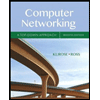
#include<iostream>
#include<cstdlib>
#include<string>
#include<cstdio>
using namespace std;
const int TABLE_SIZE = 128;
/*
* HashEntry Class Declaration
*/
class HashEntry
{
public:
int key;
int value;
HashEntry(int key, int value)
{
this->key = key;
this->value = value;
}
};
/*
* HashMap Class Declaration
*/
class HashMap
{
private:
HashEntry **table;
public:
HashMap()
{
table = new HashEntry * [TABLE_SIZE];
for (int i = 0; i< TABLE_SIZE; i++)
{
table[i] = NULL;
}
}
/*
* Hash Function
*/
int HashFunc(int key)
{
return key % TABLE_SIZE;
}
/*
* Insert Element at a key
*/
void Insert(int key, int value)
{
int hash = HashFunc(key);
while (table[hash] != NULL && table[hash]->key != key)
{
hash = HashFunc(hash + 1);
}
if (table[hash] != NULL)
delete table[hash];
table[hash] = new HashEntry(key, value);
}
/*
* Search Element at a key
*/
int Search(int key)
{
int hash = HashFunc(key);
while (table[hash] != NULL && table[hash]->key != key)
{
hash = HashFunc(hash + 1);
}
if (table[hash] == NULL)
return -1;
else
return table[hash]->value;
}
/*
* Remove Element at a key
*/
void Remove(int key)
{
int hash = HashFunc(key);
while (table[hash] != NULL)
{
if (table[hash]->key == key)
break;
hash = HashFunc(hash + 1);
}
if (table[hash] == NULL)
{
cout<<"No Element found at key "<<key<<endl;
return;
}
else
{
delete table[hash];
}
cout<<"Element Deleted"<<endl;
}
~HashMap()
{
for (int i = 0; i < TABLE_SIZE; i++)
{
if (table[i] != NULL)
delete table[i];
delete[] table;
}
}
};
/*
* Main Contains Menu
*/
int main()
{
HashMap hash;
int key, value;
int choice;
while (1)
{
cout<<"\n----------------------"<<endl;
cout<<"Operations on Hash Table"<<endl;
cout<<"\n----------------------"<<endl;
cout<<"1.Insert element into the table"<<endl;
cout<<"2.Search element from the key"<<endl;
cout<<"3.Delete element at a key"<<endl;
cout<<"4.Exit"<<endl;
cout<<"Enter your choice: ";
cin>>choice;
switch(choice)
{
case 1:
cout<<"Enter element to be inserted: ";
cin>>value;
cout<<"Enter key at which element to be inserted: ";
cin>>key;
hash.Insert(key, value);
break;
case 2:
cout<<"Enter key of the element to be searched: ";
cin>>key;
if (hash.Search(key) == -1)
{
cout<<"No element found at key "<<key<<endl;
continue;
}
else
{
cout<<"Element at key "<<key<<" : ";
cout<<hash.Search(key)<<endl;
}
break;
case 3:
cout<<"Enter key of the element to be deleted: ";
cin>>key;
hash.Remove(key);
break;
case 4:
exit(1);
default:
cout<<"\nEnter correct option\n";
}
}
return 0;
}
Based on the code above.
Update the code for Hash Tables so it will Accept an integer key and Map to String Value.
ID (Key) |
Namee |
1234 |
“Jan Smith” |
3445 |
“John Do” |
9762 |
“Jane Collins” |
1981 |
“Bill Rodgers” |
8653 |
“Eric Jones” |
4321 |
“Jill Wright” |
“8763” |
“Karen Bader” |
Use Conventional Method Names Insert, Remove, Search:
insert(key, value)
insert(ID, “NAMe”)
Search(“id”) → Method will return value
Remove(“id”) → Method will delete key and value

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- C++ Programming Redesign your class myArray using class templates so that the class can be used in any application that requires arrays to process data. #include <iostream>#include "myArray.h" using namespace std; int main(){myArray list1(5);myArray list2(5); int i; cout << "list1 : ";for (i = 0; i < 5; i++)cout << list1[i] << " ";cout << endl; cout << "Enter 5 integers: ";for (i = 0; i < 5; i++)cin >> list1[i];cout << endl; cout << "After filling list1: "; for (i = 0; i < 5; i++)cout << list1[i] << " ";cout << endl; list2 = list1;cout << "list2 : ";for (i = 0; i < 5; i++)cout << list2[i] << " ";cout << endl; cout << "Enter 3 elements: "; for (i = 0; i < 3; i++)cin >> list1[i];cout << endl; cout << "First three elements of list1: ";for (i = 0; i < 3; i++)cout << list1[i] << " ";cout << endl; myArray list3(-2, 6); cout <<…arrow_forwardAssign pizzasInStore's first element's numCalories with the value in pizzasInStore's second element's numCalories. #include <stdio.h>#include <string.h> typedef struct Pizza_struct { char pizzaName[75]; int numCalories;} Pizza; int main(void) { Pizza pizzasInStore[2]; scanf("%s", pizzasInStore[0].pizzaName); scanf("%d", &pizzasInStore[0].numCalories); scanf("%s", pizzasInStore[1].pizzaName); scanf("%d", &pizzasInStore[1].numCalories); /* Your code goes here */ printf("A %s slice contains %d calories.\n", pizzasInStore[0].pizzaName, pizzasInStore[0].numCalories); printf("A %s slice contains %d calories.\n", pizzasInStore[1].pizzaName, pizzasInStore[1].numCalories); return 0;} I tried 10 time but I cant solve it so please help mearrow_forwardCheck 1 ALLLEURE #include #include using namespace std; void PrintSize(vector numsList) { cout intList (2); PrintSize(intList); cin >> currval; while (currVal >= 0) { } Type the program's output intList.push_back(currval); cin >> currval; PrintSize(intList); intList.clear(); PrintSize(intList); return 0; CS Scanned with Calin canner Janviantars Input 12345-1 Output Feedback? 口口。arrow_forward
- #include #include #include "Product.h" using namespace std; int main() { vector productList; Product currProduct; int currPrice; string currName; unsigned int i; Product resultProduct; cin>> currPrice; while (currPrice > 0) { } cin>> currPrice; main.cpp cin>> currName; currProduct.SetPriceAndName (currPrice, currName); productList.push_back(currProduct); resultProduct = productList.at (0); for (i = 0; i < productList.size(); ++i) { Type the program's output Product.h 1 CSE Scanned Product.cpp if (productList.at (i).GetPrice () < resultProduct.GetPrice ()) { resultProduct = productList.at(i); } AM cout << "$" << resultProduct.GetPrice() << " " << resultProduct. GetName() << endl; return 0; Input 10 Cheese 6 Foil 7 Socks -1 Outputarrow_forwardC++: Consider the following code: struct node { int data; node *next; }; node myNode; node *p = &myNode; Which of these will set member variable data of myNode to the value 7? A) node->data = 7; B) p->node = 7; C) p.node = 7; D) myNode.data = 7;arrow_forwardPart-2 Debugging Debug#0int main(){char* ptr = nullptr;int size; cout << "Enter the size of the sentence: "; cin >> size; ptr = new char[size];char* ptr2 = ptr; cout << static_cast<void*>(&ptr) << endl;cout << static_cast<void*>(&ptr2) << endl; cout << "\nEnter your sentence:" << endl;for (int i = 0; i < size; i++){cin >> *(ptr + i);} cout << *ptr << endl;cout << ptr[size - 1]; while (static_cast<void*>(&ptr) <= static_cast<void*>(&ptr[size -1])){cout << "HI" << endl;cout << static_cast<void*>(&ptr) << endl;static_cast<void*>(&ptr);ptr++;} }arrow_forward
- Write a function getNeighbors which will accept an integer array, size of the array and an index as parameters. This function will return a new array of size 2 which stores the neighbors of the value at index in the original array. If this function would result in returning garbage values the new array should be set to values {0,0} instead of values from the array.arrow_forwardmain.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forward#include <iostream> #include <iomanip> #include <string> using namespace std; struct Teletype { string name; string phonenum; Teletype *nextaddr; }; void populate(Teletype *); void displayrecord(Teletype *); //void insertrecord(Teletype *); // create //void removerecord(Teletype *); //create //void modifyrecord(Teletype *); // create //int find(TeleType *, string); // Extra Credit create bool check(); int main() { int location = 0; int count = 0; char answery_n; Teletype *list, *current; list = new Teletype; current = list; cout << "Please "; do { count++; populate(current); if (check() == false) { cout << " Not storage available" << endl; } else { current->nextaddr = new Teletype; current = current->nextaddr;…arrow_forward
- In C++ struct myGrades { string class; char grade; }; Declare myGrades as an array that can hold 5 sets of data and then set a class string in each position as follows: “Math” “Computers” “Science” “English” “History” and give each class a letter gradearrow_forward#include <iostream>#include <cstdlib>using namespace std; class IntNode {public: IntNode(int dataInit = 0, IntNode* nextLoc = nullptr); void InsertAfter(IntNode* nodeLoc); IntNode* GetNext(); void PrintNodeData(); int GetDataVal();private: int dataVal; IntNode* nextNodePtr;}; // ConstructorIntNode::IntNode(int dataInit, IntNode* nextLoc) { this->dataVal = dataInit; this->nextNodePtr = nextLoc;} /* Insert node after this node. * Before: this -- next * After: this -- node -- next */void IntNode::InsertAfter(IntNode* nodeLoc) { IntNode* tmpNext = nullptr; tmpNext = this->nextNodePtr; // Remember next this->nextNodePtr = nodeLoc; // this -- node -- ? nodeLoc->nextNodePtr = tmpNext; // this -- node -- next} // Print dataValvoid IntNode::PrintNodeData() { cout << this->dataVal << endl;} // Grab location pointed by nextNodePtrIntNode* IntNode::GetNext() { return this->nextNodePtr;} int IntNode::GetDataVal() {…arrow_forwardC++arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
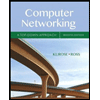
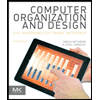
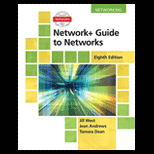
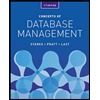
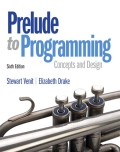
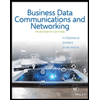