Using Java: Write the following method that returns the maximum value in an ArrayList of integers. The method returns null if the list is null or the list size is 0. public static Integer max(ArrayList list) Write a test program that prompts the user to enter a sequence of numbers ending with 0, and invokes this method to return the largest number in the input.
Using Java: Write the following method that returns the maximum value in an ArrayList of integers. The method returns null if the list is null or the list size is 0. public static Integer max(ArrayList list) Write a test program that prompts the user to enter a sequence of numbers ending with 0, and invokes this method to return the largest number in the input.
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 19RQ
Related questions
Question
Using Java:
Write the following method that returns the maximum value in an ArrayList of integers. The method returns null if the list is null or the list size is 0.
public static Integer max(ArrayList list)
Write a test
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
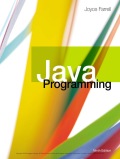
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
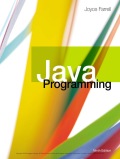
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT