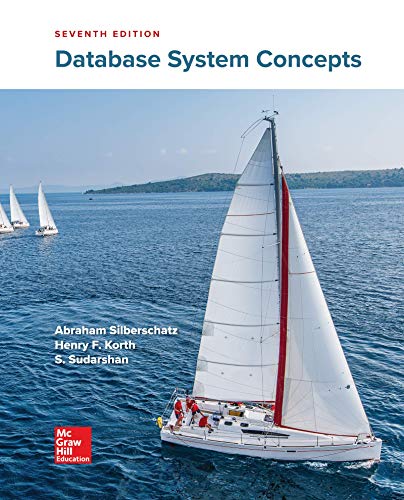
Define a method named sortArray that takes an array of integers and the number of elements in the array as parameters. Method sortArray() modifies the array parameter by sorting the elements in descending order (highest to lowest). Then write a main program that reads a list of integers from input, stores the integers in an array, calls sortArray(), and outputs the sorted array. The first input integer indicates how many numbers are in the list. Assume that the list will always contain less than 20 integers.
Ex: If the input is:
5 10 4 39 12 2
the output is:
39,12,10,4,2,
For coding simplicity, follow every output value by a comma, including the last one.
Your program must define and call the following method:
public static void sortArray(int[] myArr, int arrSize)
code:
import java.util.Scanner;
public class LabProgram {
/* Define your method here */
public static void main(String[] args) {
/* Type your code here. */
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write the following method that returns the sum of all numbersin an ArrayList:public static double sum(ArrayList<Double> list)Write a test program that prompts the user to enter five numbers, stores them inan array list, and displays their sum.arrow_forwardProblem: Write a method that will determine whether a given value can be made given an array of coin values. For example, each value in the array represents a coin with that value. An array with [1, 2, 3] represents 3 coins with the values, 1, 2, and 3. Determine whether or not these values can be used to make a desired value. The coins in the array are infinite and can only be used as many times as needed. Return true if the value can be made and false otherwise. Dynamic Programming would be handy for this problem. Data: An array containing 0 or more coin values and an amount. Output: Return true or false. Sample Data ( 1, 2, 3, 12, 5 ), 3 ( 4, 15, 16, 17, 1 ), 21 ( 1 ), 5 ( 3 ), 7 Sample Output true true true falsearrow_forwardIN JAVA Given an array, write a program to print the array elements one by one in a new line. If the array element is divisible by 4, print Four. If it is divisible by 6, print Six. If it is divisible by both 4 and 6, print Four Six. Use the following input array Int[] input_array = {2, 5, 10, 8, 18, 24, 3, 48, 30, 9, 12} Output should be 2 5 10 Four Six Four Six 3 Four Six Six 9 Four Sixarrow_forward
- 1. Write a program that calls a method that takes a character array as a parameter (you can initialize an array in the main method) and returns the number of vowels in the array. Partial program is given below. public class CountVowels{ public static void main(String[] args){ char[] arr= System.out.print("There are "+vowels(arr)+" vowels in the array");arrow_forwardJava - Sort an Arrayarrow_forwardjava a. A method named contains exists that takes an array of Strings and a single String, in that order, as arguments and returns a boolean value that is true if the array contains the given String. Assume that an array of Strings named names is already declared and initialized. Call the method to see if the array contains the name "Fred", and save the result in a new variable named fredIsHere. b. Given a method named printMessage that takes a String argument and returns nothing, call the method to print out "I love Java!".arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
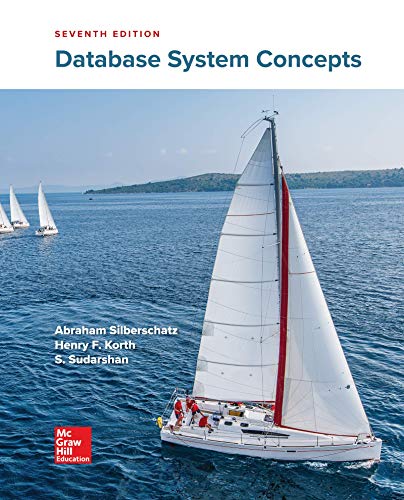
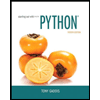
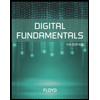
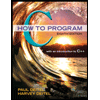
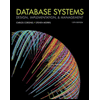
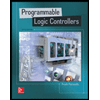