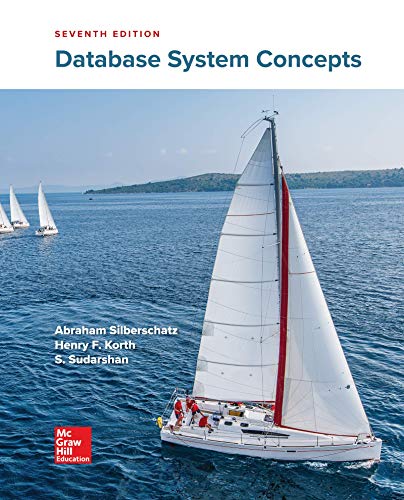
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
- Using Python
- Recursion is the concept of a function calling itself until the problem is solved when the Base Case is met.
- Study Recursion: See slides in Modules, Practice Slides, lab12 and also Recursion is in chapter 9 of the textbook.
- Some examples of recursion are: compute [ factorial of a number, towers of Hanoi, fractals (as shown in the textbook), and many more].
- Example: See slides Page 2
- For this assignment we'll use Collatz Cojecture (see Wikipedia).
- Collatz Conjecture
algorithm : - Given a number n, first call to the function: f(n):
- In the function:
- if n == 1 return 1:
- if n is even then f(n/2), i.e. call self with the new value.
- else (n is odd) call self with the new value, f((n*3)+1) and repeat. All numbers eventually end up with 1.
- The program should test for the base case which is: if n == 1, in which case it returns to the caller with 1.
- This problem is perfect to demonstrate Recursion.
Create a list with random numbers (you can just do this part manually) in the list as 1, 2, 3, 4, 5, 6 digit numbers, one or two of each. - Call the function from a loop going through the list with the numbers.
- Program should print each new number and at the end print the numbers of recursive calls.
- Example:
- Please enter an integer: 11
11
34
17.0
52.0
26.0
13.0
40.0
20.0
10.0
5.0
16.0
8.0
4.0
2.0
1.0 num visits: 15 - If you try this with 9, you'll see it will take more recursions.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Need help with this python recursive basic pathfinding question. I have the layout of the code but I need help filling in the parts that say "pass" and I need the output of the code to match the sample run below. Our goal is to find a path (not the best path, but just any path) from A to Z. You see that the green path is not the shortest but it does let us navigate from start to finish. (The picture below) First, we need to know A and Z, our starting and ending points. We'll pass these into our function. I'm going to use a dictionary to represent this graph. Each node (vertex, circle) will have a name, in this case "A" and "Z" were the names of the nodes, but in the generated maps I'm going to use "Node 1", "Node 2", "Node 3", etc. Here is an example web_map web_map = { 'Node 1': ['Node 3', 'Node 2'], 'Node 2': ['Node 1', 'Node 4'], 'Node 3': ['Node 1'], 'Node 4': ['Node 2'] } Node 1 is connected to 2 and 3 for instance, and then also note that Node 3 is connected back to…arrow_forwardUsing recursive functions in Python, given three letters in the alphabet, get their permutations together with the combinations of any two letters from it.arrow_forwardWrite a recursive function diff (java) which utilizes two positive integer arguments (x and y) and returns |x – y|. An x - y calculation may not be performed during the function. Thanks so much for your help!arrow_forward
- Define Data Abstraction.arrow_forwardWhy does dynamic programming provide faster solutions that recursive algorithms solving the same problem? 1.avoids resolving overlapping subproblems 2.dynamic programming uses dynamic memory that is more efficient than stack memory 3.loops are always faster than recursion 4.dynamic uses arrays that are faster than function callsarrow_forwardWhen recursion is used to solve a problem, why must the recursive method call itself to solve a smaller version of the original problem?arrow_forward
- There are two important parts to every simple recursive function: the base case, and the recursive call that makes progress towards the base case. Something that can go wrong with recursion when it is used incorrectly is a stack overflow. Explain two different ways that a recursive function could be written incorrectly that could lead to stack overflow. Hint: one has something to do with the base case, and the other with the recursive call. 1. Enter your answer here 2. Enter your answer herearrow_forwardIn Python: Which of the following is true about recursive functions? Can make some repetitive problems easier to solve and understand. Require less memory than non-recursive functions Faster to execute than non-recursive functions can only be called from the main functionarrow_forwardThe Fibonacci algorithm is a famous mathematical function that allows us to create a sequence of numbers by adding together the two previous values. For example, we have the sequence:1, 1, 2, 3, 5, 8, 13, 21…Write your own recursive code to calculate the nth term in the sequence. You should accept a positive integer as an input, and output the nth term of the sequence.Once you have created your code, add comments describing how the code works, and the complexity of any code you have created.arrow_forward
- Demonstrate Recursion Assignment Instructions Overview The programs we’ve discussed so far are generally structured as methods that call one another in a hierarchical manner. For some problems, it’s useful to have a method call itself—this is known as a recursive method. Such a method can call itself either directly or indirectly through another method. Recursion is an important topic discussed at length in upper-level computer-science courses. Instructions Write a recursive method printArray() that displays all the elements in an array of integers, separated by spaces. The array must be 100 elements in size and filled using a for loop and a random number generator. The pseudo-code for this is as follows: //Instantiate an integer array of size 100 //fill the array For (int i=0; i<array.length; i++) Array[i] = random number between 1 and 100 inclusive printArray(integer array); For this assignment make sure that your screen shots show your program running and that your runtime…arrow_forwardRecursion is a technique that calls the function by itself. Demonstrate and write a program to find the GCD of two numbers using recursion and mention the advantages of recursion.arrow_forwardWritten explaination requiredarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
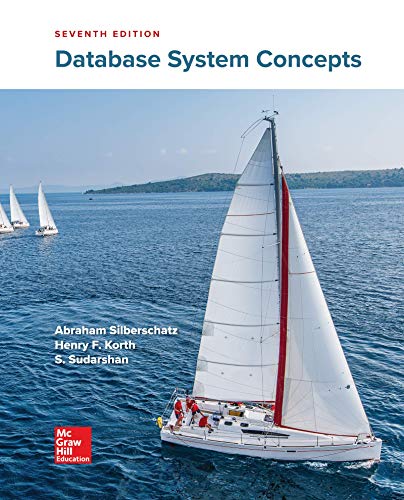
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
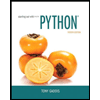
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
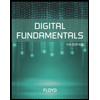
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
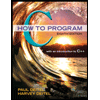
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
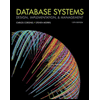
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
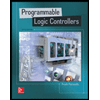
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education