Explain the code and how it works:
Explain the code and how it works:
#include <iostream>
#include <iomanip>
#include <string>
#include <
using namespace std;
class Song
{
private:
string songtitle = "";
string artist = "";
int year = 0;
public:
void set_song_title(string songtitle_param);
void set_artist_name(string songartist_param);
string get_song_title() const; // "const" safeguards class variable changes within function
string get_artist_name()const;
string get_song_title_upper() const;
string get_artist_name_upper() const;
void set_year(int year_param);
int get_year() const;
}; // NOTICE: Class declaration ends with semicolon!
void Song::set_song_title(string songtitle_param)
{
songtitle = songtitle_param;
}
void Song::set_artist_name(string songartist_param)
{
artist = songartist_param;
}
string Song::get_song_title() const
{
return songtitle;
}
string Song::get_artist_name() const
{
return artist;
}
string Song::get_song_title_upper() const
{
string title_upper;
for (char c : songtitle) {
title_upper.push_back(toupper(c));
}
return title_upper;
}
string Song::get_artist_name_upper() const
{
string song_upper;
for (char c : artist) {
song_upper.push_back(toupper(c));
}
return song_upper;
}
void Song::set_year(int year_param)
{
year = year_param;
}
int Song::get_year() const
{
return year;
}
int main()
{
cout << "The Song List program\n\n"
<< "Enter a song...\n\n";
// get vector of song objects
vector<Song> songs;
char another = 'y';
while (tolower(another) == 'y')
{
Song song;
string songtitle;
string artist;
cout << "Title: ";
getline(cin, songtitle);
song.set_song_title(songtitle);
cout << "Artist: ";
getline(cin, artist);
int year;
cout << "Year: ";
cin >> year;
song.set_year(year);
songs.push_back(song);
cout << "\nEnter another song? (y/n): ";
cin >> another;
cin.ignore();
cout << endl;
}
// display the songs
const int w = 10;
cout << left
<< setw(w * 3) << "TITLE"
<< setw(w) << "YEAR" << endl;
for (Song song : songs)
{
cout << setw(w * 3) << song.get_song_title()
<< setw(w*3) << song.get_artist_name()
<< setw(w * 3) << song.get_year() << endl;
}
cout << endl;
cout << endl;
// Output with titles in ALL CAPS
for (Song song : songs)
{
cout << setw(w * 3) << song.get_song_title_upper()
<< setw(w*3) << song.get_artist_name_upper()
<< setw(w * 3) << song.get_year() << endl;
}
}

//Including the required headerfiles
#include <iostream>
#include <iomanip>
#include <string>
#include <vector>
// using the standard namespace
using namespace std;
//create a class named song
class Song
{
//declaring private data fileds
private:
string songtitle = "";
string artist = "";
int year = 0;
//declaring public member function
public:
void set_song_title(string songtitle_param);
void set_artist_name(string songartist_param);
string get_song_title() const; // "const" safeguards class variable changes within function
string get_artist_name()const;
string get_song_title_upper() const;
string get_artist_name_upper() const;
void set_year(int year_param);
int get_year() const;
}; // NOTICE: Class declaration ends with semicolon!
// writting the definition of all the member function of class song
//these we call getters and setters
void Song::set_song_title(string songtitle_param)
{
songtitle = songtitle_param;
}
void Song::set_artist_name(string songartist_param)
{
artist = songartist_param;
}
string Song::get_song_title() const
{
return songtitle;
}
string Song::get_artist_name() const
{
return artist;
}
string Song::get_song_title_upper() const
{
string title_upper;
for (char c : songtitle) {
title_upper.push_back(toupper(c));
}
return title_upper;
}
string Song::get_artist_name_upper() const
{
string song_upper;
for (char c : artist) {
song_upper.push_back(toupper(c));
}
return song_upper;
}void Song::set_year(int year_param)
{
year = year_param;
}
int Song::get_year() const
{
return year;
}
//main function or driver program to test the working of song class
int main()
{
cout << "The Song List program\n\n"
<< "Enter a song...\n\n";
// get vector of song objects
//create vector of song class with songs name
vector<Song> songs;
char another = 'y';
//while our input is y
while (tolower(another) == 'y')
{
Song song;
string songtitle;
string artist;
cout << "Title: ";
getline(cin, songtitle);
song.set_song_title(songtitle);
cout << "Artist: ";
getline(cin, artist);
int year;
cout << "Year: ";
cin >> year;
song.set_year(year);
songs.push_back(song);
cout << "\nEnter another song? (y/n): ";
cin >> another;
cin.ignore();
cout << endl;
}
//if we do not press y
// display the songs
const int w = 10;
cout << left
<< setw(w * 3) << "TITLE"
<< setw(w) << "YEAR" << endl;
for (Song song : songs)
{
cout << setw(w * 3) << song.get_song_title()
<< setw(w*3) << song.get_artist_name()
<< setw(w * 3) << song.get_year() << endl;
}
cout << endl;
cout << endl;
// Output with titles in ALL CAPS
for (Song song : songs)
{
cout << setw(w * 3) << song.get_song_title_upper()
<< setw(w*3) << song.get_artist_name_upper()
<< setw(w * 3) << song.get_year() << endl;
}
}
Step by step
Solved in 2 steps

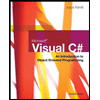
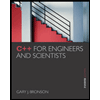
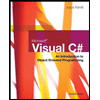
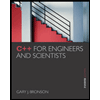