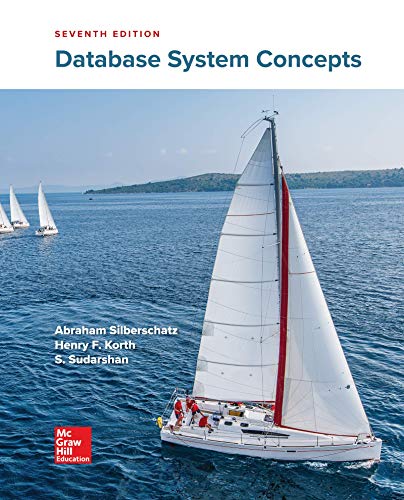
Describe the standard O(n^3) time dynamic

Dynamic programming: Matrix Chain Multiplication
Description In this assignment you are asked to implement a dynamic programming algorithm for the matrix chain multiplication problem , the goal is to find the most computationally efficient matrix order when multiplying an arbitrary number of matrices. in a row. you can assume that the entire input will be given as integers that can be stored using the standard C++ int type and that matrix sizes will be at least 1.
Input The input has the following format. The first number, n≥1, in the test case will tell you how many matrices are in the sequence. The first number will be then followed by n+1 is enough to fully specify the dimensions of the multiplied.
Output First you need to output the minimum number of scalar multiplication needed to multiply the given matrices. Then, print the matrix multiplication sequence, via parentheses, that minimizes the total number of number multiplication.
Each matrix should be named A#, where # is the matrix number starting at 0 (zero) and ending at n-1. see the examples below.
Examples of input and output:
2
2 3 5
30
(A0A1)
3
10 10)A2)
6
30 35 10 5 50
7500
((A0A1)A2)
3
10 30 5 60
4500
((A0A15 5 10 20 25
15125
((A0(A1A2))((A3A4)A5))
Recurrence relation:
A recurrence relation is an equation that uses recursion to relate terms in a sequence or elements in an array. It is a way to define a sequence or array in terms of itself. Recurrence relation have application in many areas of mathematics:
Sometimes, a recurrence relation can be "solved" by defining the terms of a sequence in terms of its index rather than previous terms in the sequence. This given a closed form expression for each term in the sequence and eliminates the need for an iterative process to solve for terms in the sequence. there are several ways to accomplish this:
Solving linear recurrence relations
solving recurrence relations with generating functions
solving recurrence relation with the substitution method
solving recurrence relation with the method of summation factors.
Even if the solution of this form is not possible, a recurrence relation is still useful, as it can use to develop computer algorithms. When terms in a sequence are stored, dynamic programming allows one to compute new terms in a sequence efficiently. Recurrence relation is also applicable for recursive backtracking, in which recursion is used to optimize algorithms.
Step by stepSolved in 3 steps

- Python programming language please help me understand this question with as much explanation as possible please.arrow_forwarda. Correctness of dynamic programming algorithm: Usually, a dynamic programming algorithm can be seen as a recursion and proof by induction is one of the easiest way to show its correctness. The structure of a proof by strong induction for one variable, say n, contains three parts. First, we define the Proposition P(n) that we want to prove for the variable n. Next, we show that the proposition holds for Base case(s), such as n = 0, 1, . . . etc. Finally, in the Inductive step, we assume that P(n) holds for any value of n strictly smaller than n' , then we prove that P(n') also holds. Use the proof by strong induction properly to show that the algorithm of the Knapsack problem above is correct. b. Bounded Knapsack Problem: Let us consider a similar problem, in which each item i has ci > 0 copies (ci is an integer). Thus, xi is no longer a binary value, but a non-negative integer at most equal to ci , 0 ≤ xi ≤ ci . Modify the dynamic programming algorithm seen at class for this…arrow_forwardA. Construct a RECURSIVE solution for following iterative solution to find a value in a circular-linked list B. Analyze the runtime complexity (correctly explain the scenario, show how much work would be done, and represent the work using asymptotic notations, i.e. big O), of the given iterative solution and your recursive solution for the best case scenario and the worse case scenario. C. prove the correctness of your recursive solution by induction /**@param value - a value to search for@return true if the value is in the list and set the current reference to itotherwise return false and not updating the current reference*/public boolean find(T value){ if(this.cur == null) return false; //get out, nothing is in here Node<T> tmp = this.cur; //start at the current position if(tmp.data == value) return true; // found it at the starting location tmp = tmp.next; //adv. to next node, if there is one while(tmp != cur) { if(tmp.data == value){ this.cur = tmp;…arrow_forward
- 4. Let A be a m x n matrix where each element is 0 or 1. We are interested in finding the largest square sub-matrix of A such that each of its elements is 1. Design a dynamic-programming algorithm, running in O(mn) time, that finds such a largest [1 0 0 1 1 1 1 1 1 1 101 0 square sub-matrix in A. For example, let A = 0 1 1 1 1 0 1 1 1 1 1 1 1 10 In this case, the 3 × 3 square submatrix formed by columns 2, 3, 4 and rows 4, 5, 6 of all 1s should be returned by the algorithm.arrow_forward[a] Prove that the "Bin Packing Problem" (BPP) is NP-Complete. Like the rest of the exam, you may use online resources but should cite your source and summarize the proof in your own words. [b] Write an approximation algorithm for the online version of BPP that randomly considers up to three partially filled bins, and if none have room, then create a new bin and use that. [c] What modification to your solution in [b] would you recommend to solve the offline version of BPP. [d] In light of your prior knowledge of and experience with approximation algorithms for BPP, provide a reasonable estimate of the error boundarrow_forwardSolutions to a dynamic programming problem have (at minimum) three things: – A recurrence relation – A brief description of what your recurrence function represents and what each case represents. – A brief description of the memory element/storage and how it’s filled in. – Always give complete solutions, not just examples.– Always declare all your variables, in English. In particular, always describe the specific - problem your algorithm is supposed to solve. – Never use weak induction.arrow_forward
- Solutions to a dynamic programming problem have (at minimum) three things: – A recurrence relation – A brief description of what your recurrence function represents and what each case represents. – A brief description of the memory element/storage and how it’s filled in. – Always give complete solutions, not just examples.– Always declare all your variables, in English. In particular, always describe the specific - problem your algorithm is supposed to solve. – Never use weak induction.arrow_forwardPlease Help ASAP!!!!arrow_forwardPlease solve using C++arrow_forward
- Answer by hand an analysis of the complexity of the distribution, sorting, and searching algorithms. Use T(n) for explaining the time complexity and O(n) for the Big O notation. What are the best, average, and worst-case scenarios? For: Iterative, recursive distribution and merge sort for sorting and binary search for searching. Show the equations and how they are dirived also explain if the number of input increases or decrease what is the effect.arrow_forwardThis is a practice question from my Data Structures course: h(n) = {1 if n = 1; 3 × h(n - 1) - 1 otherwise} Rewrite h(n) using an explicit formula that is not recursive (i.e., succinctly express h(n) as a polynomial/exponential function of n). Also, prove the correctness of your expression using mathematical induction. Please explain how to do this (as opposed to simply providing the answer). Thank you for your assistance.arrow_forward5. Find a closed form representation for the function defined recursively by f(0) = 5 and f(n+1)=2f(n)+4". Prove that your representation is correct using an inductive argument.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
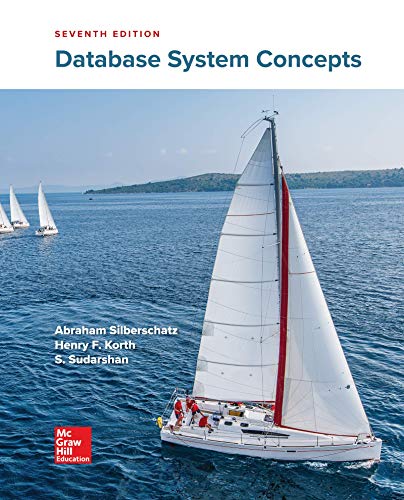
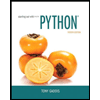
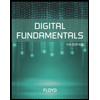
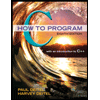
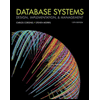
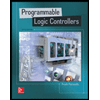