Create a UML diagram to help design the class baby. class Baby{ //data members private String name; private int age; //default constructor Baby() { name = "xyz"; age = 3; } //parameterized constructor Baby(String n,int a){ name = n; age = a; } //sets name public void setname(String n){ if(n.isEmpty()) name = "xyz"; else name = n; } //sets age public void setage(int a){ if(!(a>=1 && a<=4)) age = 2; else age = a; } //returns name public String getname(){ return name; } //returns age public int getage(){ return age; } //check if two objects have same name and age public boolean equals(Baby b){ if (age==b.getage() && name.equalsIgnoreCase(b.getname())) return true; else return false; } } public class Main { public static void main(String[] args) { //two objects of type Baby Baby A=new Baby("abcd",3); Baby B= new Baby("abcd",3); //print information of object System.out.println("Name:" + A.getname() + " age:"+ A.getage()); //call equals on obejct A and pass object B to it System.out.println("The two babies are identical? " + A.equals(B)); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create a UML diagram to help design the class baby.
class Baby{
//data members
private String name;
private int age;
//default constructor
Baby()
{
name = "xyz";
age = 3;
}
//parameterized constructor
Baby(String n,int a){
name = n;
age = a;
}
//sets name
public void setname(String n){
if(n.isEmpty())
name = "xyz";
else
name = n;
}
//sets age
public void setage(int a){
if(!(a>=1 && a<=4))
age = 2;
else
age = a;
}
//returns name
public String getname(){
return name;
}
//returns age
public int getage(){
return age;
}
//check if two objects have same name and age
public boolean equals(Baby b){
if (age==b.getage() && name.equalsIgnoreCase(b.getname()))
return true;
else
return false;
}
}
public class Main
{
public static void main(String[] args) {
//two objects of type Baby
Baby A=new Baby("abcd",3);
Baby B= new Baby("abcd",3);
//print information of object
System.out.println("Name:" + A.getname() + " age:"+ A.getage());
//call equals on obejct A and pass object B to it
System.out.println("The two babies are identical? " + A.equals(B));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

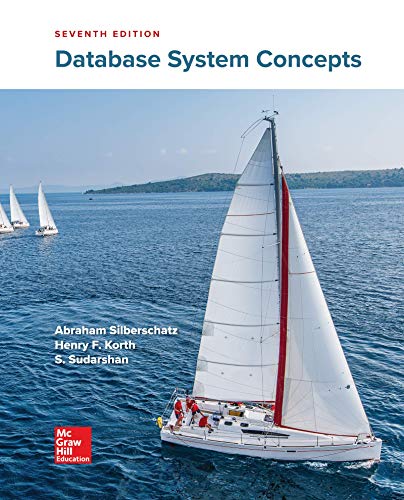
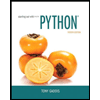
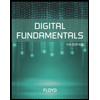
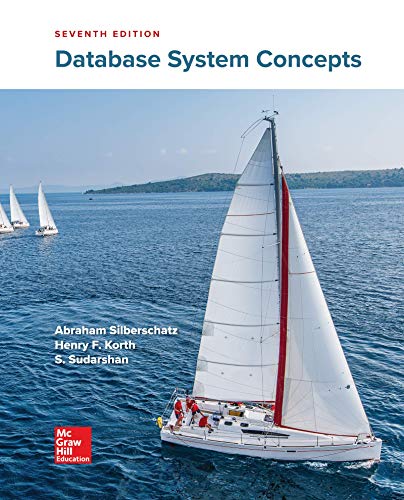
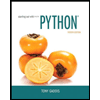
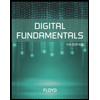
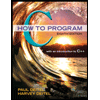
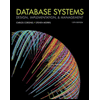
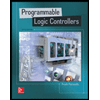