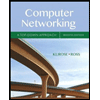
Create abstract class Pet , which is the abstract superclass of all animals.Declare a integer attribute called legs, which records the number of legs for this animal.Define a constructor that initializes the legs attribute.Declare an abstract method eat.Declare a concrete method walk that prints out something about how the animals walks (include the number of legs).Create the Dog class.The Dog class extends the Pet class.This class must include a String attribute to store the name and food of the petDefine a default constructor that calls the superclass constructor to specify that all dogs have 4 legs and name.override the eat and walk method.Create the Cat class that extends Pet.This class must include a String attribute to store the name of the pet, and a string to save food .Define a constructor that takes one String parameter that specifies the cat's name and food. This constructor must also call the superclass constructor to specify that all cats have four legs.Override walk method.Implement the eat method.Create the Fish class with fins, food this also extends pet, Override the Pet methods to specify that fish can't walk and don't have legs, implement eat method.Create a PetOwner Class which represents a person who have pets, this class contain name, age, and pet , Provide appropriate methods for the class.Create an TestAnimals program:
Create an arraylist of type PetOwner and then by asking about type of pet one want add that type pet to the arraylist.
e.g. if(choice== cat){
Cat cat=new Cat(“meow”);
PetOwner p=new PetOwner(“ali”,cat)’;
arraylist.add(p);
}
Same for dog and fish, at the end print arraylist also call eat and walk methods.
Use Inheritance, Abstraction, in java

Trending nowThis is a popular solution!
Step by stepSolved in 7 steps

- Construct a Time class containing integer data members seconds, minutes, and hours. Have the class contain two constructors: The first should be a default constructor having the prototype Time(int, int, int), which uses default values of 0 for each data member. The second constructor should accept a long integer representing a total number of seconds and disassemble the long integer into hours, minutes, and seconds. The final member method should display the class data members.arrow_forwardModify the Car class example and add the following: A default constructor that initializes strings to “N/A” and numbers to zero. An overloaded constructor that takes in initial values to set your fields (6 parameters). accel() method: a void method that takes a double variable as a parameter and increases the speed of the car by that amount. brake() method: a void method that sets the speed to zero. To test your class, you will need to instantiate at least two different Car objects in the main method in the CarDemo class. Each of your objects should use a different constructor. For the object that uses the default constructor, you will need to invoke the mutator methods to assign values to your fields. Invoke the displayFeatures() method for both objects and display the variables. Demonstrate the use of the accessor method getColor() by accessing the color of each car and printing it to the console. Use the method accel to increase the speed of the first car by 65 mph. Use…arrow_forward2. Student (extend the class Person) that has the following: Data Fields: private int ID prvate double grade Constructor: Constructor to create a Student object with specifiedname address, ID & grade. Methods: Accessor and mutator methods for all data fields.arrow_forward
- 1. Design a new Triangle class that extends the abstract GeometricObject class. Write a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filled. The program should create a Triangle object with these sides and set the color and filled properties using the input. The program should display the area, perimeter, color, and true or false to indicate whether it is filled or not.arrow_forwardDesign a console program that will print details of DVDs available to buy. Make use of an abstract class DVD with variables that will store the title, yearReleased, running time and price of a DVD. Create a constructor that accepts the title, yearReleased and running time through parameters and assign these to the class variables. Create an abstract set method for the price of a DVD; also create get methods for the variables.Create a subclass InstructionalDVD that extends the DVD class and implements an iPrintable interface. The interface that must be added is shown below:public interface iPrintable { String ShowDetails();}The InstructionalDVD subclass has a private variable named category for which a get method must be written. The constructor of the InstructionalDVD class must accept the title, yearReleased, running time and category through parameters. Write the code for the setPrice() and ShowDetails() methods.Write a useDVD class and instantiate 2 objects of the InstructionalDVD…arrow_forwardCreate an Account class that a bank might use to represent customers’ bank accounts. Include a data member to represent the account balance. Provide a constructor that receives an initial balance and uses it to initialize the data member. The constructor should validate the initial balance to ensure that it is greater than or equal to 0. If not, set the balance to 0. Provide three member functions:- credit($amount) should add amount to the current balance- debit($amount) should ensure that amount does not exceed the current balance and then reduce the current balance by amount. The balance should not change if amount exceeds the balance.- getBalance() should return the current balanceNote: Create a set of test data and then write a program to test the class.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
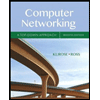
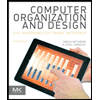
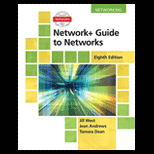
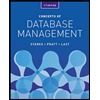
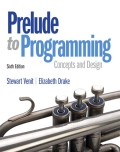
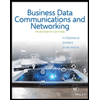