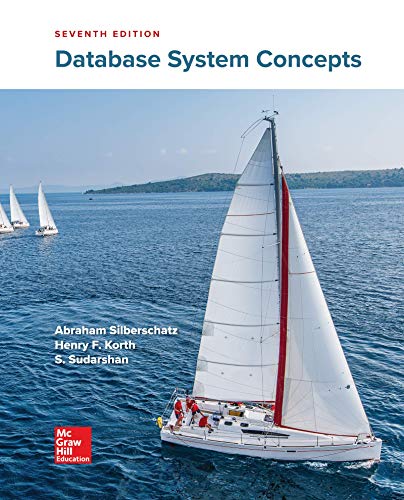
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
def get_task(task_collection, ...):
"""
param: task_collection (list) - holds all tasks;
maps an integer ID/index to each task object (a dictionary)
param: ... (str) - a string that is supposed to represent
an integer ID that corresponds to a valid index in the
collection
returns:
0 - if the collection is empty.
-1 - if the provided parameter is not a string or if it is not
a valid integer
Otherwise, convert the provided string to an integer;
returns None if the ID is not a valid index in the list
or returns the existing item (dict) that was requested.
"""
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Data structures unique_values(a_dict:dict)-> dict This function will receive a single dictionary parameter known as a_dict. a_dict will contain a single letter as a string and numbers as values. This function is supposed to search a_dict to find all values that appear only once in the a_dict. The function will create another dictionary named to_ret. For all values that appear once in a_dict the function will add the value as a key in to_ret and set the value of the key to the key of the value in a_dict (swap the key-value pairs, since a_dict contains letters to numbers, to_ret will contain Numbers to Letters). Finally, the function should return to_ret. Example: a_dict: {'X': 2, 'Y': 5, 'N': 2, 'L': 2, 'W': 1, 'G': 0, 'R': 1} Expected: {5: 'Y', 0: 'G'} a_dict: {'Z': 3, 'P': 3, 'E': 2, 'G': 0, 'T': 5, 'L': 1, 'Q': 0} to_ret: {2: 'E', 5: 'T', 1: 'L'} a_dict: {'E': 3, 'X': 3} to_ret: {} a_dict: {'G': 3, 'D': 3, 'C': 4, 'Q': 1, 'H': 1, 'M': 2, 'Z': 1, 'W': 3} to_ret: {4: 'C', 2: 'M'}…arrow_forwardclass Stack: def __init__(self): self.items = [] def is_empty(self): return not self.items def push(self, items): self.items.append(items) def pop(self): return self.items.pop() def peek(self): return self.items[-1] def size(self): return len(self.items) def __str__(self): return str(self.items) if __name__ == "__main__": s = Stack() print(s) print(s.is_empty()) s.push(3) print(s) s.push(7) s.push(5) print(s) print(s.pop()) print(s) print(s.peek()) print(s.size()) HOW TO REVERSE THIS PYTHON CODE USING STACKS AND BY NOT USING SLICING?arrow_forwardUnique Values This function will receive a single map parameter known as a_map. a_map will contain a single letter as a string and numbers as values. This function is supposed to search a_map to find all values that appear only once in a_map. The function will create another map named to_ret. For all values that appear once in a_map the function will add the value as a key in to_ret and set the value of the key to the key of the value in a_map (swap the key-value pairs, since a_map contains letters to numbers, to_ret will contain Numbers to Letters). Finally, the function should return to_ret. Signature: public static HashMap<String, Integer> uniqueValues(HashMap<Integer, String> a_map) Example: INPUT: {0=M, 2=M, 3=M, 5=M, 6=n, 7=M, 9=M}OUTPUT: {n=6} INPUT: {0=A, 1=c, 2=c, 4=c, 5=a, 8=Q, 9=c}OUTPUT: {A=0, a=5, Q=8}arrow_forward
- Python S3 Get File In the Python file, write a program to get all the files from a public S3 bucket named coderbytechallengesandbox. In there there might be multiple files, but your program should find the file with the prefix and cb - then output the full name of the file. You should use the boto3 module to solve this challenge. You do not need any access keys to access the bucket because it is public. This post might help you with how to access the bucket. Example Output ob name.txt Browse Resources Search for any help or documentation you might need for this problem. For exampler array indexing, Ruby hash tables, etc.arrow_forwardA WriteOnly attribute may be implemented automatically. True vs. Falsearrow_forwardcreateDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forward
- Pointer variable stores the address where another object resides and system will automatically do garbage collection. Group of answer choices True Falsearrow_forwardBackground Often, data are stored in a very compact but not human-friendly way. Think of how dishes in a menu can be stored in a restaurant database somewhere in the cloud. One way a dish object can be stored is this: { "name": "Margherita", "calories": 800, "price": 18.90, "is_vegetarian": "yes", "spicy_level": 2 } Obviously, this format is not user-readable, so the restaurant's frontend team produced many lines of code to render and display this content in a way that's more understandable to us, the restaurant users. We have a mini-version of the same task coming up. Given a list of similar complex objects (represented by Python dictionaries), display them in a user-friendly way that we'll define in these instructions. What is a "dish"? In this project, one dish item is a dictionary object that is guaranteed to have the following keys: "name": a string that stores the dish's name. "calories": an integer representing the calorie intake for one serving of the dish. "price": a float to…arrow_forwardWhat is a way to safely dereference a pointer?arrow_forward
- dictionaries = [] dictionaries.append({"First":"Bob", "Last":"Jones"}) dictionaries.append({"First":"Harpreet", "Last":"Kaur"}) dictionaries.append({"First":"Mohamad", "Last":"Argani"}) for i in range(0, len(dictionaries)): print(dictionaries[i]['First']) ************************************************* please modity the above code to "Add an ‘if’ statement(s) to the loop in your solution in Exercise 7 to only show the full name if the first name is “Bob” or if the last name is “Kaur”.arrow_forwardClasses, Objects, Pointers and Dynamic Memory Program Description: This assignment you will need to create your own string class. For the name of the class, use your initials from your name. The MYString objects will hold a cstring and allow it to be used and changed. We will be changing this class over the next couple programs, to be adding more features to it (and correcting some problems that the program has in this simple version). Your MYString class needs to be written using the .h and .cpp format. Inside the class we will have the following data members: Member Data Description char * str pointer to dynamic memory for storing the string int cap size of the memory that is available to be used(start with 20 char's and then double it whenever this is not enough) int end index of the end of the string (the '\0' char) The class will store the string in dynamic memory that is pointed to with the pointer. When you first create an MYString object you should…arrow_forwardOctave assignment 1-Introduction to Octave Introduction This assignment is meant to be a gentle introduction to Octave, the free version of Matlab. It assumes that you have no prior coding experience. Objectives Download Octave and run it or use https://octave-online.net/ Learn the basics of the Octave GUI. • Learn how to create a short executable file called an m-file (.m extension) and run it. • Learn what a data type is. • Learn how to declare variables of different data types. • Learn how to create matrices. • Learn how to use several of Octave’s functions for creating objects with random numbers. Instructions 1.) Create a file call it with the form exercise_1_first name_last name. Include the underscores in your file name. At the top of the file add the comment "“My first Octave assignment. I'm so excited, I just can't hide it." 2.) Create the following variables a = 2.3; b = -87.3; A = [1,2; 4,5]; Create a matrix 2 × 2 B using the rand() function. Create two random complex…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
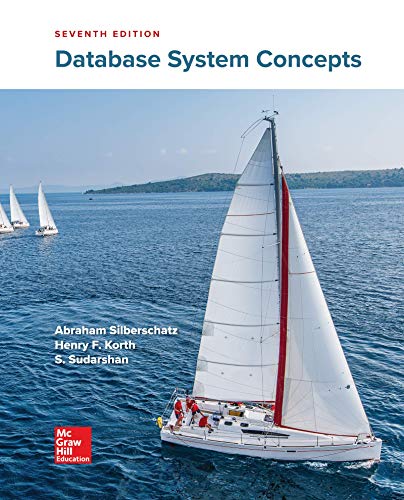
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
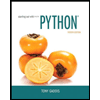
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
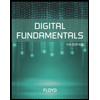
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
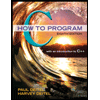
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
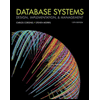
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
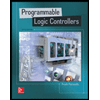
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education