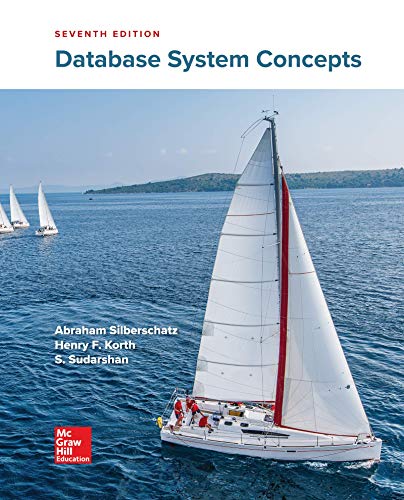
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Explain the following code :
public static void printLeafNodes(TreeNode node) {
// base case
if (node == null) {
return;
}
if (node.left == null && node.right == null) {
System.out.printf("%d ", node.value);
}
printLeafNodes(node.left);
printLeafNodes(node.right);
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Lab Goal : This lab was designed to teach you more about recursion. Lab Description : luckyThrees will return a count of the 3s in the number unless the 3 is at the start. A 3 at the start of the number does not count. /* luckyThrees will return the count of 3s in the number* unless the 3 is at the front and then it does not count* 3 would return 0* 31332 would return 2* 134523 would return 2* 3113 would return 1* 13331 would return 3* 777337777 would return 2* the solution to this problem must use recursion*/public static int luckyThrees( long number ){} Sample Data : 331332134523311313331777337777 Sample Output : 022132arrow_forwardvoid listEmployees (void) { for (int i=0; i 10000. Make a guess about why the comparison function takes 2 struct Employee parameters (as opposed to struct Employee *) **arrow_forward#ifndef LLCP_INT_H#define LLCP_INT_H #include <iostream> struct Node{ int data; Node *link;};void DelOddCopEven(Node*& headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0); // prototype of DelOddCopEven of Assignment 5 Part 1 #endifarrow_forward
- Given the following C code: struct Node int data; struct Node struct Node next; prev; struct Node head; void funx ( ) if (head==NULL) return; struct Node current=head; while (current->next!=NULL) current=current-->next; while (current!=NULL) printf("&d ", current->data; current=current->prev; What is the functionality of this function? a) Print the contents of linked list b) Print the contents of linked list in reverse order c) Nonearrow_forwarddef is_balanced(root): return __is_balanced_recursive(root) def __is_balanced_recursive(root): """ O(N) solution """ return -1 != __get_depth(root) def __get_depth(root): """ return 0 if unbalanced else depth + 1 """ if root is None: return 0 left = __get_depth(root.left) right = __get_depth(root.right) if abs(left-right) > 1 or -1 in [left, right]: return -1 return 1 + max(left, right) # def is_balanced(root):# """# O(N^2) solution# """# left = max_height(root.left)# right = max_height(root.right)# return abs(left-right) <= 1 and is_balanced(root.left) and# is_balanced(root.right) # def max_height(root):# if root is None:.arrow_forward#include <iostream> usingnamespace std; class Queue { int size; int* queue; public: Queue(){ size = 0; queue = new int[100]; } void add(int data){ queue[size]= data; size++; } void remove(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } else{ for(int i =0; i < size -1; i++){ queue[i]= queue[i +1]; } size--; } } void print(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } for(int i =0; i < size; i++){ cout<<queue[i]<<" <- "; } cout << endl; } //your code goes here }; int main(){ Queue q1; q1.add(42); q1.add(2); q1.add(8); q1.add(1); Queue q2; q2.add(3); q2.add(66); q2.add(128); q2.add(5); Queue q3 = q1+q2; q3.print();…arrow_forward
- #include <iostream>#include <cstdlib>using namespace std; class IntNode {public: IntNode(int dataInit = 0, IntNode* nextLoc = nullptr); void InsertAfter(IntNode* nodeLoc); IntNode* GetNext(); void PrintNodeData(); int GetDataVal();private: int dataVal; IntNode* nextNodePtr;}; // ConstructorIntNode::IntNode(int dataInit, IntNode* nextLoc) { this->dataVal = dataInit; this->nextNodePtr = nextLoc;} /* Insert node after this node. * Before: this -- next * After: this -- node -- next */void IntNode::InsertAfter(IntNode* nodeLoc) { IntNode* tmpNext = nullptr; tmpNext = this->nextNodePtr; // Remember next this->nextNodePtr = nodeLoc; // this -- node -- ? nodeLoc->nextNodePtr = tmpNext; // this -- node -- next} // Print dataValvoid IntNode::PrintNodeData() { cout << this->dataVal << endl;} // Grab location pointed by nextNodePtrIntNode* IntNode::GetNext() { return this->nextNodePtr;} int IntNode::GetDataVal() {…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardWhat happens when a programmer attempts to access a node's data fields when the node variable refers to None? How do you guard against it? *PYTHONarrow_forward
- The numbers on the left margin denote line numbers. line#01 class LL_node {02 int val;03 LL_node next;04 public LL_node (int n) {05 val = n;06 next = null;07 }08 public void set_next (LL_node nextNode) {09 next = nextNode;10 }11 public LL_node get_next () {12 return next;13 }14 public void set_value (int input) {15 val = input;16 }17 public int get_value () {18 return val;19 }20 }21 public class LL {22 protected LL_node head;23 protected LL_node tail;24 public LL () {25 head = null;26 tail = null;27 }28 public int append (int n) {29 if (head == null) {30 head = new LL_node(n);31 tail = head;32 } else {33 LL_node new_node;34 new_node = new LL_node(n);35 tail.set_next (new_node);36 tail = new_node;37 }38 return n;39 }40 } which the following statement…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
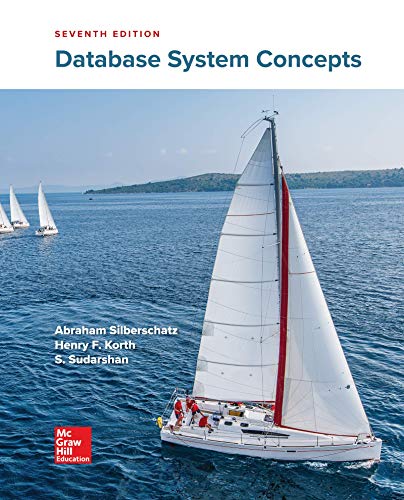
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
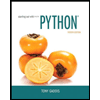
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
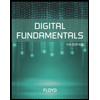
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
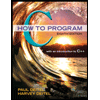
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
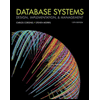
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
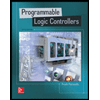
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education