautiful
====== CPE 204L Dictionary =====
[1] : Insert Word
[2] : Find Word
[3] : Delete Word
[4] : Exit
============================
Enter your choice : 3
Enter word: pretty
Word is successfully deleted
====== CPE 204L Dictionary =====
[1] : Insert Word
[2] : Find Word
[3] : Delete Word
[4] : Exit
============================
Enter your choice : 2
Enter word: pretty
Word not Found.
====== CPE 204L Dictionary =====
[1] : Insert Word
[2] : Find Word
[3] : Delete Word
[4] : Exit
============================
Enter your choice : 4
Thank you for using the dictionary program

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- This section has been set as optional by your instructor. OBJECTIVE: Write a program in Python that prints the middle value of a dictionary's values, using test_dict from the test_data.py module. NOTE: test_dict is created & imported from the module test_data.py into main.py Example 1: test dict = {'a':1,'b':9,'c':2,'d':5} prints 5 Example 2: test_dict = {'b':9,'d':5} prints 5 Hint: You may(or may not) find the following function useful: list.sort() which sorts the elements in a list. Note: the result is automatically stored in the original list, and return value from the function is None Туре list = [1, 2,3,2,1] After calling: list = [1,1,2,2,3] list.sort(), 339336.2266020 x3zgy7 LAB 13.11.1: Middlest Value (Structured Types) АCTIVITY 0/11 File is marked as read only Current file: test_data.py 1 #This module creates the test_dict to be used in main.py 2 #Reads input values from the user & places them into test_dict 3 dict_size = int(input()) #Stores the desired size of test_dict. 4…arrow_forwardMust show it in Python: Please show step by step with comments. Please show it in simplest form. Input and Output must match with the Question Please go through the Question very carefully.arrow_forwardIn python: student_dict is a dictionary with students' name and pedometer reading pairs. A new student is read from input and added into student_dict. For each student in student_dict, output the student's name, followed by "'s pedometer reading: ", and the student's pedometer reading. Then, assign average_value with the average of all the pedometer readings in student_dict..arrow_forward
- Python, input file has the following structure (number, then animal each on a line): 4Cows5Camels1Peacock3Whales4Lions2Scorpions Two output files are required:first output_file: sort by (dictionary) key but those sharing the same key should be listed on the same line with a ";" separated 1: Peacock2: Scorpions3: Whales4: Cows; lions ------------------------------> separated by ';' because they share the same key 45: Camels second output_file:Only values are sorted as follows and written to file: CamelsCowsLions PeacockScorpionsWhales Thank youarrow_forwardin PythonCreate a dictionary from these points: • The variables is “dictionary1”• Create 4 keys called: D1,D2,D3,D4• The values will be for D1 a tuple with three elements, D2 and D4 a different list with 5 elements each and D3 will be a string Print the dictionary to verify that it was created correctly, if not, repeat the steps until it is created and test access to key valuesarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
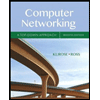
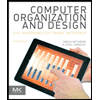
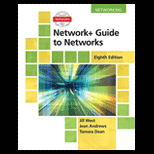
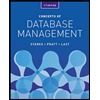
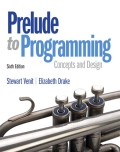
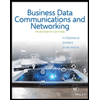