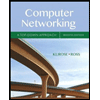
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
![Exercise (5): Strings
A. String Length method
public class MyClass {
public static void main(String[] args) {
String txt = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
System.out.println("The length of the txt string is: " + txt.length());
) }
B. Uppercase and Lowercase methods
public class MyClass {
public static void main(String[] args) {
String txt = "Hello World";
System.out.println(txt.toUpperCase ()); // Outputs "HELLO WORLD"
System.out.println(txt.toLowerCase ()); // Outputs "hello world"
}}
C. String concatenation
The + operator can be used between strings to combine them.
This is called concatenation.
You can also use the concat() method to concatenate two strings
public class MyClass {
public static void main(String[] args) {
String firstName = "Amani";
String fatherName = "Sabri";
+ fatherName);
System.out. println(firstName +
System.out.println(firstName.concat (fatherName)); }}
1. Computer Programming (1)
By: lect. Amani Abumansour](https://content.bartleby.com/qna-images/question/5114e966-abb6-4998-8183-95d5889adf14/0d5cd457-48c0-4de9-89a0-ccf02238a6ac/ci9nplc_thumbnail.jpeg)
Transcribed Image Text:Exercise (5): Strings
A. String Length method
public class MyClass {
public static void main(String[] args) {
String txt = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
System.out.println("The length of the txt string is: " + txt.length());
) }
B. Uppercase and Lowercase methods
public class MyClass {
public static void main(String[] args) {
String txt = "Hello World";
System.out.println(txt.toUpperCase ()); // Outputs "HELLO WORLD"
System.out.println(txt.toLowerCase ()); // Outputs "hello world"
}}
C. String concatenation
The + operator can be used between strings to combine them.
This is called concatenation.
You can also use the concat() method to concatenate two strings
public class MyClass {
public static void main(String[] args) {
String firstName = "Amani";
String fatherName = "Sabri";
+ fatherName);
System.out. println(firstName +
System.out.println(firstName.concat (fatherName)); }}
1. Computer Programming (1)
By: lect. Amani Abumansour
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 6 images

Knowledge Booster
Similar questions
- Given the previous Car class, the following members have been added for you: Private: string * parts; //string array of part names int num_parts; //number of parts Public: void setParts(int numpart, string newparts[]) //set the numparts and parts int getNumParts() string * getParts() string getPart(int index) //return the string in parts at index num Please implement the following for the Car class: Add the copy constructor. (deep copy!) Add the copy assignment operator. (deep copy!) Add the destructorarrow_forwardThe provided file has syntax and/or logical errors. Determine the problem and fix the program.arrow_forwardJavaarrow_forward
- Fibonacci A fibonacci sequence is a series of numbers in which each number is the sum of the two preceding numbers. For example: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on… For this, you will implement a class that takes a positive integer (n) and returns the number in the nth position in the sequence. Examples When n is 1, the returned value will be 0. When n is 4, the returned value will be 2. When n is 9, the returned value will be 21. come up with the formula and base cases Implementation Create a class Fibonacci with a public static method getValue. Create a Main class to test and run your Fibonacci class.arrow_forwardDon't delete the code. Fix the error and make sure it runs. #include using namespace std; class Puzzle { public: char arr[4][4] // constructor Puzzle() { char arr[4][2] = {'f', 'k', 's','s'} {'b', 'j', 'o','u'} {'b', 'o', 'b', 'n'} {'x', 'y', 'w', 'y'} } // destructor ~Puzzle() { } // print method void print() { for (int i=0; i> word; if (p.search(word)) cout << "The word is found in the puzzle." << endl; else cout << "The word is not found in the puzzle." << endl; return 0; }arrow_forwardQ: What's a better way to write this? (C#) public string SomeMethod(string OriginalZip) { string zip = "Zip Not Available"; zip = GetZip(zip, OriginalZip); return zip; } private static string GetZip(string zip, string OriginalZip) { int zipLength = (OriginalZip.HasValue) ? OriginalZip.Value.ToString().Length: 0; int NumOf Leading Zeroes = (zipLength > O && zipLength < 5) ? 5 - zipLength: 0; if (OriginalZip.HasValue) { i++) } zip = OriginalZip.Value.ToString(); for (int i = 0; i < NumOf Leading Zeroes; { } zip = "0" + zip; } return zip;arrow_forward
- Practice / Frequency of Characters Write a method that returns the frequency of each characters of a given String parameter. If the given String is null, then return null If the given String is empty return an empty map Example input: output: empty map explanation: input is empty Example input: null output: null explanation: input is null. Since problem output is focused on the frequency we can comfortably use Map data structure. Because we can use characters as key and the occurrences of them as value. Example input: responsible output: {r=1, e=2, s=2, p=1, o=1, n=1, i=1, b=1, l=1} explanation: characters are keys and oc values ences arearrow_forwardEnhanced selection sort algorithm SelectionSortDemo.java package chapter7; /** This program demonstrates the selectionSort method in the ArrayTools class. */ public class SelectionSortDemo { public static void main(String[] arg) { int[] values = {5, 7, 2, 8, 9, 1}; // Display the unsorted array. System.out.println("The unsorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); // Sort the array. selectionSort(values); // Display the sorted array. System.out.println("The sorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); } /** The selectionSort method performs a selection sort on an int array. The array is sorted in ascending order. @param array The array to sort. */ public static void selectionSort(int[] array) { int startScan, index, minIndex, minValue; for (startScan = 0; startScan < (array.length-1); startScan++) { minIndex = startScan; minValue…arrow_forwardusing System;class TicTacToe{ static void Print(char[] board) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); } static void Main(string[] args) { char[] board = new char[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); }} Hello! This program is in C# could a line of code be added to ask the user if they want to be "X" or if they want to be "O"?arrow_forward
- using System; class TicTacToe { staticint player = 1; staticint choice; staticvoid Print( char[] board); staticvoid Main(string[] args) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); } staticvoid Main(string[] args) { char[] board = newchar[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; if( board[i]== 'O') Console.Write("It is your turn to place an X"); } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); } } Hello! I need help with getting my code to compile. It's written in C# and i'm a beginner. it's giving me two errors and i'm not sure how to fix them. Please don't copy paste an answer from google.arrow_forwardpublic class Program7 { publicstaticvoidmain(String[]args){ int[]numbers=newint[100]; String[]names={"Maria","Aris","Galin","Galena"}; double[]balances=newdouble[]{2,56,12.57,36.57,57.89}; //length System.out.println(numbers.length); System.out.println(names.length); System.out.println(balances.length); //modifying names[3]="Celine Dion"; System.out.println("__________________________"); //looping for(inti=0;i<names.length;i++){ System.out.println(names[i]); } System.out.println("__________________________"); for(Stringname:names){ System.out.println(name); } //modifying array System.out.println("__________________________"); for(inti=0;i<names.length;i++){ names[i]=names[i]+" Annan"; System.out.println(names[i]); } System.out.println("__________________________"); doubletotal=0.0; for(inti=0;i<balances.length;i++){ total+=balances[i]; } System.out.println("Total Balance: "+ total); } } //calculate the average of the total balancearrow_forwardclass Container {private int val1=1;private void printValue(){final int val2=2;class Containee{public void f(){System.out.println(String.format("Values are: %d and %d."),val1);}}}public static void main(String[] args) {(new Container()).printValue();}} a.The above does not compile because the method f() requires an instance of class Container b.The above does not compile because the class definition in done within the body of the function c.The above code complies with no errors, but does not produce any ouput d.The above code does not compile because the methodf() cannot access the variable val1arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
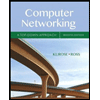
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
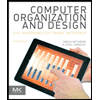
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
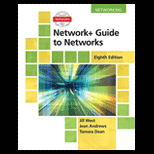
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
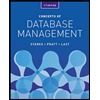
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
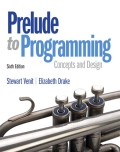
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
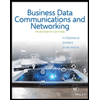
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY