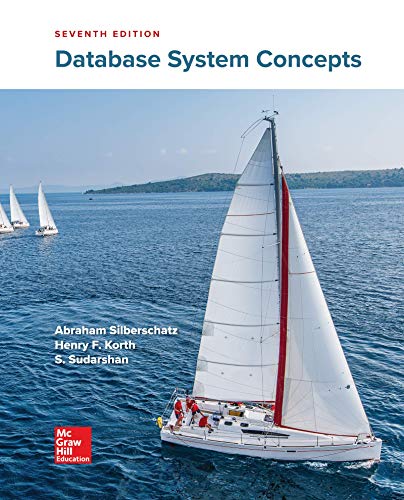
C++ PROGRAM: Please complete my program
Implement the 4 functions:
bool search(int num), bool insert(int num) , bool remove(int num), bool isEmpty
bstree.h
#include "tree.h"
#include <iostream>
using namespace std;
class BSTree {
BTree* tree;
public:
BSTree() {
tree = new BTree();
}
////////////////////////////////////////////////
bool search(int num) {
}
////////////////////////////////////////////////
bool insert(int num) {
// TODO insert
}
////////////////////////////////////////////////
bool remove(int num) {
// TODO remove
}
///////////////////////////////////////////////
// WARNING. Do not modify this method.
void print() {
if (isEmpty()) {
cout << "EMPTY";
return;
}
cout << "PRE-ORDER: ";
print_preorder(tree->getRoot());
cout << endl << "IN-ORDER: ";
print_inorder(tree->getRoot());
cout << endl << "POST-ORDER: ";
print_postorder(tree->getRoot());
cout << endl << "STATUS: " << check_health(tree->getRoot(), NULL);
}
/////////////////////////////////////////////////////
bool isEmpty() {
// TODO isEmpty
}
/////////////////////////////////////////////////////
void print_preorder(node* curr) {
// TODO preorder traversal
cout << curr->element << " ";
if(curr->left != NULL){
print_preorder(curr->left);
}
if(curr->right != NULL){
print_preorder(curr->right);
}
}
// }
/////////////////////////////////////////////////////
// WARNING. Do not modify this method.
void print_inorder(node* curr) {
if (curr->left != NULL) {
print_inorder(curr->left);
}
cout << curr->element << " ";
if (curr->right != NULL) {
print_inorder(curr->right);
}
}
////////////////////////////////////////////////////
void print_postorder(node* curr) {
// TODO postorder traversal
if(curr->left != NULL){
print_postorder(curr->left);
}
if(curr->right != NULL){
print_postorder(curr->right);
}
cout << curr->element << " ";
}
// }
////////////////////////////////////////////////////
// WARNING. Do not modify this method.
bool check_health(node* curr, node* parent) {
return tree->check_health(curr, parent);
}
};

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- given code lab4 #include <stdio.h>#include <stdlib.h> /* typical C boolean set-up */#define TRUE 1#define FALSE 0 typedef struct StackStruct{int* darr; /* pointer to dynamic array */int size; /* amount of space allocated */int inUse; /* top of stack indicator - counts how many values are on the stack */} Stack; void init (Stack* s){s->size = 2;s->darr = (int*) malloc ( sizeof (int) * s->size );s->inUse = 0;} void push (Stack* s, int val){/* QUESTION 7 *//* check if enough space currently on stack and grow if needed */ /* add val onto stack */s->darr[s->inUse] = val;s->inUse = s->inUse + 1;} int isEmpty (Stack* s){if ( s->inUse == 0)return TRUE;elsereturn FALSE;} int top (Stack* s){return ( s->darr[s->inUse-1] );} /* QUESTION 9.1 */void pop (Stack* s){if (isEmpty(s) == FALSE)s->inUse = s->inUse - 1;} void reset (Stack* s){/* Question 10: how to make the stack empty? */ } int main (int argc, char** argv){Stack st1; init (&st1);…arrow_forwardC++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the main code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS int main(int argc, char** argv) { SLLStack* stack = new SLLStack(); int test; int length; string str; char top; bool flag = true; cin >> test; switch (test) { case 0: getline(cin, str); length = str.length(); for(int i = 0; i < length; i++){ if(str[i] == '{' || str[i] == '(' || str[i] == '['){ stack->push(str[i]); } else if (str[i] == '}' || str[i] == ')' || str[i] == ']'){ if(!stack->isEmpty()){ top = stack->top(); if(top == '{' && str[i] == '}' || top == '(' && str[i] == ')' ||…arrow_forwardprogram Linked List: modify the following program to make a node containing data values of int, char, and string. #include <iostream> using namespace std; struct node { int data; struct Node *next; }; struct Node* head = nullptr;//or Null or 0; void insert(int new_data) { struct Node* new_node=(struct Node*) new(struct Node); new_mode->data=new_data; new_mode->next=head; head=new_node; } void display() { struct Node* ptr; ptr=head; while(ptr ! = NULL) { cout<<ptr->data<<""; ptr=ptr->next; } } int main() { insert{2}; display{}; return0; }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
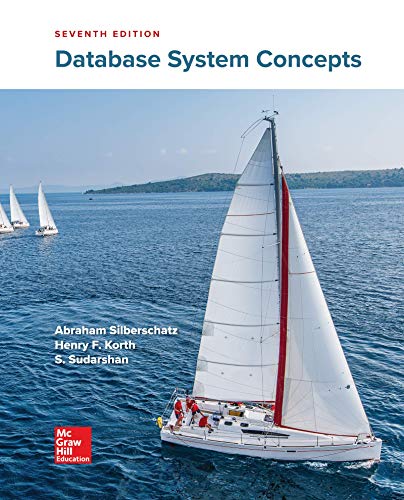
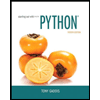
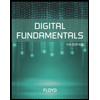
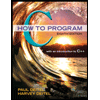
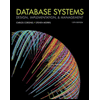
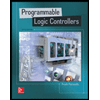