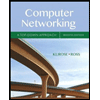
Java Program
This assignment requires one project with two classes.
Class Employee
-
Class Employee- I will attach the code for this:
//Import the required packages.
import java.text.DecimalFormat;
import java.text.NumberFormat;
//Define the employee class.
class Employee {
//Define the data members.
private String id, lastName, firstName;
private int salary;
//Create the constructor.
public Employee(String id, String lastName,
String firstName, int salary) {
this.id = id;
this.lastName = lastName;
this.firstName = firstName;
this.salary = salary;
}
//Define the getter methods.
public String getId() {
return id;
}
public String getLastName() {
return lastName;
}
public String getFirstName() {
return firstName;
}
public int getSalary() {
return salary;
}
//Define the method to return the employee details.
@Override
public String toString() {
//Use number format and decimal format
//to format the salary to desired output.
NumberFormat nfm = NumberFormat.getCurrencyInstance();
String pt = ((DecimalFormat) nfm).toPattern();
String npt = pt.replace("\u00A4", "").trim();
NumberFormat nfm2 = new DecimalFormat(npt);
nfm2.setMinimumFractionDigits(0);
//Return the attributes with message.
return "ID " + id + ":" + lastName + ", " + firstName
+ ", salary $" + nfm2.format(salary);
}
}
Executable Class
- create an array of Employee objects.
- create an ArrayList of Employee objects from that array.
- use an enhanced for loop to print all employees as shown in the sample output.
- create a TreeMap that uses Strings for keys and Employees as values.
- this TreeMap should map Employee ID numbers to their associated Employees.
- process the ArrayList to add elements to this map.
- print all employees in ID # order as shown, but do so using the TreeMap's forEach method and a lambda expression
Example output
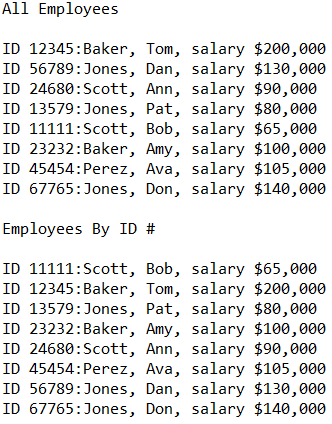

Step by stepSolved in 2 steps

- Inheritance The more general class is typically known as the parent class, super class, or base class. The more specific class is typically known as the child class, sub class, or derived class. The Java keyword extends allows you to indicate that one class should be the child class of the original parent class. The child will then inherit everything from the parent class, including variables and methods. {0} Online (1) Main.java x Student.java public class Main { 1 2 3 4 5 6 Run V 7 8 9 10 11 12 13 14 } public static void main(String[] args) { /* Person class example */ Person teacher = new Person(); teacher.setName("Laurel"); System.out.println(teacher); } Reid Moseley X Person.java x /* Student class example */ Student student = new Student (); student.setName("Alice"); System.out.println(student); CONSOLE SHELL Name: Laurel Name: Alice Reset to Template Open Desktoparrow_forwardDefining Classes Write and document the definition for the Money class. The Money class represents an amount in U.S. dollars. Money Class e Fields cents : int dollars : int e Methods getValue() : double Money(int d, int c) setCents(int c) : void setDollars(int d) : void toString() : String • Each Money object has two int instance variables: dollars and cents. • The class has one constructor that takes two int parameters. The first int represents whole dollars, and the second int represents cents. • The class has one accessor method named getValue() which returns the amount as a double. (Just divide cents by 100.0 and add it to dollars. Return that amount.) • The class has two mutator methods-setDollars() and setCents()–that modify the corresponding instance variables. • The class has a toString() method that returns the amount as a String in the form: $ 2345.75. Use concatenation to produce the output. Do not worry about the decimal places.arrow_forwardPYTHON CLASSES AND OBJECTarrow_forward
- Inheritance and UML (Java) Design a class named Employee. The class keeps the following information in fields: - Employee Name - Employee Number in the format XXX-L, where each X is a digit within the range 0-9 and the L is a letter within the range A-M. - Hire Date - Shift (an integer) - Hourly pay rate (a double) Write one or more constructors and the appropriate accessor and mutator methods for the class. The workday is divided into two shifts: day and night. The shift field will be an integer value representing the shift that the employee works. The day shift is shift1 and the night shift is shift 2. a) Draw a UML diagram of the class. Be sure to include notation showing each field and method’s access specification and data type. Also include notation showing any method parameters and their data types. b) Write a program that demonstrates the class.arrow_forwardPROGRAMMING LANGUAGE: C++ Write a program that has a base class named FlightCrew. This class shouldhave three data members: an integer to store the ID of the crew member, aninteger to store the number of years of service and another integer to storethe total salary of the member. Provide a parameterized constructor in theclass to set the values of the data members.Derive a class Pilot from FlightCrew to contain two additional data members,an integer to store the number of hours of flight and a boolean to storewhether the Pilot has military experience or not. Provide a paramete rizedconstructor in the Pilot class. Provide a function bonus() in the class wherethe bonus of a pilot is his number of flight hours times the 10% of his salary.Likewise, provide a function isEligible() in the class to find out if the pilot iseligible for promotion or not. A pilot is eligible for promotion to the nextrank if he has at least 5 years of experience and the number of total flighthours is greater…arrow_forwardJava - Product Classarrow_forward
- Given below is a UML diagram for address class. Address -Street: String -City: String -State: String -Postal code: String -country: String +methods a) Write the code for default constructor and set the values of the data fields to null? b) Write the code for overloaded constructor with formal parameters and set the values of the data fields to the parameter listing. c) Write the code for mutator and accessor methods. d) Write the code for printAddress() method that returns the full address.arrow_forwardjavaarrow_forwardPython Programming 3. Write an Employee class that keeps data attributes for the following pieces of information:(a). Employee name(b). Employee numberNext, write a class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information:(c). Shift number (an integer,such as1 for morning shift, 2 for evening shift)(d). Hourly payrateWrite the appropriate accessor and mutator methods for each class.arrow_forward
- C++arrow_forwardUse Java Programming Language Create a Loan Account Hierarchy consisting of the following classes: LoanAccount, CarLoan, PrimaryMortgage , UnsecuredLoan, and Address. Each class should be in it's own .java file. The LoanAccount class consists of the following properties: principal- the original amount of the loan. annualInterestRate - the annual interest rate for the loan. It is not static as each loan can have it's own interest rate. months - the number of months in the term of the loan, i.e. the length of the loan. and the following methods: a constructor that takes the three properties as parameters. calculateMonthlyPayment() - takes no parameters and calculates the monthly payment using the same formula as Assignment 1. getters for the three property variables. toString() - displays the information about the principle, annualInterestRate, and months as shown in the example output below. The CarLoan class which is a subclass of the LoanAccount class and consists of the…arrow_forwardCSC 1302: PRINCIPLES OF COMPUTER SCIENCE II Lab 6 How to Submit Please submit your answers to the lab instructor once you have completed. Failure to submit will result in a ZERO FOR THIS LAB. NO EXCEPTIONS. The class diagram with four classes Student, Graduate, Undergraduate, and Exchange is given. Write the classes as described in the class diagram. The fields and methods for each class is given below. Student Exchange Graduate Undergraduate Student: Fields: major (String) gpa (double) creditHours (int) Methods: getGpa: returns gpa getYear: returns “freshman”, “sophomore”, “junior” or “senior” as determined by earned credit hours: Freshman: Less than 32 credit hours Sophomore: At least 32 credit hours but less than 64 credit hours Junior: At least credit hours 64 but less than 96 credit hours Senior: At least 96 credit hours Graduate:…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
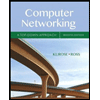
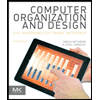
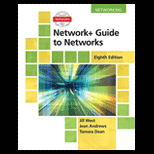
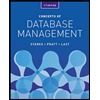
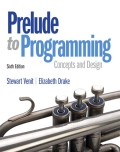
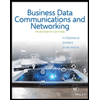