Exercise PrintTriangles: Write a method to print each of the following patterns using nested-loops in a class called PrintTriangles. The program shall prompt user for the numRows. The signatures of the methods are: public static void printXxxTriangle(int numRows) // Xxx is the pattern's name 1 1 2 1 1 2 4 2 1 1 2 4 8 4 2 1 1 2 4 8 16 8 4 2 1 1 2 4 8 16 32 16 8 4 2 1 1 2 4 8 16 32 64 32 16 8 4 2 1 1 2 4 8 16 32 64 128 64 32 16 8 4 2 1 (a) PowerOf2Triangle
Exercise PrintTriangles: Write a method to print each of the following patterns using nested-loops in a class called PrintTriangles. The program shall prompt user for the numRows. The signatures of the methods are:
public static void printXxxTriangle(int numRows) // Xxx is the pattern's name
1
1 2 1
1 2 4 2 1
1 2 4 8 4 2 1
1 2 4 8 16 8 4 2 1
1 2 4 8 16 32 16 8 4 2 1
1 2 4 8 16 32 64 32 16 8 4 2 1
1 2 4 8 16 32 64 128 64 32 16 8 4 2 1
(a) PowerOf2Triangle
1 1
1 1 1 1
1 2 1 1 2 1
1 3 3 1 1 3 3 1
1 4 6 4 1 1 4 6 4 1
1 5 10 10 5 1 1 5 10 10 5 1
1 6 15 20 15 6 1 1 6 15 20 15 6 1
(b) PascalTriangle1 (c) PascalTriangle2
Exercise TrigonometricSeries: Write a method to compute sin(x) and cos(x) using the following series expansion, in a class called TrigonometricSeries. The signatures of the methods are:
public static double sin(double x, int numTerms) // x in radians
public static double cos(double x, int numTerms)
Compare the values computed using the series with the JDK methods Math.sin(), Math.cos() at x=0, π/6, π/4, π/3, π/2 using various numbers of terms.
Hints: Do not use int to compute the factorial; as factorial of 13 is outside the int range. Avoid generating large numerator and denominator. Use double to compute the terms as:
Exercise Exponential Series: Write a method to compute e and exp(x) using the following series expansion, in a class called TrigonometricSeries. The signatures of the methods are:
public static double exp(int numTerms) // x in radians
public static double exp(double x, int numTerms)
Exercise SpecialSeries: Write a method to compute the sum of the series in a class called SpecialSeries. The signature of the method is:
public static double sumOfSeries(double x, int numTerms)
Exercise FibonacciInt (Overflow) : Write a program called FibonacciInt to list all the Fibonacci numbers, which can be expressed as an int (i.e., 32-bit signed integer in the range of [-2147483648, 2147483647]). The output shall look like:
F(0) = 1
F(1) = 1
F(2) = 2
...
F(45) = 1836311903
F(46) is out of the range of int
Hints: The maximum and minimum values of a 32-bit int are kept in constants Integer.MAX_VALUE and Integer.MIN_VALUE, respectively. Try these statements:
System.out.println(Integer.MAX_VALUE);
System.out.println(Integer.MIN_VALUE);
System.out.println(Integer.MAX_VALUE + 1);
Take note that in the third statement, Java Runtime does not flag out an overflow error, but silently wraps the number around. Hence, you cannot use F(n-1) + F(n-2) > Integer.MAX_VALUE to check for overflow. Instead, overflow occurs for F(n) if (Integer.MAX_VALUE – F(n-1)) < F(n-2) (i.e., no room for the next Fibonacci number).
Write a similar program for Tribonacci numbers.
Exercise FactorialInt (Overflow): Write a program called Factorial1to10, to compute the factorial of n, for 1≤n≤10. Your output shall look like:
The factorial of 1 is 1
The factorial of 2 is 2
...
The factorial of 10 is 3628800
Modify your program (called FactorialInt), to list all the factorials, that can be expressed as an int (i.e., 32-bit signed integer in the range of [-2147483648, 2147483647]). Your output shall look like:
The factorial of 1 is 1
The factorial of 2 is 2
...
The factorial of 12 is 479001600
The factorial of 13 is out of range
Hints: The maximum and minimum values of a 32-bit int are kept in constants Integer.MAX_VALUE and Integer.MIN_VALUE, respectively. Try these statements:
System.out.println(Integer.MAX_VALUE);
System.out.println(Integer.MIN_VALUE);
System.out.println(Integer.MAX_VALUE + 1);
Take note that in the third statement, Java Runtime does not flag out an overflow error, but silently wraps the number around.
Hence, you cannot use F(n) * (n+1) > Integer.MAX_VALUE to check for overflow. Instead, overflow occurs for F(n+1) if (Integer.MAX_VALUE / Factorial(n)) < (n+1), i.e., no room for the next number.
Try: Modify your program again (called FactorialLong) to list all the factorial that can be expressed as a long (64-bit signed integer). The maximum value for long is kept in a constant called Long.MAX_VALUE.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

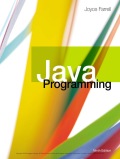
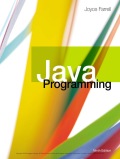