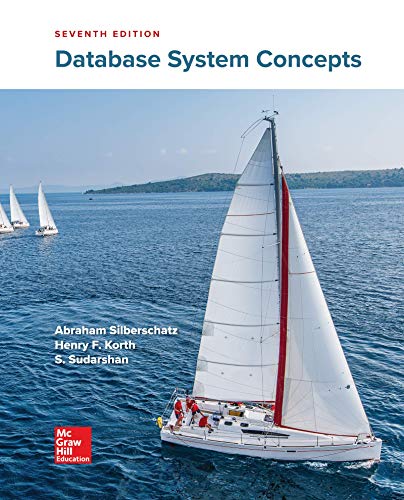
Concept explainers
CREATE TABLE EMPLOYEE(
ENUM DECIMAL(12) NOT NULL,/* Employee number */
FNAME VARCHAR(50) NOT NULL,/* First name */
LNAME VARCHAR(50) NOT NULL,/* Last name */
DOB DATE NULL,/* Date of birth */
CONSTRAINT EMPLOYEE_PKEY PRIMARY KEY(ENUM) );
CREATE TABLE DRIVER(
ENUM DECIMAL(12) NOT NULL,/* Employee number */
LNUM DECIMAL(8) NOT NULL,/* Driving license number */
STATUS VARCHAR(10) NOT NULL,/* Driver status */
CONSTRAINT DRIVER_PKEY PRIMARY KEY(ENUM),
CONSTRAINT DRIVER_UNIQUE UNIQUE(LNUM),
CONSTRAINT DRIVER_FKEY FOREIGN KEY(ENUM) REFERENCES EMPLOYEE(ENUM),
CONSTRAINT DRIVER_STATUS CHECK (
STATUS IN ('AVAILABLE', 'BUSY', 'ON LEAVE')) );
CREATE TABLE TRUCK(
REGNUM VARCHAR(10) NOT NULL,/* Registration number */
CAPACITY DECIMAL(7) NOT NULL,/* Capacity */
WEIGHT DECIMAL(7) NOT NULL,/* Weight */
STATUS VARCHAR(10) NOT NULL,/* Present status */
CONSTRAINT TRUCK_PKEY PRIMARY KEY(REGNUM),
CONSTRAINT TRUCK_STATUS CHECK
( STATUS IN ('AVAILABLE', 'USED', 'MAINTAINED')),
CONSTRAINT TRUCK_WEIGHT CHECK
( WEIGHT > 0.0 AND WEIGHT < 500 ),
CONSTRAINT TRUCK_CAPACITY CHECK
( CAPACITY > 0.0 AND CAPACITY < 100 ) );
CREATE TABLE TRIP(
TNUM DECIMAL(10) NOT NULL,/* Trip number */
LNUM DECIMAL(8) NOT NULL,/* Driving license number */
REGNUM VARCHAR(10) NOT NULL,/* Truck registration number */
TDATE DATE NOT NULL,/* Trip date */
CONSTRAINT TRIP_PKEY PRIMARY KEY (TNUM),
CONSTRAINT TRIP_CKEY UNIQUE (LNUM, REGNUM, TDATE),
CONSTRAINT TRIP_FKEY1 FOREIGN KEY (LNUM) REFERENCES DRIVER(LNUM),
CONSTRAINT TRIP_FKEY2 FOREIGN KEY (REGNUM) REFERENCES TRUCK(REGNUM) );
CREATE TABLE TRIPLEG(
TNUM DECIMAL(10) NOT NULL,/* Trip number */
LEGNUM DECIMAL(2) NOT NULL,/* Leg number */
DEPARTURE VARCHAR(30) NOT NULL,/* Departure city */
DESTINATION VARCHAR(30) NOT NULL,/* Destination city */
CONSTRAINT TRIPLEG_PKEY PRIMARY KEY (TNUM, LEGNUM),
CONSTRAINT TRIPLEG_UNIQUE UNIQUE(TNUM, DEPARTURE, DESTINATION),
CONSTRAINT TRIPLEG_FKEY1 FOREIGN KEY (TNUM) REFERENCES TRIP(TNUM) );
Refer to the above table
Write SQL statements that implement the following structural modifications of a
and data manipulations on the contents of a database. Note, that implementation of some
of the tasks listed below may need more than one SQL statement.
(1) We would like to add to a database information about the skills possessed by each
driver. Assume, that a driver has many skills and a skill can be possessed by many
drivers. It may happen that some skills are not possessed by any driver. The types of
the new columns are up to you.
(2) Delete from a database information about the second and third leg of a trip 36.
(3) Change a date of trip number 26 to the next day after the present date of trip 26.
(4) Assume that a trip number 25 has 3 legs. A trip number 25 started in Sydney and it
ended in Perth. Add one more leg to a trip 25 such that after update the trip ends in
the same city it started from.
(5) Assume that a trip 20 has 2 legs and that a destination of the first leg is Sydney.
Update information about a trip 20 such that after update a destination of the first leg
is Perth.

Step by stepSolved in 2 steps with 1 images

- //stored procedure create or replace procedure firstthree (my_seqid in varchar2) as cursor c1 is select sname, grade from student s join taken t on s.sid=t.sid where seqid=my_seqid order by grade desc; -- my_sname varchar2(40); my_sname student.sname%TYPE; -- my_grade number(2,1); my_grade taken.grade%TYPE; begin /* clear the my_tmp table */ delete from my_tmp; commit; open c1; for i in 1..3 loop fetch c1 into my_sname, my_grade; exit when c1%notfound; /* in case the number requested */ /* is more than the total */ /* number of enrolled students */ /* display the result */ dbms_output.put_line('name: ' || my_sname || ' ' || 'grade: ' || my_grade); /* put into temporary table */ insert into my_tmp values(my_sname, my_grade); commit; end loop; close c1; end; / -------------- //php <? $connection = oci_connect ("test", "test", "test"); if ($connection == false){ $e = oci_error();…arrow_forwardcreate database GA2gouse GA2gocreate table salesman(salesman_id int primary key, name varchar(30), city varchar(20), commission varchar(30));insert into salesman (salesman_id, name, city, commission) values (5001, 'James Hoog', 'New York', 0.15); insert into salesman (salesman_id, name, city, commission) values (5002, 'Nail Knite', 'Paris', 0.13); insert into salesman (salesman_id, name, city, commission) values (5005, 'Pit Alex', 'London', 0.11); insert into salesman (salesman_id, name, city, commission) values (5006, 'Mc Lyon', 'Paris', 0.14); insert into salesman (salesman_id, name, city, commission) values (5007, 'Paul Adam', 'Rome', 0.13); insert into salesman (salesman_id, name, city, commission) values (5003, 'Lauson Hen', 'San Jose', 0.12); Create table Customer (customer_id int primary key, cust_name varchar(30), city varchar(10), grade varchar(30), salesman_id int,CONSTRAINT FK_salesman_id FOREIGN KEY (salesman_id) REFERENCES salesman(salesman_id));insert into customer…arrow_forwardSQL CODE FOR For the players who show up in Batting, Bowling, and Fielding tables, create a list that shows their names, runs they have scored, wickets they have taken, and catches they have taken? table is in picture (bowling table is same as batting and fielding )arrow_forward
- Access Assignment Problem: JMS TechWizards is a local company that provides technical services to several small businesses in the area. The company currently keeps its technicians and clients’ records on papers. The manager requests you to create a database to store the technician and clients’ information. The following table contains the clients’ information. Client Number Client Name Street City State Postal Code Telephone Number Billed Paid Technician Number AM53 Ashton-Mills 216 Rivard Anderson TX 78077 512-555-4070 $315.50 $255.00 22 AR76 The Artshop 722 Fisher Liberty Corner TX 78080 254-555-0200 $535.00 $565.00 23 BE29 Bert's Supply 5752 Maumee Liberty Corner TX 78080 254-555-2024 $229.50 $0.00 23 DE76 D & E Grocery 464 Linnell Anderson TX 78077 512-555-6050 $485.70…arrow_forwardThe Save Transaction button depicted in the screen attached is used to save relevant data to the sales table and the salesdetails tables from the depicted schema. When this button is clicked it calls the saveTransaction() function that is within the PosDAO class, it passes to this function an ArrayList of salesdetails object, this list contains the data entered into the jTable which is the products and qty being sold.Write the saveTransaction function. You are to loop through the items and get the total sales, next you are to insert the current date and the total sales into the sales table. Reminder that the sales table SalesNumber field is set to AUTO-INCREMENT, hence the reason for only entering the total sales and current date in sales table.arrow_forwardINFO 2303 Database Programming Assignment : PL/SQL Practice Note: PL/SQL can be executed in SQL*Plus or SQL Developer or Oracle Live SQL. Write an anonymous PL/SQL block that will delete all patients for doctors that works in the Family Practice Verify that patients have been deleted accordingly. Then do a select * from patients where doc_id = (select doc_id from doctor where area = ‘Family Practice’. You should get no rows.arrow_forward
- First normal form says: a. No nonkey columns depend on another nonkey column b. Every column that's not part of the primary key is fully dependent on the primary key. c. Eliminate repeated fields. d. None of the abovearrow_forward• Write a simple stored function that Takes a gender as an input ● Find the staff whose gender matches the input Return the results Write a simple stored procedure that Takes a gender as an input Find the staff whose gender matches the input • Print the resultsarrow_forwardComposite main keys have prospective keys.arrow_forward
- How do you index a CLOB datatype for faster search operations?arrow_forwardWorksOn Database: Employee (EmployeeNumber, EmployeeName, BirthDate, Title, Salary, DepartmentNumber) Project (ProjectNumber, ProjectName, Budget, DepartmentNumber) Department (DepartmentNumber, DepartmentName, ManagerNumber) WorksOn (EmployeeNumber, ProjectNumber, Responsibility, Hours) Questions: 1) Write an SQL query that returns the project number and name for projects with a budget no greater than $1,050,000. 2) Write an SQL query that returns all works on records where hours worked is less than 5 and the responsibility is 'Manager'. 3) Write an SQL query that returns the employees (number and name only) who have a title of 'EE' or 'SA' and make more than $50,000. 4) Write an SQL query that returns the employees (name and salary only) in department 'D1' ordered by decreasing salary. 5) Write an SQL query that returns the departments (all fields) ordered by descending department name.arrow_forwardCreate a list to save the elements and search if a specific key is in the list. Header file typedef struct list { int array[100]; int size; }SqList; Source file #include <stdio.h> #include <stdlib.h> #include "list.h" void create(SqList *l)//create the sequential list of n elements { } void seqSearch(SqList *l, int key)//sequential search { } void binSearch(SqList *l, int x)//binary search { } void main()//main function { SqList *L; L=(SqList *)malloc(sizeof(SqList)); }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
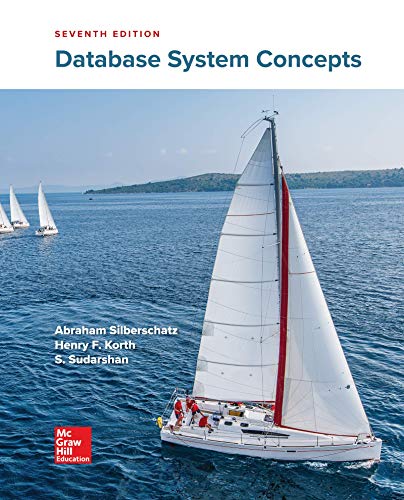
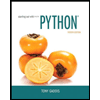
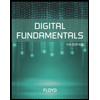
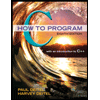
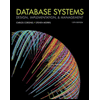
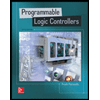