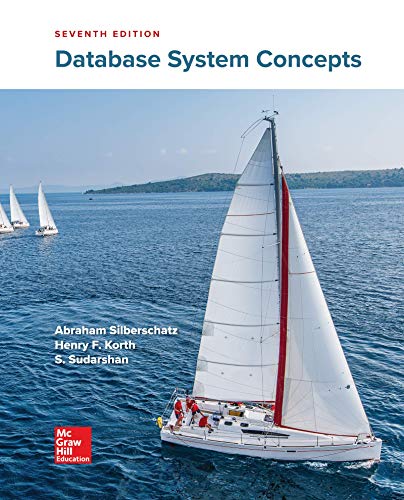
Concept explainers
Please written by computer source
Using C++, use a linked list to create an alphabetical Contact Book to store the names, addresses, and phone numbers of our contacts. The data structure used contain contacts should be a linked list and each time a new contact is added the contact will be inserted into the correct alphabetical location by last name. Assume there are no contacts that have the same last name. There will be two classes. One named LinkedList and the other named PersonNode.
The LinkedList class will be used to create a single LinkedList object.
- The class will have two PersonNode pointer fields
*headPtr that will maintain the head of the linked list
*tailPtr that will maintain the tail of the linked list
- The class will have the following functions::
*a constructor that initialized the headPtr and tailPtr to NULL
*addLink that will take a PersonNode reference or pointer and add the node to the linked list
*findInsertSpot that will return a PersonNode pointer that points to the location where a PersonNode is to be inserted into the linked list
*getHeadPtr that will return the headPtr
The PersonNode class will be used to create the nodes that are part of the linked list
- The class will have the following fields:
*fName - string contains the contacts first name
*lName - string contains the contact last name
*address - string contains the contacts address
*phone - string contains the contacts phone number
*next - PersonNode pointer that points to the next contact
- The class will contain all of the getters and setters for the above named fields (see class diagram below) in addition to
*getFullName - returns the string of lName, fName
*a constructor that takes no arguments and sets next to NULL
*a constructor that takes arguments to set fName, lName, address, and phone and sets next to NULL
- Finally, create the ability to search for a PersonNode entry from the Contact Book and remove a PersonNode entry from the Contact Book.
- Please include all .cpp, .h, and the main Source.cpp file with main.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- You are working for a major car manufacturer and have been tasked with creating an application to maintain vehicle inventory records for one of its dealerships. Create a class to maintain a Dealership. A dealership has the following information that must be stored in the Dealership class: the dealership name, the maximum number of vehicles that the dealership’s lot can hold, and a vector of the vehicles currently on the lot. The information for each vehicle should be encapsulated in a Vehicle class and should include the vehicle’s make, model, and year. Your Dealership class should support operations (functions) to add a vehicle to the dealership’s inventory (vector) and to list all vehicle records (i.e. print out the make, model, and year of each vehicle object in the vector). Your Vehicle class should include operations to allow entry of the make, model, and year, and to return the values in these variables (In other words, you need a separate setter and getter for each data…arrow_forwardplease help me with this JAVA programming 1 assignment. You are creating a database of fey creatures found in Western Arkansas. Your data includes the creature's species, description, diet, and difficulty to catch. Include the following in your database: Species Description Diet Danger Level Times Caught Sprite Small winged humanoid berries medium 1 Goblin Common evil fey Omnivorous medium 5 Troll Large, dangerous, ugly humanoids high 1 Boggart Mischievous teleporting goblin-like creature stolen pies low 4 Gnome Small, shy, burrowing burrowing critters low 2 Dryad tree-maiden spirit tied to a forest unknown medium 0 Using one or more arrays, display this list. Modify the program so the user enters a number. Then, display all the creatures (and their info) who have been caught at least that many times. In addition to the above, allow the user to enter the name of a creature. If that creature is in the list, add one to the number of times that creature has been…arrow_forwardfast in pyth pleasearrow_forward
- Which of the following lists are used to mean that a class has been derived from another class? super, base, parent sub, derived, child Object, super, equals None of thesearrow_forwardAs the first step in writing an application for the local book store, we will design and implement a Book class that will define the data structure of the application. Each book has a name, author, type (paperback, hardback, magazine), integer ID and pageCount. Provide some data validation in the appropriate method to ensure that the ID and pageCount are not negative. Design and implement a Book class with separation, i.e., separate all functions and methods into both the prototype and an implementation below the main. Provide constructor methods for Book(void), Book(name, author), and one for all components Book(name, author, type, ID, pageCount) Provide accessor (get and set) methods for each property as well as a display method. Provide data validation for the ID and pageCount in the appropriate methods. Provide a method to calculate the cost for buying books (with a 7.75% sales tax) according to the following chart: Hardcover: $29.95 per book Paperback:…arrow_forwardPart 2: Sorting the WorkOrders via dates Another error that will still be showing is that there is not Comparable/compareTo() method setup on the WorkOrder class file. That is something you need to fix and code. Implement the use of the Comparable interface and add the compareTo() method to the WorkOrder class. The compareTo() method will take a little work here. We are going to compare via the date of the work order. The dates of the WorkOrder are saved in a MM-DD-YYYY format. There is a dash '-' in between each part of the date. You will need to split both the current object's date and the date sent through the compareTo() parameters. You will have three things to compare against. You first need to check the year. If the years are the same value then you need to go another step to check the months, otherwise you compare them with less than or greater than and return the corresponding value. If you have to check the months it would be the same for years. If the months are the same you…arrow_forward
- Create an instance of the MovieDetails class called new_movie. Then, read three values from input into new_movie's attributes in the following order: year (int) duration (int) title (str)arrow_forwardComplete the docstring using the information attached (images):def upgrade_stations(threshold: int, num_bikes: int, stations: List["Station"]) -> int: """Modify each station in stations that has a capacity that is less than threshold by adding num_bikes to the capacity and bikes available counts. Modify each station at most once. Return the total number of bikes that were added to the bike share network. Precondition: num_bikes >= 0 >>> handout_copy = [HANDOUT_STATIONS[0][:], HANDOUT_STATIONS[1][:]] >>> upgrade_stations(25, 5, handout_copy) 5 >>> handout_copy[0] == HANDOUT_STATIONS[0] True >>> handout_copy[1] == [7001, 'Lower Jarvis St SMART / The Esplanade', \ 43.647992, -79.370907, 20, 10, 10] True """arrow_forwardTODO 2 Complete the TODO by finding the locations of the data samples for each iris flower type. Hint: Use the code for how we found all the setosa_locs as an example! Using iris_df, find the location of all the data sample locations for the versicolor class which has a label of 1. To do so, use NumPy's np.where() function. Using iris_df, find the location of all the data sample locations for the virginica class which has a label of 2. To do so, use NumPy's np.where() function. setosa_locs = np.where(iris_df['class'] == 0)[0] # TODO 2.1versicolor_locs = # TODO 2.2virginica_locs = todo_check([ (type(versicolor_locs) is np.ndarray, "'versicolor_locs' is not type np.ndarray. Make sure you indexed np.where() currently!"), (type(virginica_locs) is np.ndarray, "'virginica_locs' is not type np.ndarray. Make sure you indexed np.where() currently!"), (versicolor_locs[0] == 50, "'versicolor_locs' has an incorrect value"), (virginica_locs[0] == 100, "'virginica_locs' has an…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
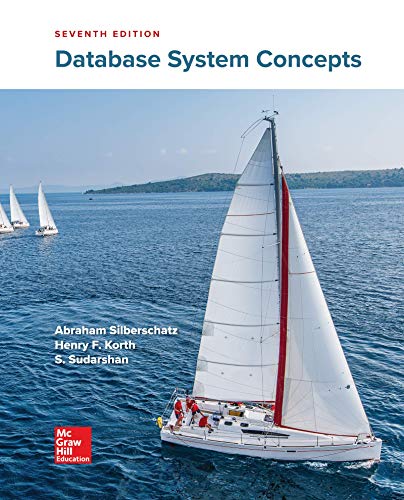
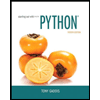
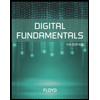
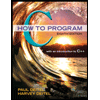
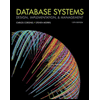
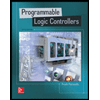