Question
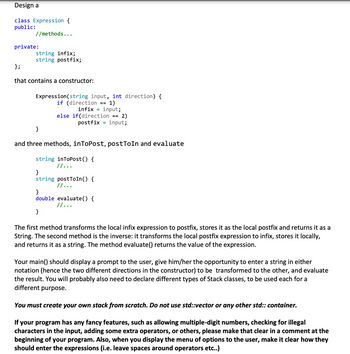
Transcribed Image Text:Design a
class Expression {
public:
//methods...
private:
};
string infix;
string postfix;
that contains a constructor:
Expression(string input, int direction) {
if (direction == 1)
infix = input;
else if(direction == 2)
postfix input;
}
and three methods, inToPost, postToIn and evaluate
string inToPost() {
// ...
}
string postToIn() {
// ...
}
double evaluate() {
// ...
}
The first method transforms the local infix expression to postfix, stores it as the local postfix and returns it as a
String. The second method is the inverse: it transforms the local postfix expression to infix, stores it locally,
and returns it as a string. The method evaluate() returns the value of the expression.
Your main() should display a prompt to the user, give him/her the opportunity to enter a string in either
notation (hence the two different directions in the constructor) to be transformed to the other, and evaluate
the result. You will probably also need to declare different types of Stack classes, to be used each for a
different purpose.
You must create your own stack from scratch. Do not use std::vector or any other std:: container.
If your program has any fancy features, such as allowing multiple-digit numbers, checking for illegal
characters in the input, adding some extra operators, or others, please make that clear in a comment at the
beginning of your program. Also, when you display the menu of options to the user, make it clear how they
should enter the expressions (i.e. leave spaces around operators etc..)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- A class object can encapsulate more than one [answer].arrow_forwardplease fix what is wrong with the below C# code: using System; namespace Animals{ class Animals { public static bool CheckGuess(string animalType, string typeGuess) { static bool Main(string[] args, string animalType, string typeGuess) { if (String.Equals(animalType, typeGuess)){ return true; } return false; } string animalType = "Dog"; string typeGuess; int count = 0; bool result; Console.Write("Guess the animal: "); typeGuess = Console.ReadLine(); while(true){ count++; result = CheckGuess(animalType, typeGuess); if(result){ Console.WriteLine("You have Guess it Correct in " + count + " times.\n"); break; } else{ Console.WriteLine("You have Guess it Wrong " + count + " times!!\n"); Console.Write("Guess the animal again: "); typeGuess = Console.ReadLine();…arrow_forwardpublic class ItemToPurchase { private String itemName; private int itemPrice; private int itemQuantity; // Default constructor public ItemToPurchase() { itemName = "none"; itemPrice = 0; itemQuantity = 0; } // Mutator for itemName public void setName(String itemName) { this.itemName = itemName; } // Accessor for itemName public String getName() { return itemName; } // Mutator for itemPrice public void setPrice(int itemPrice) { this.itemPrice = itemPrice; } // Accessor for itemPrice public int getPrice() { return itemPrice; } // Mutator for itemQuantity public void setQuantity(int itemQuantity) { this.itemQuantity = itemQuantity; } // Accessor for itemQuantity public int getQuantity() { return itemQuantity; }} import java.util.Scanner; public class ShoppingCartPrinter { public static void main(String[] args) { Scanner scnr = new…arrow_forward
- int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // *** returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // *** move the center of the circle to the new coordinates +intersects(Circle other): bool //*** returns true if the center of the other circle lies inside this circle else returns false +resize(double…arrow_forwardJava A class always has a constructor that does not take any parameters even if there are other constructors in the class that take parameters. Choose one of the options:TrueFalsearrow_forwardPython language Write a initializer method for the class Test. It has 2 parameters/field variables: correct and totalarrow_forward
- public class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardSubclass toString should call include super.toString(); re-submit these codes. public class Vehicle { private String registrationNumber; private String ownerName; private double price; private int yearManufactured; Vehicle [] myVehicles = new Vehicle[100]; public Vehicle() { registrationNumber=""; ownerName=""; price=0.0; yearManufactured=0; } public Vehicle(String registrationNumber, String ownerName, double price, int yearManufactured) { this.registrationNumber=registrationNumber; this.ownerName=ownerName; this.price=price; this.yearManufactured=yearManufactured; } public String getRegistrationNumber() { return registrationNumber; } public String getOwnerName() { return ownerName; } public double getPrice() { return price; } public int getYearManufactured() { return yearManufactured; }…arrow_forwardpublic class Course { private String courseNumber, courseTitle; public Course() { } public Course(String courseNumber, String courseTitle) { this.courseNumber = courseNumber; this.courseTitle = courseTitle; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseTitle(String courseTitle) { this.courseTitle = courseTitle; } public String getCourseNumber() { return courseNumber; } public String getCourseTitle() { return courseTitle; } public void printInfo() { System.out.println("Course Information: "); System.out.println(" Course Number: " + courseNumber); System.out.println(" Course Title: " +…arrow_forward
- Indicate each error or mistake in this codearrow_forwardTrue or False Constructors can accept arguments in the same way as other methods.arrow_forwardclass TenNums {private: int *p; public: TenNums() { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = i; } void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }};int main() { TenNums a; a.display(); TenNums b = a; b.display(); return 0;} Continuing from Question 4, let's say I added the following overloaded operator method to the class. Which statement will invoke this method? TenNums TenNums::operator+(const TenNums& b) { TenNums o; for (int i = 0; i < 10; i++) o.p[i] = p[i] + b.p [i]; return o;} Group of answer choices TenNums a; TenNums b = a; TenNums c = a + b; TenNums c = a.add(b);arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios