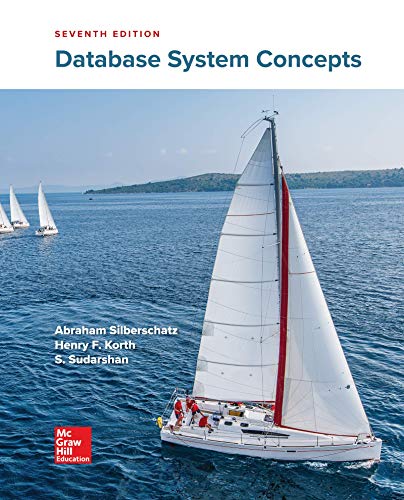
Concept explainers
Java:
Write a complete method to create an array of random integers.
Method Specification
The method takes in three integer parameters: the array size, the lower bound, and the upper bound. The method also takes in a boolean parameter.
The method creates and returns an integer array of the specified size that contains random numbers between the lower and upper bound., using these rules:
- The lower bound is always inclusive.
- If the boolean parameter is true, the upper bound is also inclusive.
- If the boolean parameter is false, the upper bound is not inclusive (meaning it is exclusive).
If any of the parameters are invalid, null is returned.
Driver/Tester Program
I have provided you with a driver/tester program that you can use to test your code before submitting. Paste your code into the placeholder at the top of the file.
I strongly recommend running this program before you submit your homework. The program is designed to help you catch common errors and fix them before submitting. Run the program multiple times since random number selection is involved. Each time, review the output to see if your actual results match what was expected. A "**********TEST FAILED" message should also print if a test case fails.
The driver program has some tester methods at the end. These method are used to streamline the running of multiple repeated tests. You don't need to understand, review, or modify these methods. The driver program was too long to paste so it is in two pictures. See attached images for the driver program.
![}
/* TESTER METHODS */
* The methods below are designed to help support the tests cases run from main. You don't
* need to use, modify, or understand these methods. You can safely ignore them. :)
public static void testGenerateRandomArrayWith InvalidParameters(int size, int low, int high, boolean inclusive, String testDescription) {
int[] array
generateRandomArray (size, low, high, inclusive);
System.out.println("\nExpected size of returned array: " + size);
String inclusiveString = inclusive? "] (inclusive)" : ") (EXCLUSIVE)";
System.out.println("Expected Range of values: (inclusive) [" + low + ",
if(array!=null) {
}
} else {
}
}
public static void testGenerateRandomArray(int size, int low, int high, boolean inclusive) {
int[] array
generateRandomArray (size, low, high, inclusive);
System.out.println("\nExpected size: " + size);
System.out.println(" Actual size: " + array.length);
if(size==0) {
+ high + inclusiveString);
System.out.println("**********TEST FAILED for invalid parameters. Returned array should be null. Test: " + test Description);
allTests Passed = false;
System.out.println("Expected result: null\n Actual result: " + array);
if(array!=null && array.length>0) {
allTests Passed = false;
System.out.print("*
}
return;
}
String inclusiveString = inclusive? "] (inclusive)" : ") (EXCLUSIVE)";
System.out.println("Expected Range: (inclusive) [" + low + ", ..., " + high + inclusiveString);
System.out.println("Actual Array: " + Arrays.toString(array));
if(array.length != size) {
}
}
}
boolean validNumbers = true;
int badNumber = -1;
for(int num: array) {
if (num < low) {
allTests Passed = false;
System.out.println("**********TEST FAILED: array is not the requested size");
validNumbers = false;
badNumber = num;
***TEST FAILED for requested size of 0. ");
if(inclusive && num > high) {
validNumbers = false;
}
if(!validNumbers) {
*/
badNumber = num;
} else if(!inclusive && num >= high) {
validNumbers = false;
badNumber = num;
allTestsPassed = false;
System.out.println("**********TEST FAILED: one or more numbers is outside of the range. One number outside the range is " + badNumber);](https://content.bartleby.com/qna-images/question/7024c4db-e4c4-44c2-8fab-3045baf15ef1/0c6158ba-c928-4a0d-9895-dfe1f40e432d/vz2595b_thumbnail.png)
![import java.util.*;
public class Homework5BDriver {
public static int[] generateRandomArray (int size, int lower, int upper, boolean inclusive) {
// YOUR CODE HERE
return new int[] {}; // placeholder: replace with your own code when you write the method
}
public static void main(String[] args) {
System.out.println(".
System.out.println("
-TESTING generateRandomArray METHOD-
-");
*IMPORTANT NOTE: run these tests multiple times because they involve random numbers. \nThis will increase your chance of finding any bugs.");
// the parameters sent to the tester method are the same as the ones sent to the generateRandomArray method
// parameter 1: the size of the array
// parameter 2: the lower bound
// parameter 3: the upper bound
// parameter 4: whether or not the upper bound is inclusive
testGenerateRandomArray (20, 1, 10, true);
testGenerateRandomArray (20, -10, 0, true);
testGenerateRandomArray (8, -1, 1, true);
testGenerateRandomArray(100, 1, 10, false);
testGenerateRandomArray(16, 1, 5, true);
testGenerateRandomArray (15, 1, 5, false);
testGenerateRandomArray(18, 4, 5, false);
testGenerateRandomArray (12, 7, 7, true);
System.out.println("\n----
---TESTS WITH INVALID PARAMETERS---
----");
testGenerateRandomArrayWith Invalid Parameters (10, 4, 3, true, "lower bound is greater than upper bound");
testGenerateRandomArrayWithInvalid Parameters (10, 4, 4, false, "lower bound and upper bound are equal but the results should NOT be exlusive");
testGenerateRandomArrayWithInvalidParameters (10, 4, 2, false, "lower bound is greater than upper bound");
testGenerateRandomArrayWith Invalid Parameters(-1, 4, 14, true, "negative array size");
if (allTests Passed) {
} else {
}
System.out.println("\n----------Summary-
System.out.flush();
System.err.println("\n**********Summary*
}
private static boolean allTests Passed = true;
\nAll automated tests have passed. \nManually review your code for style. \nManually view the expected output to make sure it matches.");
ERROR: There is failure in at least one automated test. Review the output above for details.");](https://content.bartleby.com/qna-images/question/7024c4db-e4c4-44c2-8fab-3045baf15ef1/0c6158ba-c928-4a0d-9895-dfe1f40e432d/litqyx8_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- JavaDriver Program Write a driver program that contains a main method and two other methods. Create an Array of Objects In the main method, create an array of Food that holds at least two foods and at least two vegetables. You can decide what data to use. Method: Print Write a method that prints the text representation of the foods in the array in reverse order (meaning from the end of the array to the beginning). Invoke this method from main. Method: Highest Sugar Food Write a method that takes an array of Food objects and finds the Food that has the most sugar. Note that the method is finding the Food, not the grams of sugar. Hint: in looping to find the food, keep track of both the current highest index and value. Invoke this method from main.arrow_forwardprogramming used: javaarrow_forwardAg 1- Random Prime Generator Add a new method to the Primes class called genRandPrime. It should take as input two int values: 1owerBound and upperBound. It should return a random prime number in between TowerBound (inclusive) and upperBound (exclusive) values. Test the functionality inside the main method. Implementation steps: a) Start by adding the method header. Remember to start with public static keywords, then the return type, method name, formal parameter list in parenthesis, and open brace. The return type will be int. We will have two formal parameters, so separate those by a comma. b) Now, add the method body code that generates a random prime number in the specified range. The simplest way to do this is to just keep trying different random numbers in the range, until we get one that is a prime. So, generate a random int using: int randNum = lowerBound + gen.nextInt(upperBound); Then enter put a while loop that will keep going while randNum is not a prime number - you can…arrow_forward
- Ajva.arrow_forwardProblem 1: Complete the sumOfDiagonals method in SumOfDiagonals.java to do the following: The method takes a 2D String array x as a parameter and returns no value. The method should calculate and print the sum of the elements on the major diagonal of the array x. In order to have a major diagonal, the array passed into the method should be a square (n-by-n), if it's not a square your program should handle that situation by throwing an exception. (Do Not worry about ragged arrays) If the array is a square, but there is a non-integer value on the major diagonal, your program should handle that situation by throwing an exception. When handling the exceptions, be as specific as you can be, (i.e. Do Not just use the Exception class to handle all exceptions in one catch block). Make the proper calls to the sumOfDiagonals method from the main method to test your sumOfDiagonals method on all the String arrays provided in the main method.arrow_forward1) Write a method that returns the range of an array of integers. The range is the difference between the highest and lowest values in the array. The signature of the method should be as follows. public static int computeRange(int[] values)arrow_forward
- JAVA PROGRAM Chapter 7. PC #1. Rainfall Class Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: • the total rainfall for the year • the average monthly rainfall • the month with the most rain • the month with the least rain Demonstrate the class in a complete program. Main class name: RainFall (no package name) est Case 1 Enter the rainfall amount for month 1:\n1.2ENTEREnter the rainfall amount for month 2:\n2.3ENTEREnter the rainfall amount for month 3:\n3.4ENTEREnter the rainfall amount for month 4:\n5.1ENTEREnter the rainfall amount for month 5:\n1.7ENTEREnter the rainfall amount for month 6:\n6.5ENTEREnter the rainfall amount for month 7:\n2.5ENTEREnter the rainfall amount for month 8:\n3.3ENTEREnter the rainfall amount for month 9:\n1.1ENTEREnter the rainfall amount for month 10:\n5.5ENTEREnter the rainfall amount for month 11:\n6.6ENTEREnter…arrow_forward1Code a JavaScript callback function for the Array.map method to ('Yu Yamaguchi') evaluate an array of integersidentify the integers that are Prime numbersreturn the Prime numbers in the new array 2 Code a JavaScript callback function for the Array.reduce method to evaluate an array of integersdetermine the smalles integerreturn the smalles integer Note: if there are identical integers, the function is to return the last one.arrow_forwardWrite java code that recursively calculates the Sum of the array cells. Program requirements: Create an array named MyArr and initialize it as follow: 19 27 81 243 88 1- Write a method named ArrSum that recursively calculates the Sum of the numbers in the Array and returns the Sum of the numbers. Hint: Method with 1 parameter (the Array) that returns 1 value (the Array's Sum). 2- Write a method named Display that displays the Array contents and Sum of the numbers. Hint: Method with 2 parameters (the Array) and Sum with no returns (void) 3- Your program output format must be the same as the following: =>> Array Summation Array: 31 19 27 81 243 88 100 6 10 5 Sum: 610 =>> End of the Program Documentation / Comments 31 100 6 10 5arrow_forward
- T/F The body of a method may be emptyarrow_forwardHow is this program written in java?arrow_forwardUsing jGRASP please de-bug this /**This program demonstrates an array of String objects.It should print out the days and the number of hours spent at work each day.It should print out the day of the week with the most hours workedIt should print out the average number of hours worked each dayThis is what should display when the program runs as it shouldSunday has 12 hours worked.Monday has 9 hours worked.Tuesday has 8 hours worked.Wednesday has 13 hours worked.Thursday has 6 hours worked.Friday has 4 hours worked.Saturday has 0 hours worked.Highest Day is Wednesday with 13 hours workedAverage hours worked in the week is 7.43 hours*/{public class WorkDays{public static void main(String[] args){String[] days = { "Sunday", "Monday", "Tuesday","Wednesday", "Thursday", "Friday", "Saturday"}; int[] hours = { 12, 9, 8, 13, 6, 4}; int average = 0.0;int highest = 0;String highestDay = " ";int sum = 0; for (int index = 0; index < days.length; index++){System.out.println(days[index] + " has "…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
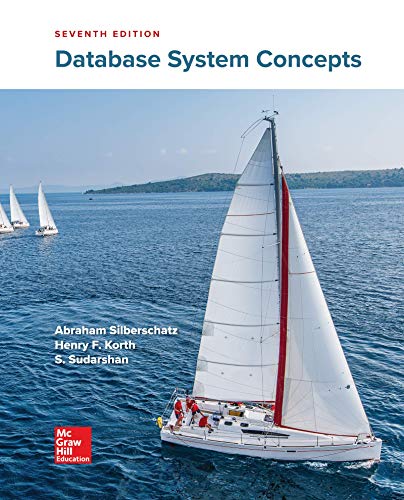
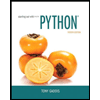
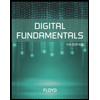
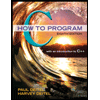
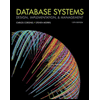
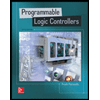