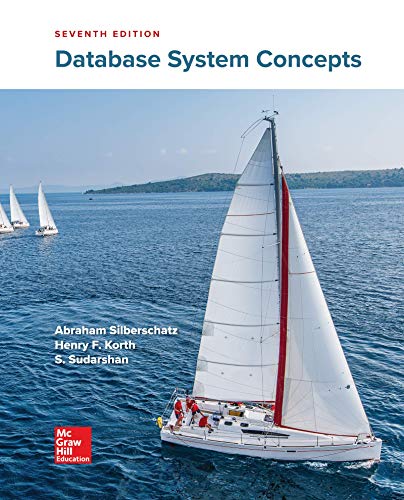
java
Exercise1:
Write a program that takes three integer numbers from the console as inputs. For each number, the program should call a static method named isPositive() to determine if a number is positive, negative, or equal to zero.
Exercise 3:
The following code asks a user to enter her/his age then determines if a user is an adult or not. Trace the code, to find and solve the syntax errors. You need to identify the errors and rewrite the correct code.
import java.util.Scanner;
public class Ex3 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("Enter your age:");
int age = input.nextInt();
double flag = isAdult(double age);
if(flag) {
System.out.println("You are an adult.");
} else {
System.out.println("You are not an adult.");
}
}
public boolean isAdult(int age) {
if(age > 18) {
return true;
} else {
return false;
}
}
}
Exercise 4:
Write a program to do the following using the Math class:
- Ask a user to enter two integer numbers
- Finds the square root of each number
- Finds the minimum
- Finds the maximum
Exercise 5:
Write a program to calculate area of circle. The main method should pass the radius of the circle to a static method named calculateArea(). The program should display the calculated area inside the main method.

Step by stepSolved in 2 steps with 1 images

- java-Find the area of circle using method... Write an application that prompts the user for the radius of a circle and uses a method called circleArea to find tarea of the circle. method method should return the computed area to the caller method.arrow_forwardWrite the following two methods: computeDiameter: This method accepts the radius (r) of a circle, and returns its diameter (2*r; radius = 7.5). Declare all variables used here & initialize radius: Show method call: Write method code here: displayData: This method accepts two arguments: radius & diameter. The output should have appropriate messages on the screen. Show method call: Write method code here:arrow_forwarda. Plan the logic for an insurance company program to determine policy premiums. The program continuously prompts the user for an insurance policy number. When the user enters an appropriate sentinel value, end the program. Call a method that prompts each user for the type of policy needed—health or auto. While the user’s response does not indicate health or auto, continue to prompt the user. When the value is valid, return it from the method. Pass the user’s response to a new method where the premium is set and returned—$550 for a health policy or $225 for an auto policy. Display the results for each policy. b.Modify Exercise 9a so that the premium-setting method calls one of two additional methods—one that determines the health premium or one that determines the auto premium. The health insurance method asks users whether they smoke; the premium is $550 for smokers and $345 for nonsmokers. The auto insurance method asks users to enter the number of traffic tickets they have received…arrow_forward
- Financial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forwardbasic java please Write a method called findTip that finds the value of a meal as a double after adding the tip to the cost. Then return this value to the calling function. For example findTip(120.10, 5) would 126.105 because that is a 5% tip. While findTip(48.0, 15) would return 55.2 because that is a 15% tip. Note that the bill is a double while the tip is an int. Don’t worry about rounding to two decimal places, just return the full value as a double.arrow_forwardJava programming The US dollar = 0.82 Euros and 1.21 Canadian dollars.Write the following methods to convert US dollars to Euros and to Canadiandollars: *** A method prompting the user to select an option (to convert to Euros orto Canadian dollars) and returns the option to the main method.arrow_forward
- Program63.javaWrite a program that estimates the cost of carpet for one or more rooms with rectangular floors. Begin by prompting for the price of carpet per square yard and the number of rooms needing this carpet. Use a loop to prompt for the floor dimensions of each room in feet. In this loop, call a value-returning method with the dimensions and carpet price as arguments. The method should return the carpet cost for each room to main, where it will be printed and accumulated. After all rooms have been processed, the program should display the total cost of the job.Sample Output (image below) Program64.javaWrite a program that demonstrates method overloading by defining and calling methods that return the area of a triangle, a rectangle, or a square.arrow_forwardjava programming: pleas see attachment as well***** In this task, you are asked to write a program called Swap.java, including at least 3 following methods: - Main() swapDigitPairs ( parameter) - swapLetterPairs (parameter) In the main method, - Use your Panther Number as the input argument, call swapDigitPairs ( parameter) - Ask user to input a number and call a method swapDigitPairs to swap the number as shown in the example of the following figure. - Ask the user to input a string of letters/numbers and call a method swapLetterPairs to swap the letters as shown in the following example. - swapDigitPairs ( parameter) - swapLetterPairs (parameter) In the main method, - Use your Panther Number as the input argument, call swapDigitPairs ( parameter) - Ask user to input a number and call a method swapDigitPairs to swap the number as shown in the example of the following figure. - Ask the user to input a string of letters/numbers and call a method swapLetterPairs to swap the letters…arrow_forwardNote:- Please type and execute this java program and also need an output for this program.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
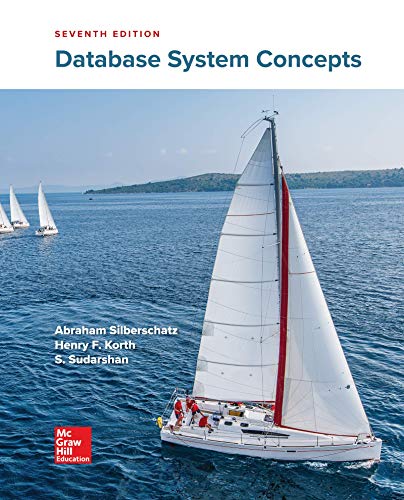
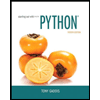
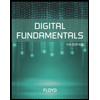
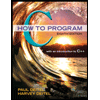
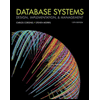
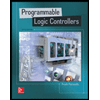