File allocation method: LINKED LIST Total blocks: 32 File allocation start at block: 8 File allocation end at block: 31 Size (kB) of each block: 1 Enter no of files: 3 Enter the name of file #1: data.csV Enter the size (kB) of file #1: 3 Enter the name of file #2: info.doc Enter the size (kB) of file #2: 5 Enter the name of file #3: music.mp3 Enter the size (kB) of file #3: 4
With linked allocation, each file is a linked list of disk blocks; the disk blocks may be scattered anywhere
on the disk. The directory contains a pointer to the first and last blocks of the file. Each block contains a pointer
to the next block. Refer to the illustration below.
Need help to fill in the codes in void main() ---
#include<stdio.h>
#include<stdlib.h>
#define TOTAL_DISK_BLOCKS 32
#define TOTAL_DISK_INODES 8
int blockStatus[TOTAL_DISK_BLOCKS]; // free = 0
int blockList[TOTAL_DISK_BLOCKS - TOTAL_DISK_INODES]; // list of blocks of a file
struct file_table {
char fileName[20];
int fileSize;
struct block *sb;
};
struct file_table fileTable[TOTAL_DISK_BLOCKS - TOTAL_DISK_INODES];
struct block {
int blockNumber;
struct block *next;
};
int AllocateBlocks(int Size) {
---
}
void main()
{
int i = 0, j = 0, numFiles = 0, nextBlock= 0, ret = 1;
char s[20]; struct block *temp;
---
for(i = 0; i < numFiles; i++) {
---
ret = AllocateBlocks(fileTable[i].fileSize);
---
}
---
//Seed the pseudo-random number generator used by rand() with the value seed
srand(1234);
---
}
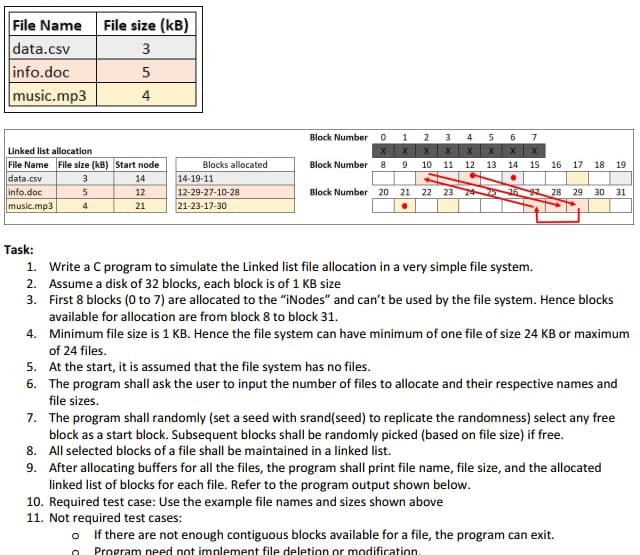
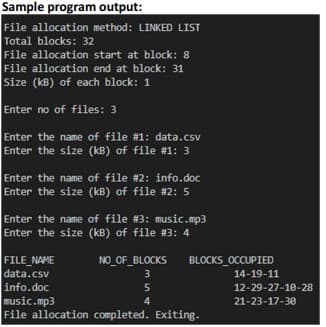

Step by step
Solved in 2 steps

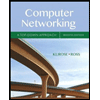
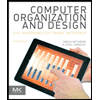
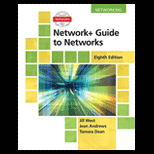
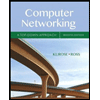
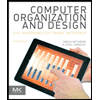
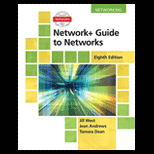
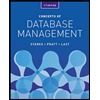
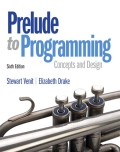
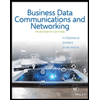