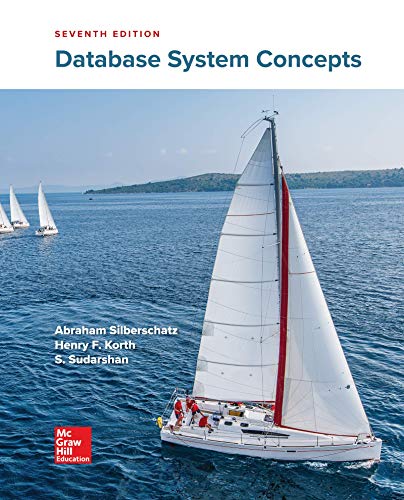
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
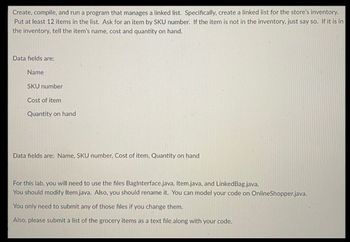
Transcribed Image Text:### Lab Assignment: Linked List Inventory Management
**Objective:**
Create, compile, and run a program that manages a linked list. Specifically, create a linked list for the store’s inventory.
**Instructions:**
1. Put at least 12 items in the list.
2. Ask for an item by SKU number.
- If the item is not in the inventory, just say so.
- If it is in the inventory, tell the item’s name, cost, and quantity on hand.
**Data Fields:**
- Name
- SKU number
- Cost of item
- Quantity on hand
**Data fields reiterated:**
- Name
- SKU number
- Cost of item
- Quantity on hand
**File Requirements:**
For this lab, you will need to use the following files:
- `BagInterface.java`
- `Item.java`
- `LinkedBag.java`
**Modifications:**
- Modify `Item.java`. Additionally, you should rename it.
- You can model your code on `OnlineShopper.java`.
**Submission Guidelines:**
- You only need to submit any of the above files if you change them.
- Submit a list of the grocery items as a text file along with your code.
![### Java Program to Search for an Item in a LinkedList
In this tutorial, we will explore a Java program that uses a `LinkedList` to store and search for items. This program prompts the user to enter the name of an item and then checks if the item exists in the list. If the item is found, it displays the item's details such as name, SKU number, unit cost, and quantity on hand.
#### Code Explanation
```java
import java.util.LinkedList;
import java.util.Scanner;
public class MyLL
{
public static void main(String[] args)
{
String newitem;
LinkedList<Item> l = new LinkedList<Item>();
l.add(new Item("soap", "1234567890", 10.5));
l.add(new Item("bag", "0987654321", 20.8));
l.add(new Item("spoon", "9876565431", 22.8));
l.add(new Item("wallet", "1234654321", 25.6));
l.add(new Item("lighter", "99812344321", 28.10));
l.add(new Item("purse", "9977614321", 26.21));
l.add(new Item("book", "9876745321", 7.8));
l.add(new Item("pen", "0187254321", 17.12));
l.add(new Item("laptop", "938563321", 1000.7));
l.add(new Item("mobile", "0987168881", 500.9));
l.add(new Item("basket", "0982653341", 20.81));
l.add(new Item("television", "998794929", 1020.6));
System.out.println("Please enter the item that you want to search in the list");
Scanner sc = new Scanner(System.in);
newitem = sc.next();
for(Item i : l)
{
if(i.item_name.equals(newitem))
{
System.out.println("The item is present in the list, here are the details:");
System.out.println("Item name: " + i.item_name);
System.out.println("SKU Number: " + i.SKU);
System.out.println("Unit Cost: " + i.unit_cost);
System.out.println("Quantity on hand: " + i.quantity_on_hand);](https://content.bartleby.com/qna-images/question/86b14285-d986-492a-886d-db2235fe87ed/5d178175-fd54-410d-89b3-a64d09d96b49/jgja6wp_thumbnail.jpeg)
Transcribed Image Text:### Java Program to Search for an Item in a LinkedList
In this tutorial, we will explore a Java program that uses a `LinkedList` to store and search for items. This program prompts the user to enter the name of an item and then checks if the item exists in the list. If the item is found, it displays the item's details such as name, SKU number, unit cost, and quantity on hand.
#### Code Explanation
```java
import java.util.LinkedList;
import java.util.Scanner;
public class MyLL
{
public static void main(String[] args)
{
String newitem;
LinkedList<Item> l = new LinkedList<Item>();
l.add(new Item("soap", "1234567890", 10.5));
l.add(new Item("bag", "0987654321", 20.8));
l.add(new Item("spoon", "9876565431", 22.8));
l.add(new Item("wallet", "1234654321", 25.6));
l.add(new Item("lighter", "99812344321", 28.10));
l.add(new Item("purse", "9977614321", 26.21));
l.add(new Item("book", "9876745321", 7.8));
l.add(new Item("pen", "0187254321", 17.12));
l.add(new Item("laptop", "938563321", 1000.7));
l.add(new Item("mobile", "0987168881", 500.9));
l.add(new Item("basket", "0982653341", 20.81));
l.add(new Item("television", "998794929", 1020.6));
System.out.println("Please enter the item that you want to search in the list");
Scanner sc = new Scanner(System.in);
newitem = sc.next();
for(Item i : l)
{
if(i.item_name.equals(newitem))
{
System.out.println("The item is present in the list, here are the details:");
System.out.println("Item name: " + i.item_name);
System.out.println("SKU Number: " + i.SKU);
System.out.println("Unit Cost: " + i.unit_cost);
System.out.println("Quantity on hand: " + i.quantity_on_hand);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- You may have found it somewhat tedious and unpleasant to use the debugger and visualizer to verify the correctness of your addFirst and addLast methods. There is also the problem that such manual verification becomes stale as soon as you change your code. Imagine that you made some minor but uncertain change to addLast]. To verify that you didn't break anything you'd have to go back and do that whole process again. Yuck. What we really want are some automated tests. But unfortunately there's no easy way to verify correctness of addFirst and addLast] if those are the only two methods we've implemented. That is, there's currently no way to iterate over our list and get bad its values and see that they are correct. That's where the toList method comes in. When called, this method returns a List representation of the Deque. For example, if the Deque has had addLast (5) addLast (9) addLast (10), then addFirst (3) called on it, then the result of toList() should be a List with 3 at the…arrow_forwardGiven the following program segment. Assume the node is in the usual info-link form with the info of the type int. (list and ptr are reference variable of the LinkedListNode type. What is the output of this program? list = new LinkedListNode (); list.info = 20; ptr = new LinkedListNode (); ptr.info = 28; ptr.link= null; list.link ptr; ptr = list; list = new LinkedListNode (); list.info = 55; list.link = ptr; ptr = new LinkedListNode (); ptr.info = 30; ptr.link= list; list = ptr; ptr = new LinkedListNode (); ptr.info = 42; ptr.link= list.link; list.link= ptr; ptr = list; while (ptr!= null) { } System.out.println (ptr.info); ptr = ptr.link;arrow_forwardIn C++,arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
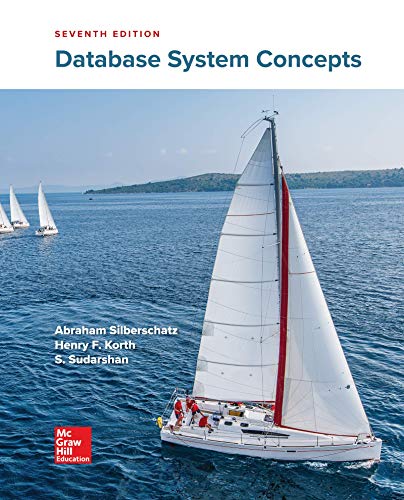
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
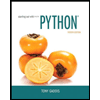
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
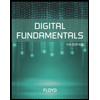
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
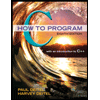
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
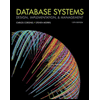
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
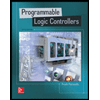
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education